Strategy in VB.NET
Encapsulates an algorithm inside a class
ReturnDefine a family of algorithms, encapsulate each one, and make them interchangeable. Strategy lets the algorithm vary independently from clients that use it. Strategy pattern encapsulate functionality in the form of an object. This allows clients to dynamically change algorithmic strategies.
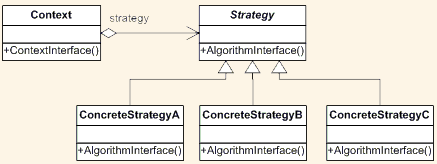
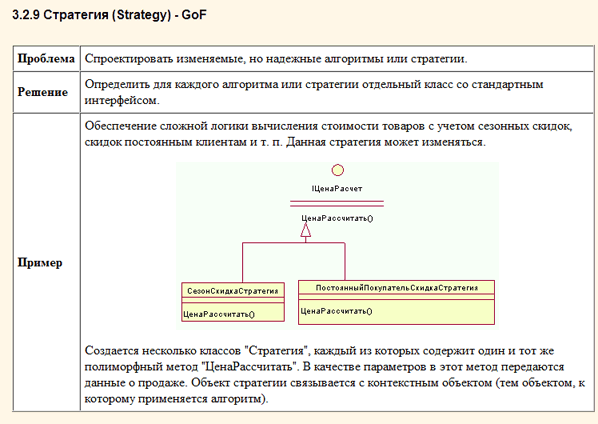
Please download project with this source code from https://github.com/ViacheslavUKR/StandardDisignOopPattern
1: ' Strategy Design Pattern.
2: ' See description in //www.vb-net.com/ProgramTheory/Strategy.htm
3: Class MainApp
4: ' Entry point into console application.
5: Public Shared Sub Main()
6: Dim context As Context
7: ' Three contexts following different strategies
8: context = New Context(New ConcreteStrategyA())
9: context.ContextInterface()
10: context = New Context(New ConcreteStrategyB())
11: context.ContextInterface()
12: context = New Context(New ConcreteStrategyC())
13: context.ContextInterface()
14: ' Wait for user
15: Console.ReadKey()
16: End Sub
17: End Class
18:
19: ' The 'Strategy' abstract class
20: MustInherit Class Strategy
21: Public MustOverride Sub AlgorithmInterface()
22: End Class
23:
24: ' A 'ConcreteStrategy' class
25: Class ConcreteStrategyA
26: Inherits Strategy
27: Public Overrides Sub AlgorithmInterface()
28: Console.WriteLine("Called ConcreteStrategyA.AlgorithmInterface()")
29: End Sub
30: End Class
31:
32: ' A 'ConcreteStrategy' class
33: Class ConcreteStrategyB
34: Inherits Strategy
35: Public Overrides Sub AlgorithmInterface()
36: Console.WriteLine("Called ConcreteStrategyB.AlgorithmInterface()")
37: End Sub
38: End Class
39:
40: ' A 'ConcreteStrategy' class
41: Class ConcreteStrategyC
42: Inherits Strategy
43: Public Overrides Sub AlgorithmInterface()
44: Console.WriteLine("Called ConcreteStrategyC.AlgorithmInterface()")
45: End Sub
46: End Class
47:
48: ' The 'Context' class
49: Class Context
50: Private _strategy As Strategy
51: ' Constructor
52: Public Sub New(strategy As Strategy)
53: Me._strategy = strategy
54: End Sub
55: Public Sub ContextInterface()
56: _strategy.AlgorithmInterface()
57: End Sub
58: End Class

See also:
Creational Patterns
- Abstract Factory in VB.NET - Creates an instance of several families of classes.
- Builder in VB.NET - Separates object construction from its representation.
- Factory Method in VB.NET - Creates an instance of several derived classes.
- Prototype in VB.NET - A fully initialized instance to be copied or cloned.
- Singleton in VB.NET - A class of which only a single instance can exist.
- Adapter in VB.NET - Match interfaces of different classes
- Bridge in VB.NET - Separates an object’s interface from its implementation
- Composite in VB.NET - A tree structure of simple and composite objects
- Decorator in VB.NET - Add responsibilities to objects dynamically
- Facade in VB.NET - A single class that represents an entire subsystem
- Flyweight in VB.NET - A fine-grained instance used for efficient sharing
- Proxy in VB.NET - An object representing another object
- Chain of Resp. in VB.NET - A way of passing a request between a chain of objects
- Command in VB.NET - Encapsulate a command request as an object
- Interpreter in VB.NET - A way to include language elements in a program
- Iterator in VB.NET - Sequentially access the elements of a collection
- Mediator in VB.NET - Defines simplified communication between classes
- Memento in VB.NET - Capture and restore an object's internal state
- Observer in VB.NET - A way of notifying change to a number of classes
- State in VB.NET - Alter an object's behavior when its state changes
- Strategy in VB.NET - Encapsulates an algorithm inside a class
- Template Method in VB.NET - Defer the exact steps of an algorithm to a subclass
- Visitor in VB.NET - Defines a new operation to a class without change
Comments (
)

Link to this page:
//www.vb-net.com/ProgramTheory/Strategy.htm
<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |