Electron learning
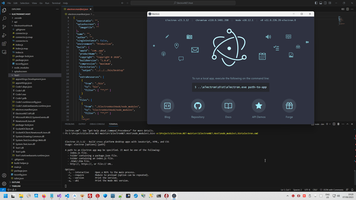
1. Electron for Desktop Apps The Complete Developer's Guide
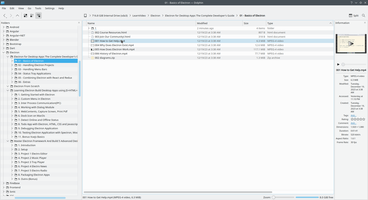
- 01 - Basics of Electron
- 001 How to Get Help
- 002 Course Resources.html
- 002 diagrams.zip
- 003 Join Our Community!.html
- 004 Why Does Electron Exist
- 005 How Does Electron Work
- 006 History of Electron
- 02 - Handling Electron Projects
- 001 App Overview
- 002 Getting Started
- 003 Starting and Stopping Electron
- 004 Loading HTML Docs
- 005 Selecting Videos
- 006 Sample Video - Download Me!.html
- 007 Reading File Details
- 008 Introduction to FFMPEG
- 009 OSX FFMPEG Installation
- 010 Windows FFMPEG Setup.html
- 011 Require Statements in the Browser
- 012 IPC Communication
- 013 Video Duration with FFProbe
- 014 Receiving IPC Events
- 015 Wrapup
- 03 - Handling Menu Bars
- 001 App Overview
- 002 App Boilerplate
- 003 Creating the MainWindow
- 004 Constructing Menu Bars
- 005 Menu Bar Gotchas
- 006 On Menu Click Functionality
- 007 Cross Platform Hotkeys
- 008 Creating Separate Windows
- 009 Another Electron Gotcha
- 010 Adding Polish to Electron
- 011 Restoring Developer Tools
- 012 IPC Between Windows
- 013 Garbage Collection with Electron
- 014 Role Shortcuts
- 015 Your Turn - Clearing Lists
- 016 Solution and Wrapup
- 04 - Status Tray Applications
- 001 App Boilerplate
- 002 What's This React Code
- 003 BrowserWindow vs Tray
- 004 BrowserWindow Config
- 005 Creating Tray Icons
- 006 Toggling BrowserWindow Visibility
- 007 Detecting Visibility
- 008 The Positioning Bounds System
- 009 Setting Position with Bounds
- 010 Positioning of Windows
- 011 Object Oriented Programming with Electron
- 012 Basics of ES6 Classes
- 013 Subclassing
- 014 The TimerTray Subclass
- 015 Finishing TimerTray Refactor
- 016 Setting Tooltips
- 017 More on Garbage Collection
- 018 Building Context Menus
- 019 Controlling Window Focus
- 020 Hiding Dock Icons
- 021 MainWindow Class
- 022 IPC Between React and Electron
- 023 Displaying Text on the Tray
- 024 Background Throttling
- 05 - Combining Electron with React and Redux
- 001 App Overview
- 002 App Challenges
- 003 BrowserWindow Creation
- 004 Starting up Electron with Webpack
- 005 Overview of React and Redux
- 006 Receiving a List of Videos
- 007 Video Metadata
- 008 Handling Async Bulk Operations with Promises
- 009 Fetching Metadata in Promises
- 010 Refactoring for Multiple Videos
- 011 Wrapup on Metadata
- 012 Output Paths
- 013 Batch Video Conversion
- 014 Handling Conversion Completion
- 015 Detecting Conversion Progress
- 016 Opening Folders with Shell
- 017 Wrapup
- 06 - Extras
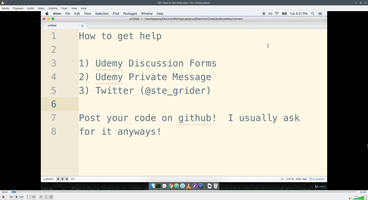
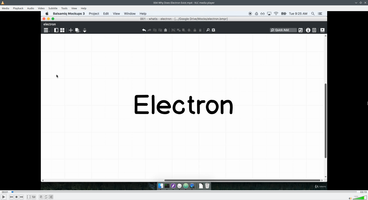
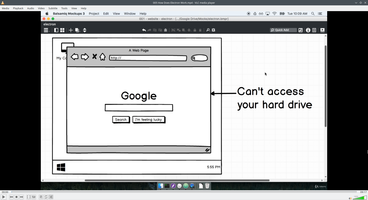
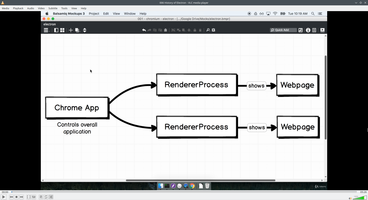
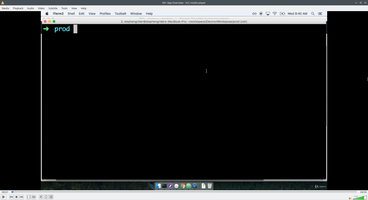
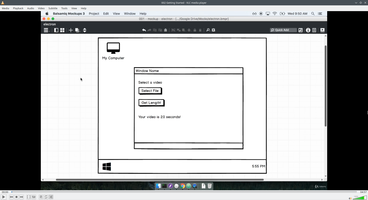
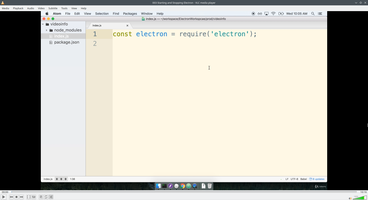
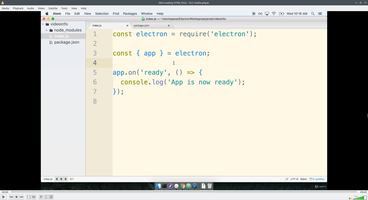
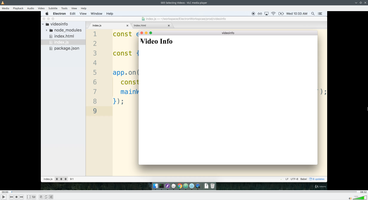
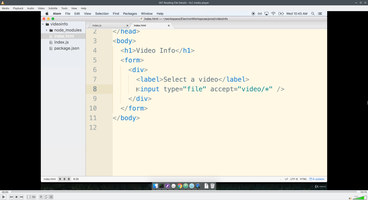
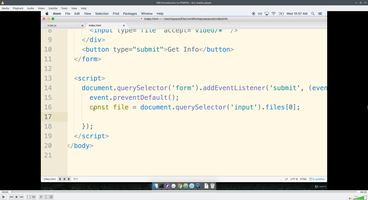
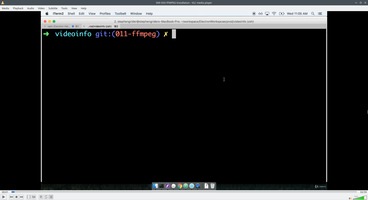
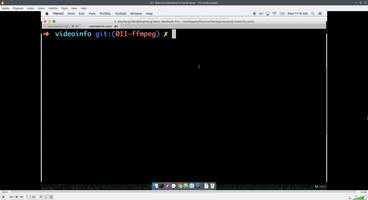
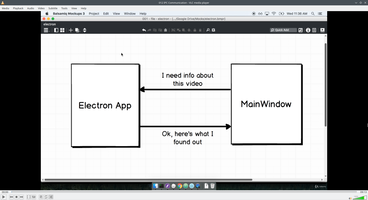
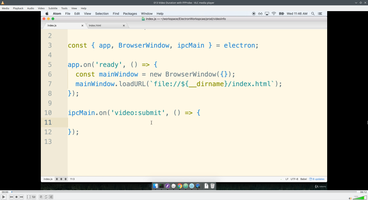
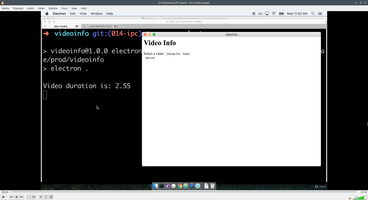
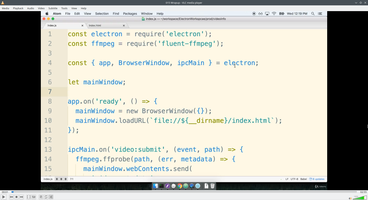
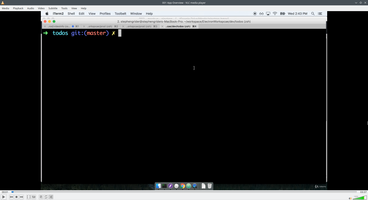
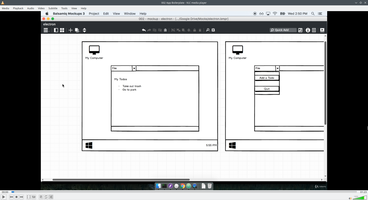
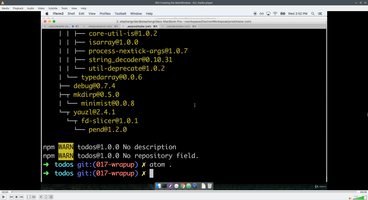
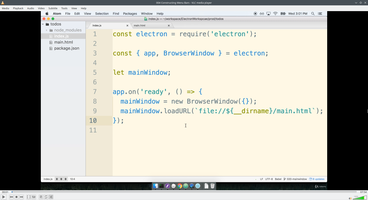
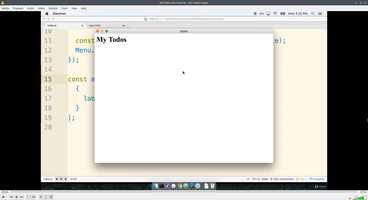
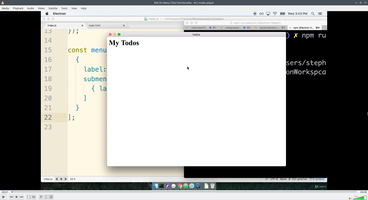
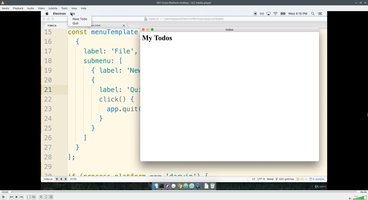
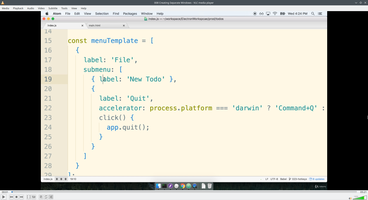
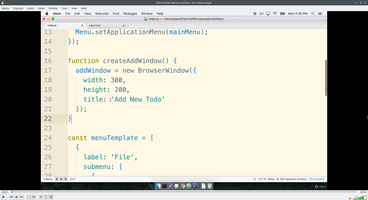
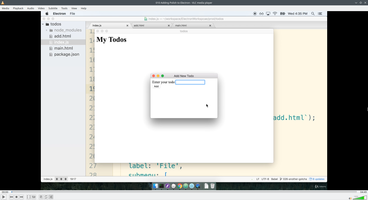
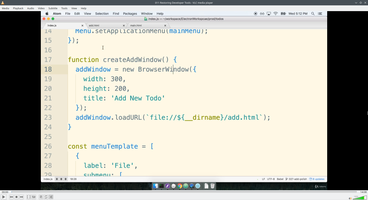
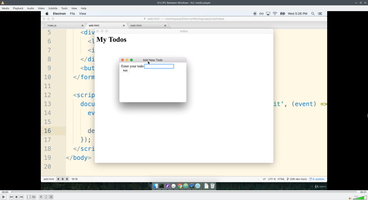
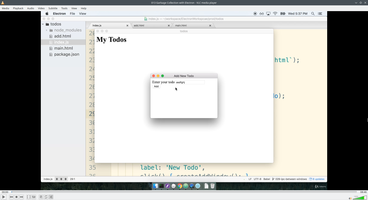
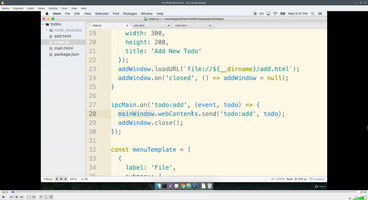
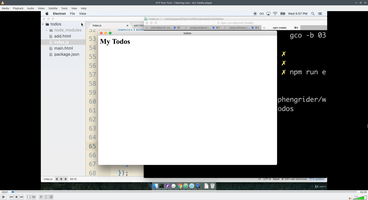
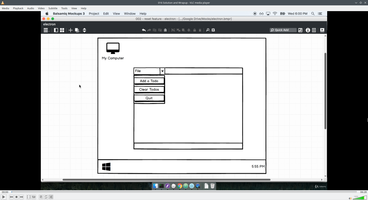
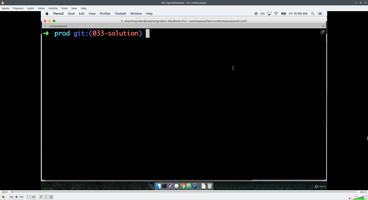
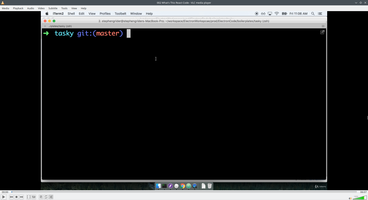
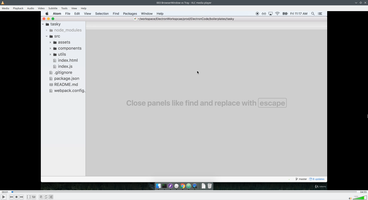
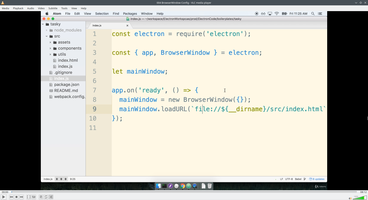
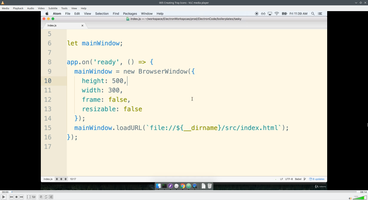
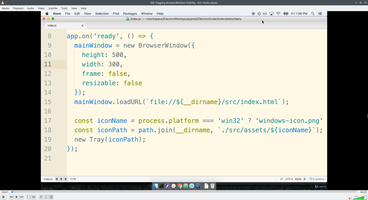
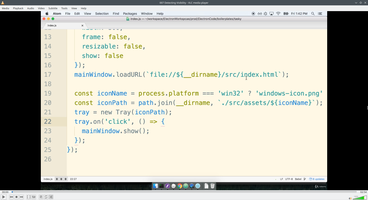
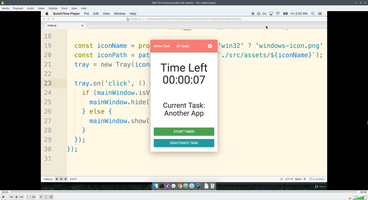
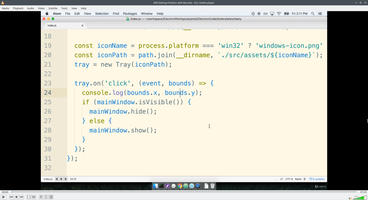
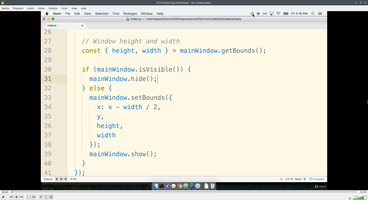
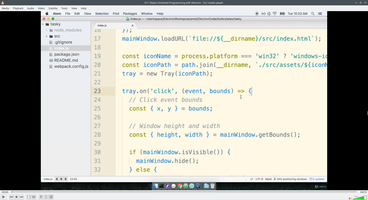
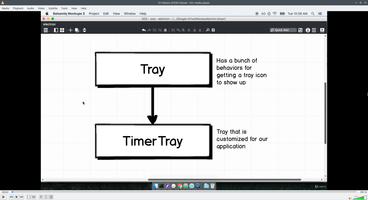
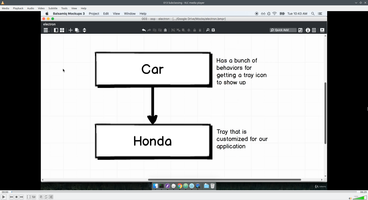
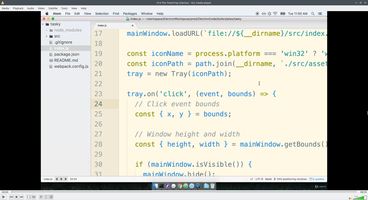
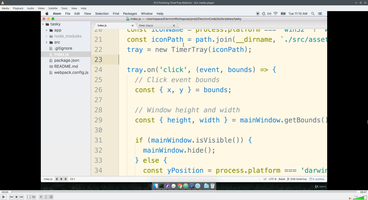
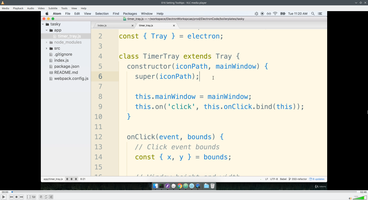
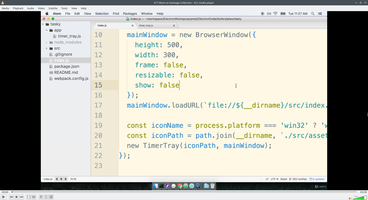
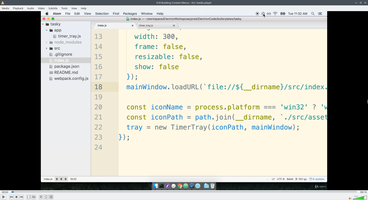
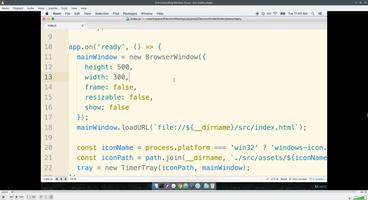
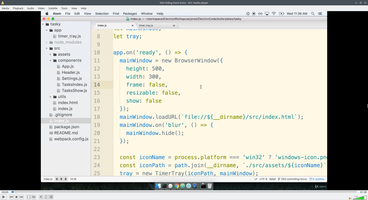
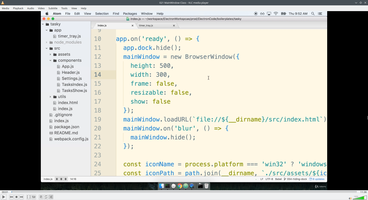
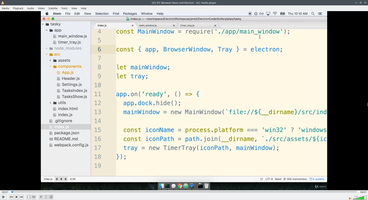
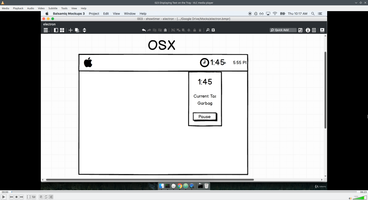
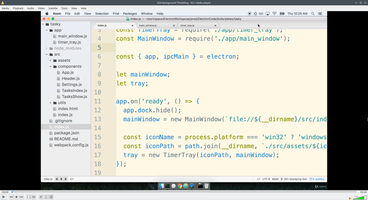
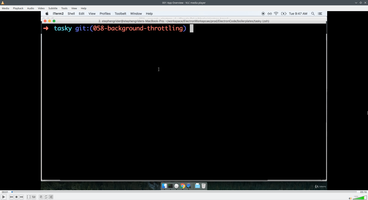
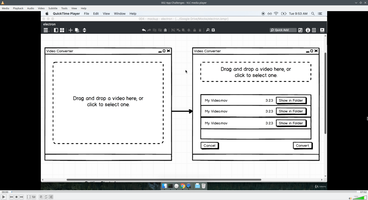
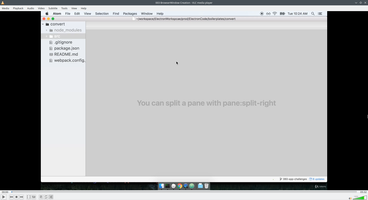
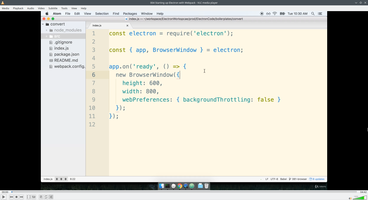
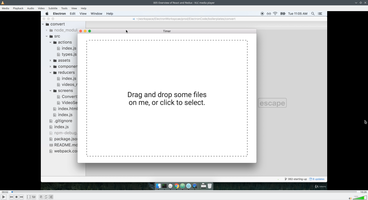
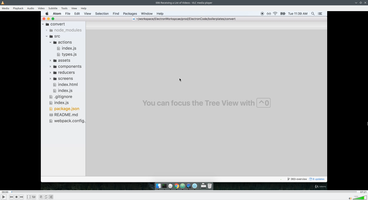
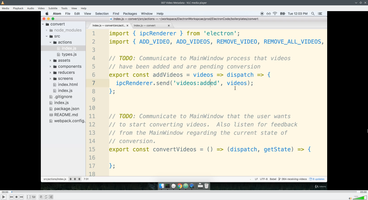
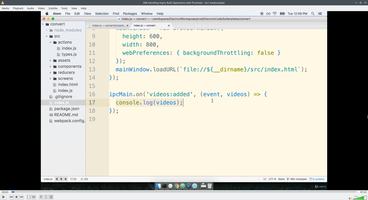
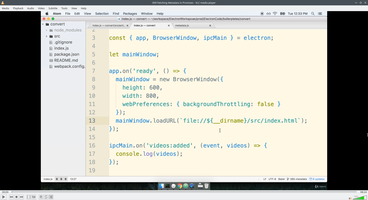
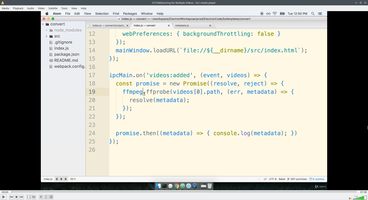
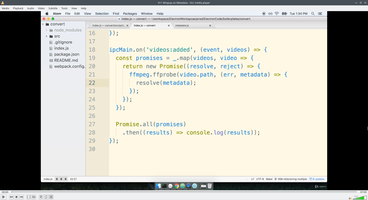
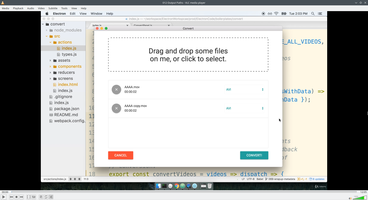
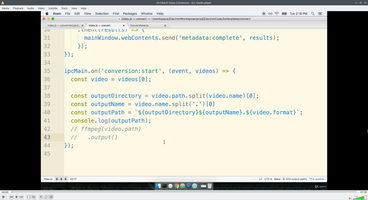
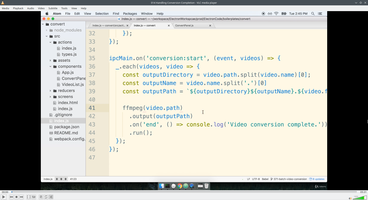
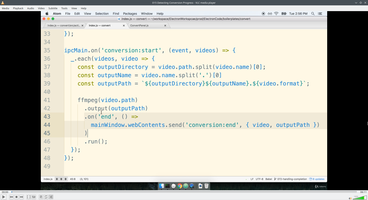
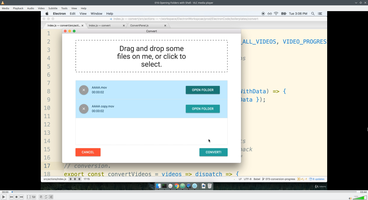
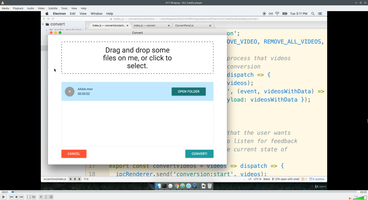
2. Electron From Scratch
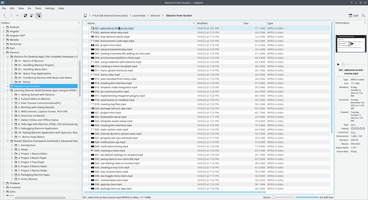
- 001. welcome-to-the-course
- 002. electron-what-why
- 003. how-electron-works
- 004. environment-code-repo
- 005. project-intro
- 006. setup-browserwindow
- 007. loading-a-window-file-adding-an-icon
- 008. environment-platform-check
- 009. using-nodemon-with-electron
- 010. creating-a-menu-template
- 011. menu-global-shortcuts
- 012. menu-roles
- 013. open-window-from-menu
- 014. creating-the-interface
- 015. renderer-node-integration
- 016. ipc-communication
- 017. implementing-imagemin-plugins
- 018. send-events-to-renderer
- 019. creating-log-files
- 020. package-test-our-app
- 021. project-intro
- 022. boilerplate-setup
- 023. template-assets-setup
- 024. creating-the-interface
- 025. static-system-stats
- 026. interval-dynamic-system-stats
- 027. progress-bar-cpu-overload
- 028. notifications-api
- 029. notification-timing
- 030. creating-a-store
- 031. set-default-settings-to-renderer
- 032. saving-data-to-our-store-file
- 033. use-settings-data-in-monitor
- 034. creating-a-tray-icon
- 035. tray-context-menu
- 036. nav-toggle-menu-item
- 037. mainwindow-class
- 038. apptray-class
- 039. package-test-our-app
- 040. project-intro
- 041. react-electron-boilerplate-setup
- 042. log-state-table
- 043. logitem-component
- 044. formatting-dates-with-moment
- 045. addlogitem-component
- 046. adding-a-log-ui-only
- 047. displaying-alerts
- 048. deleting-a-log-ui-only
- 049. setup-mongodb-atlas
- 050. connect-to-database-with-mongoose
- 051. create-log-model
- 052. get-logs-from-database
- 053. add-log-to-database
- 054. delete-logs-from-database
- 055. clear-logs-menu-item
- 056. package-test-our-app
- 057. custom-icon
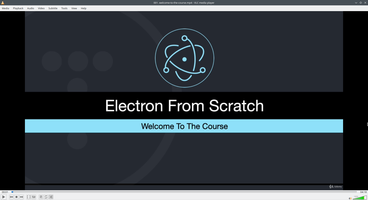
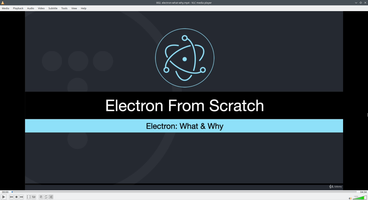
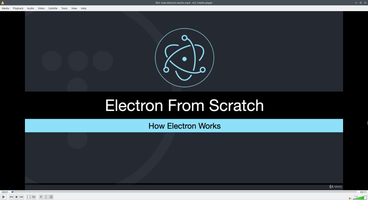
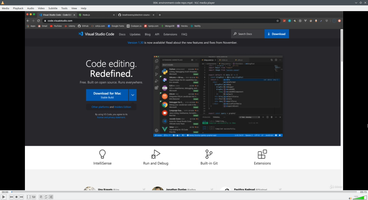
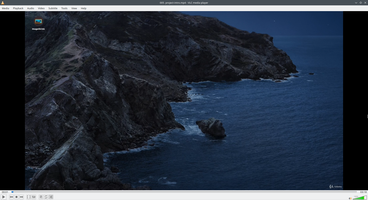
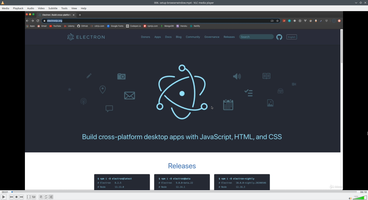
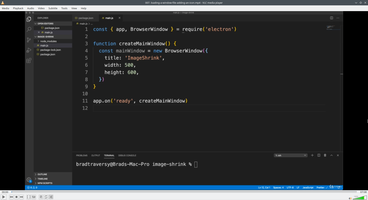
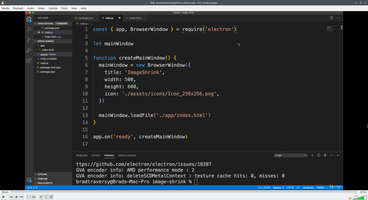
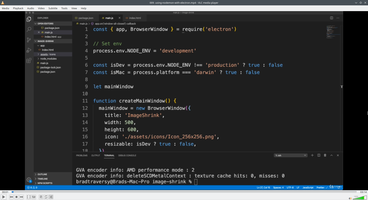
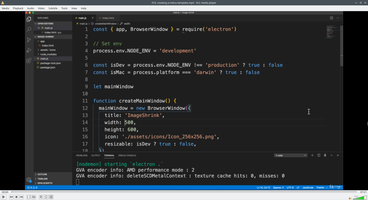
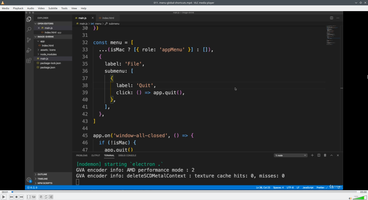
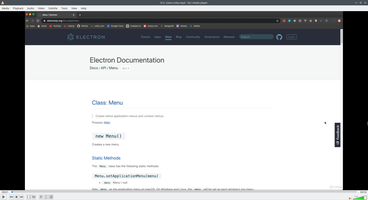
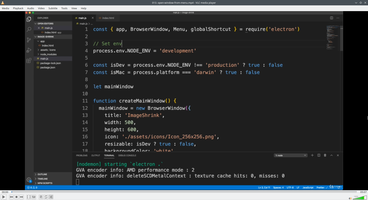
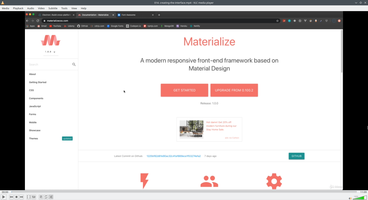
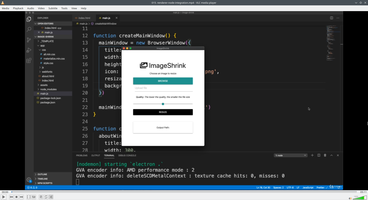
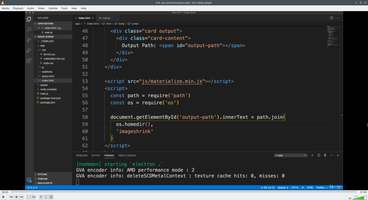
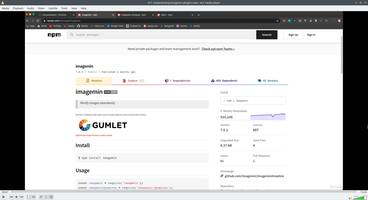
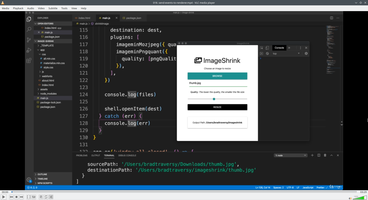
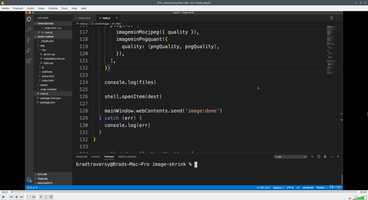
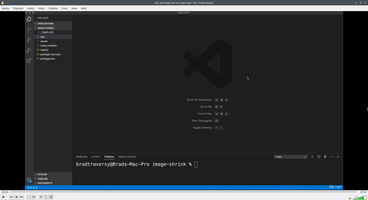
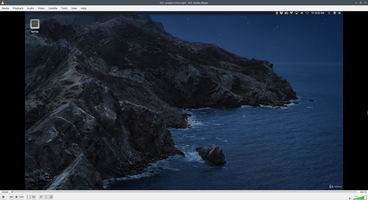
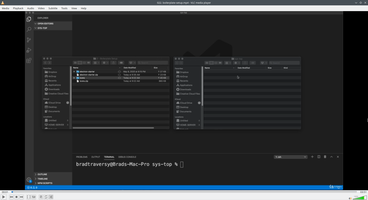
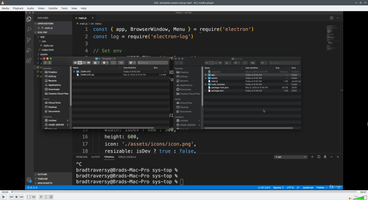
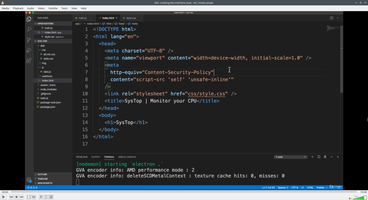
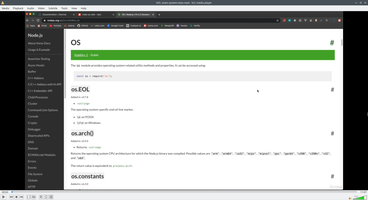
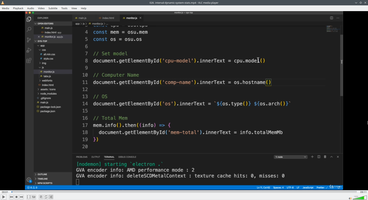
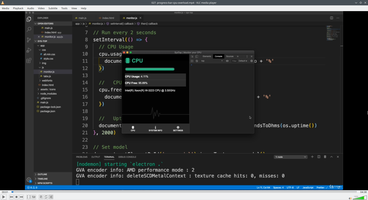
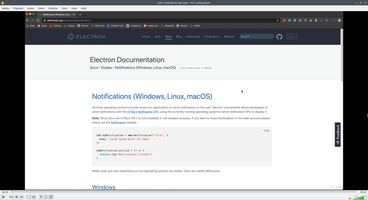
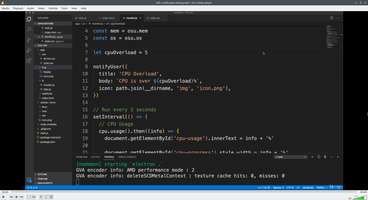
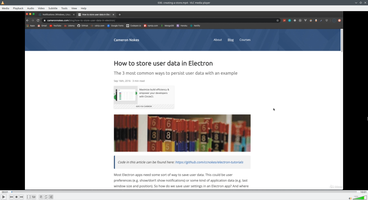
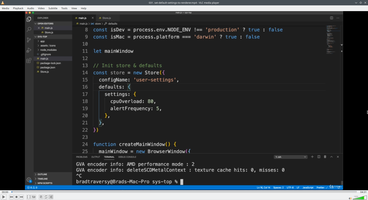
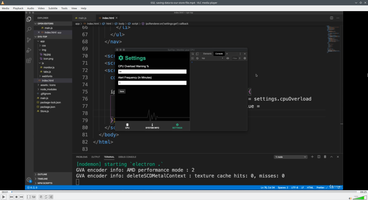
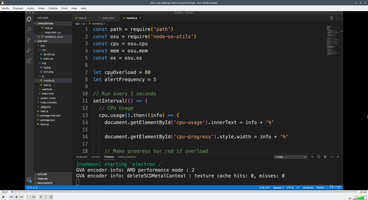
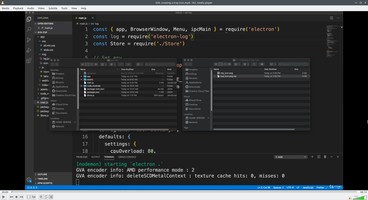
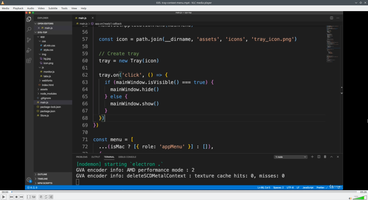
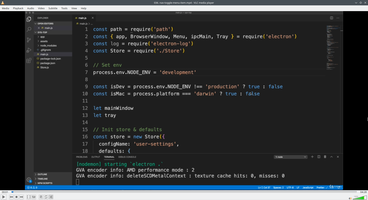
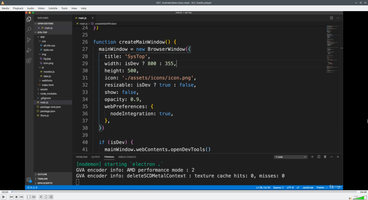
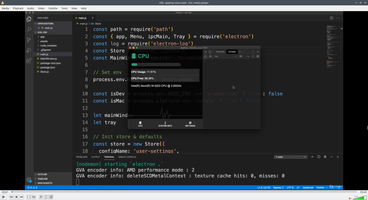
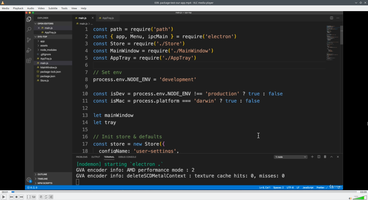
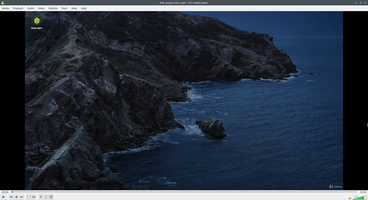
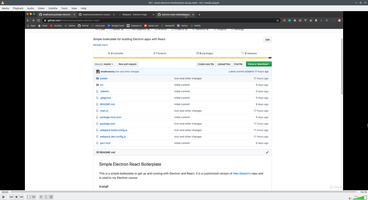
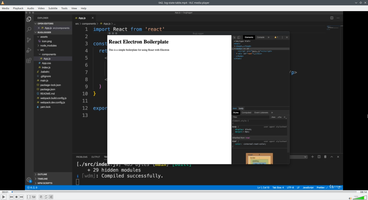
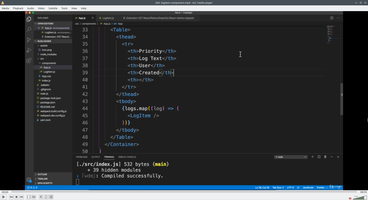
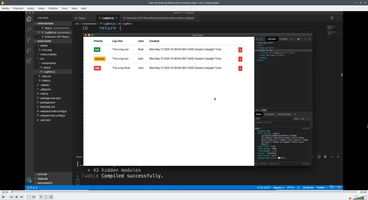
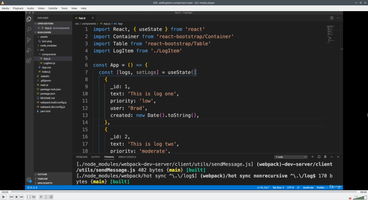
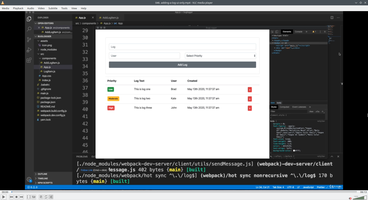
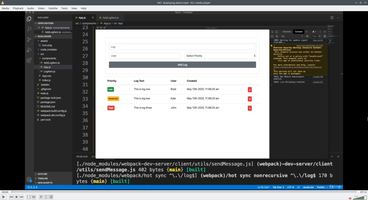
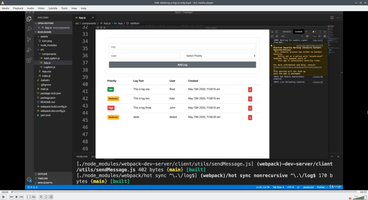
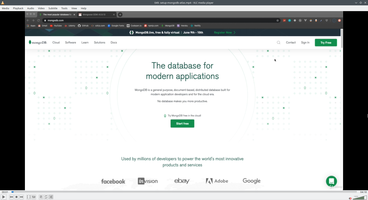
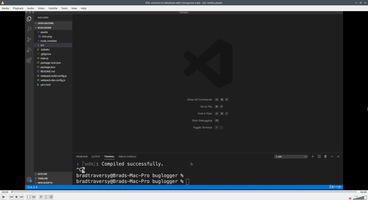
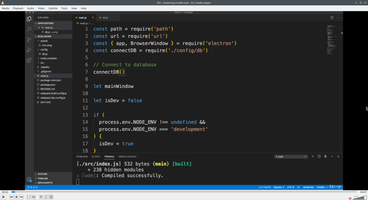
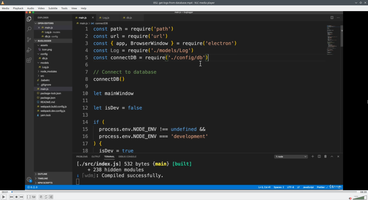
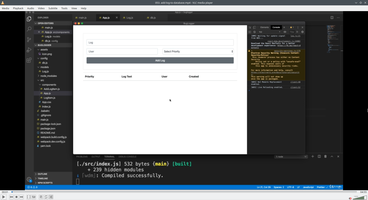
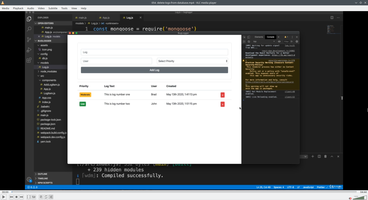
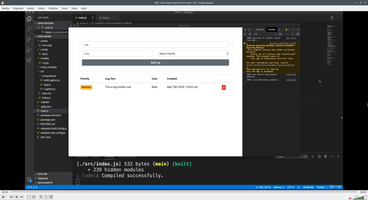
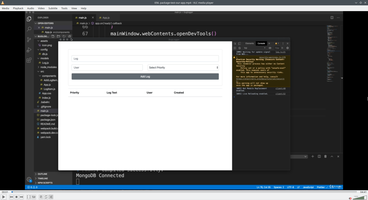
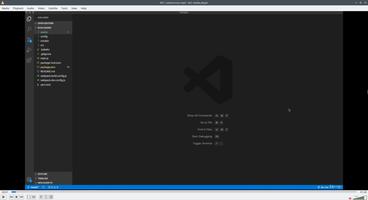
3. Learning Electron Build Desktop Apps using JS+HTML+CSS
- 1. Getting Started with Electron
- 1. Hello world in Electron
- 2. Loading Html file in Electron MainBrowserWindow
- 3. Generate file path to display web page
- 4. Load Content on BrowserWindow after rendering web page
- 5. BrowserWindow Properties
- 6. BrowserWindow title and alwaysOnTop property
- 7. Create Frameless window to Remove title and toolbar
- 8. Create Transparent Window
- 2. Custom Menu in Electron
- 1. Create Custom Menu
- 2. Define Keyboard Shortcuts and MenuItem Roles
- 3. Add Styling to Fix background text color warning
- 4. Types of MeuItem
- 5. Build MacOS Application Menu
- 6. Reload app on file changes using electron-reload
- 7. Create a View Menu Item
- 8. Create a window Menu Item
- 9. Create a Custom Menu
- 3. Inter Process Communication(IPC)
- 1. Communicate synchronously between main and renderer process
- 2. Communicate Asynchronously between main and renderer process
- 4. Working with Dialog Module
- 1. Open File Dialog to select files and directories
- 2. Add additional options for FileDialog
- 3. Open the File in NodeJs using fs.open()
- 4. Reading Writing and deleting the File
- 5. Watch for changes on file
- 6. Creating, Reading and Deleting Directory
- 7. Choose a File from PC using File Save Dialog
- 8. MessageBox Dialog
- 9. Adding custom Icons in showMessageBox Dialog
- 5. WebContents, Capture Screen, Print Pdf
- 1. Understanding webContents API
- 2. Capture the snapshot of the BrowserWindow and save as a PNG File
- 3. Prints window's web page as PDF
- 6. Dock Icon on MacOs
- 1. Update Image associated with Dock Icon
- 2. Make Dock Icon Bounce
- 3. Change Dock Icon and set badge
- 4. Make System Alert sound using shell module
- 5. Open Files in Finder and External Browser
- 7. Detect Online and Offline Status
- 1. Detect online and offline inside rendererjs
- 3. Detect online or offline status using electron-online
- 8. Todo App with Electron, HTML, CSS and Javascript
- 1. Getting setup with Main Window
- 2. Adding Bootstrap in Electron Application
- 3. File Structure for Todo App
- 4. Setup DataStore
- 5. Iterate all Todos and display them in MainBrowserWindow
- 6. Create new Menu Item and open a new Browser Window
- 7. Save new Record in DataStore
- 8. Delete Record from DataStore
- 9. Debugging Electron Application
- 1. Create Menu Item to debug Electron Application
- 2. Debug Electron Main process in Vs Code
- 3. Debug Electron app using Devtron
- 10. Testing Electron Application with Spectron, Mocha, and Chai
- 1. Testing setup with Spectron, Mocha, and Chai
- 2. Testing Spectron BrowserWindow Api
- 3. Testing BrowserWindow size
- 4. Testing inter process communication with main process and renderer process
- 11. Bonus Vue.js Basics
- 1. Getting started with Vue
- 2. Create template as the markup for the Vue Instance
- 3. Bind properties of vue instance using data
- 4. Create method to interacts with Vue app
- 5. Use computed properties to cache calculations
- 6. Execute Javascript expressions in Template Bindings
- 7. Conditional rendering using v-show directive
- 8. Conditional rendering using v-if, v-else, and v-else-if directive
- 9. Mapping an Array to Elements with v-for
- 10. Array Change detection
- 11. Using Vue.set and splice method to replace an Item from Array
- 12. Using v-for to iterate properties of an Object
- 13. Computed properties in vue
- 15. Watch a property of data object through watcher
- 16. Watching properties of Objects in the Data object
- 17. Handling click event using v-onclick
- 18. Passing Arguments to Event Handler
- 19. Event Modifiers and Key Modifiers
- 20. Form Input Bindings
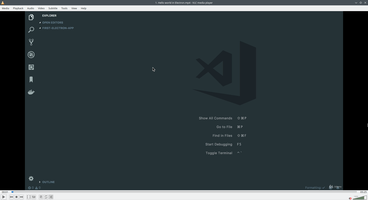
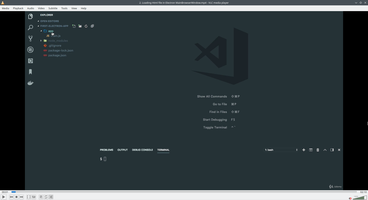
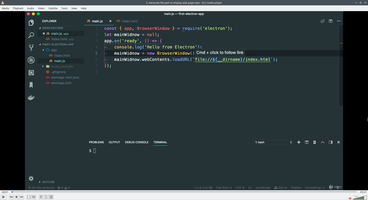
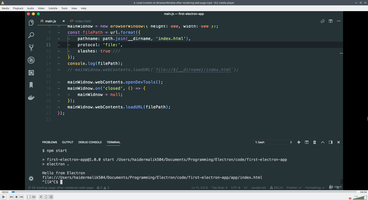
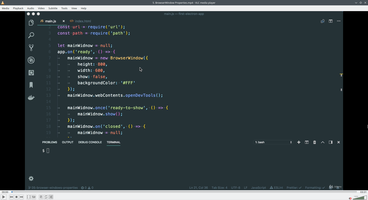
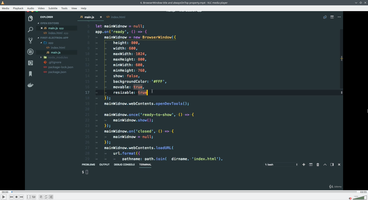
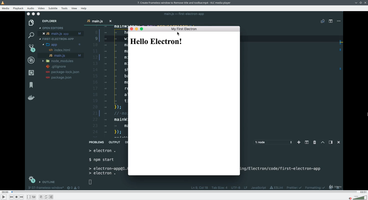
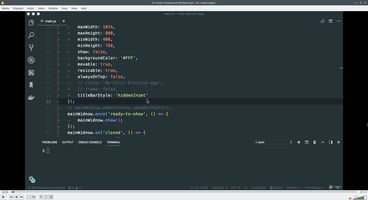
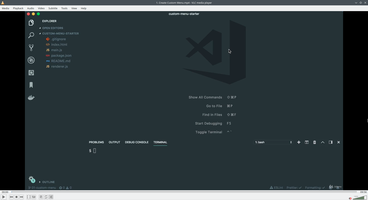
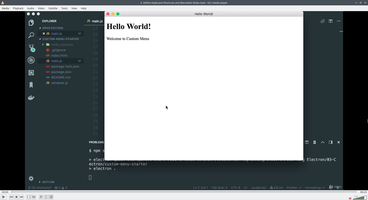
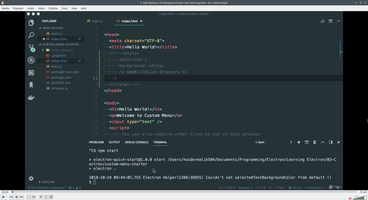
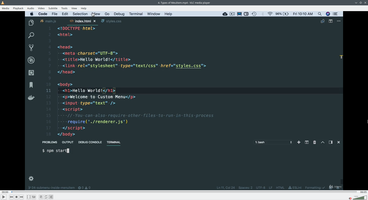
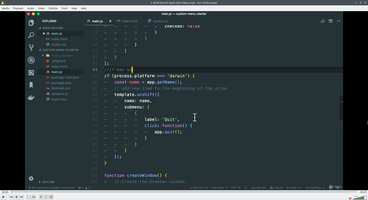
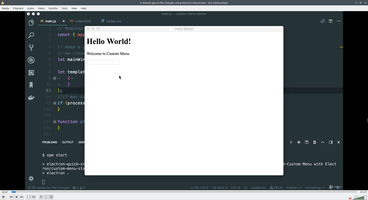
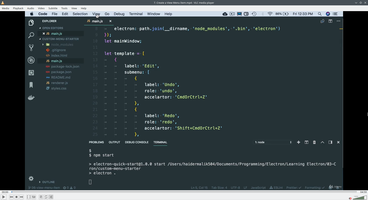
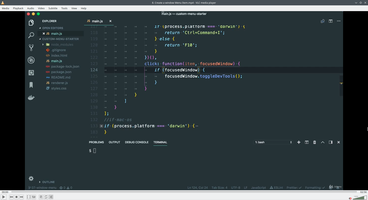
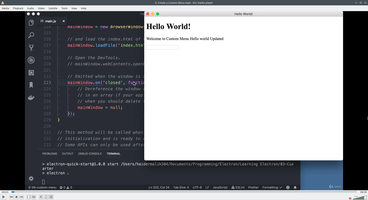
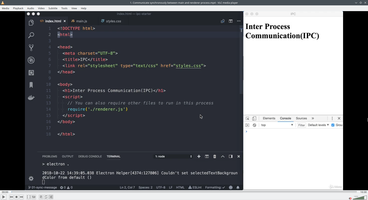
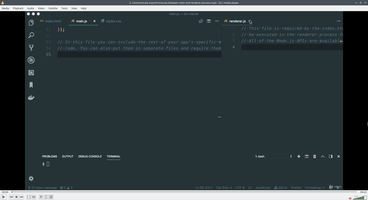
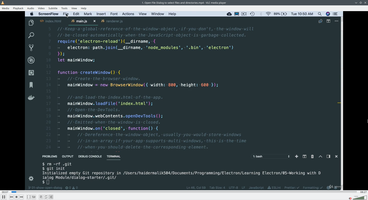
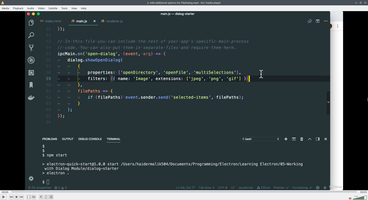
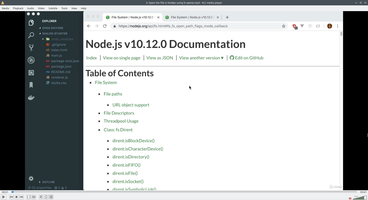
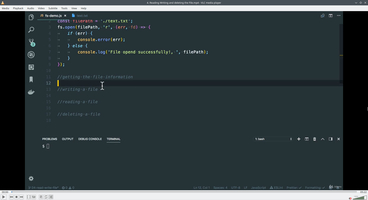
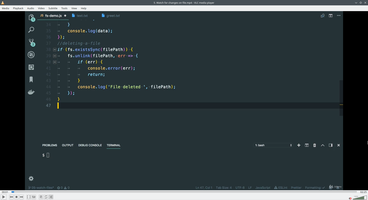
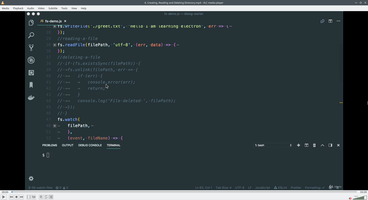
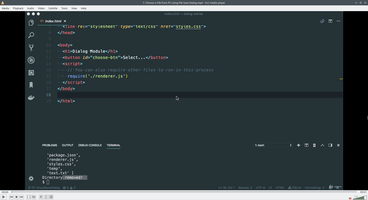
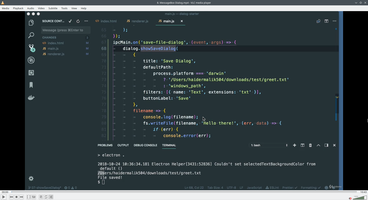
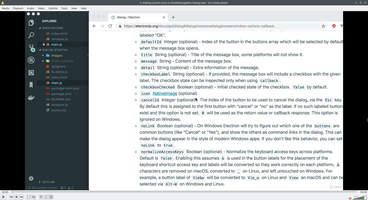
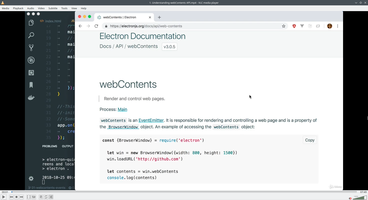
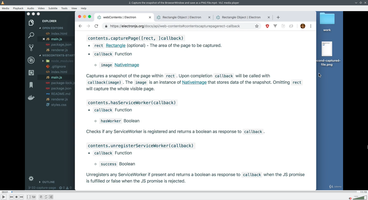
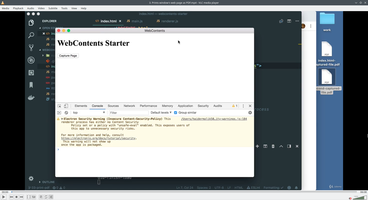
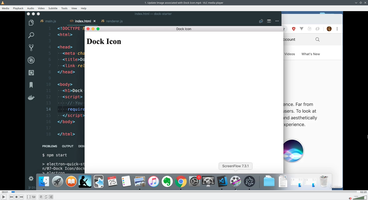
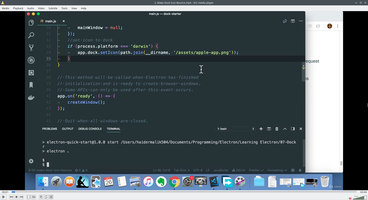
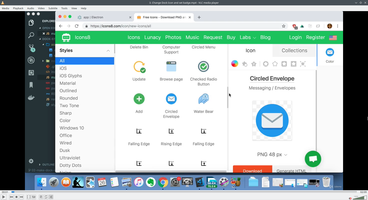
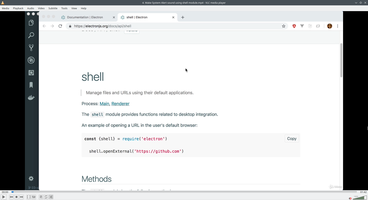
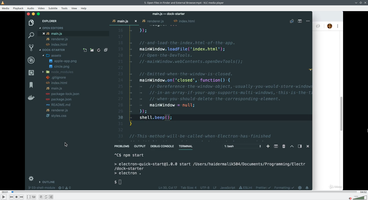
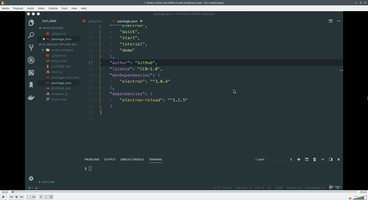
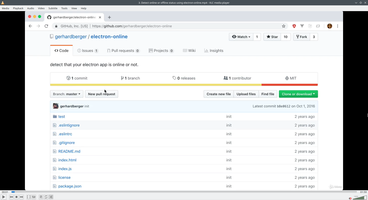
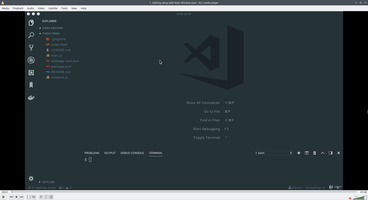
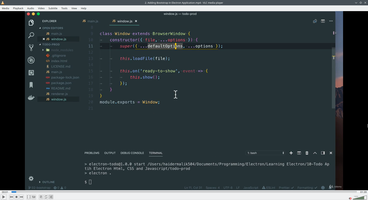
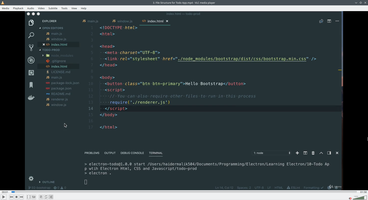
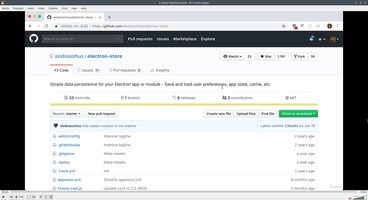
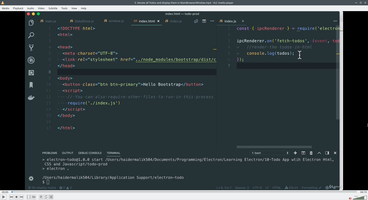
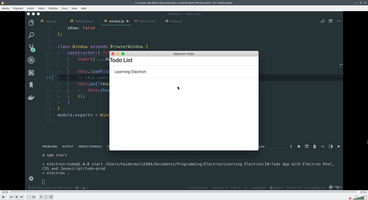
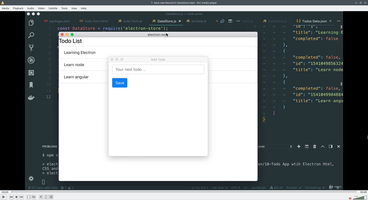
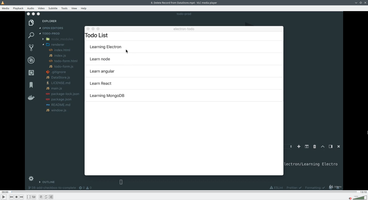
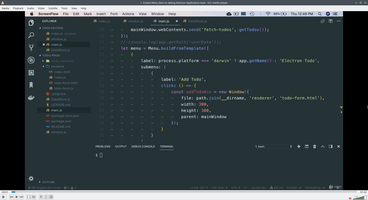
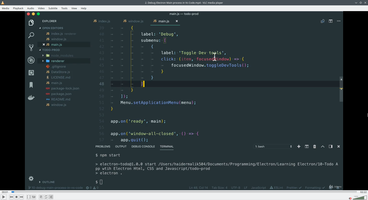
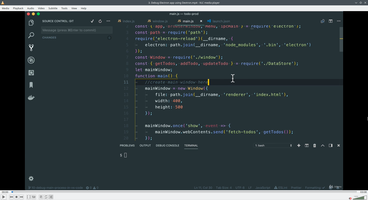
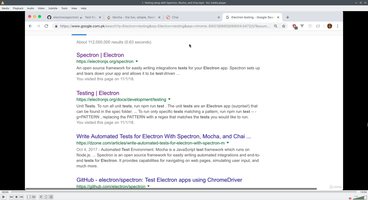
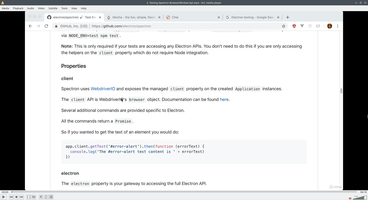

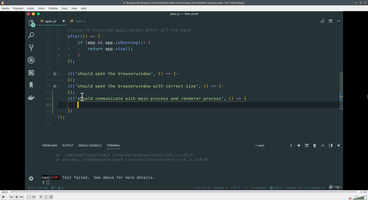
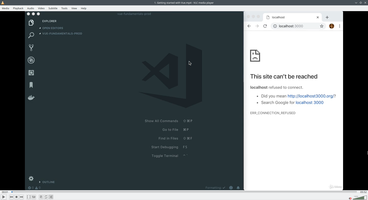
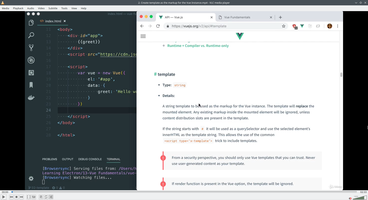
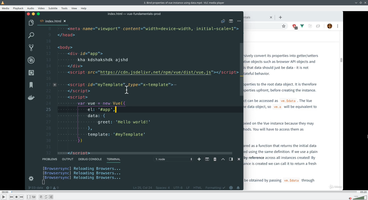
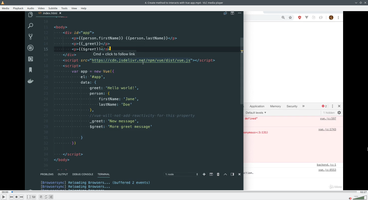
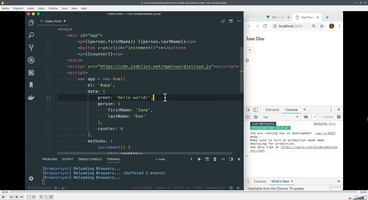
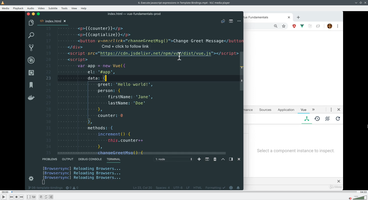
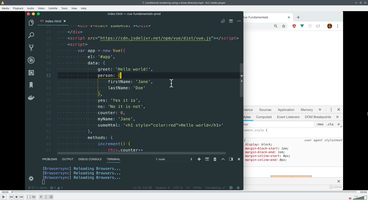
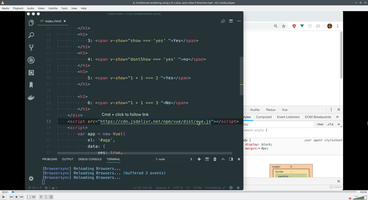
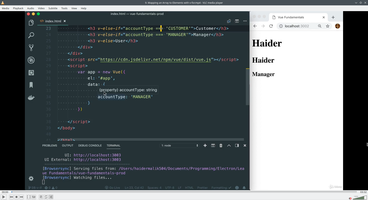
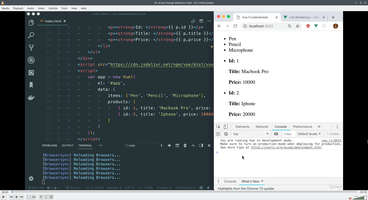
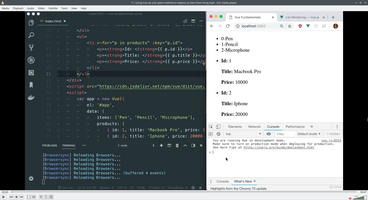
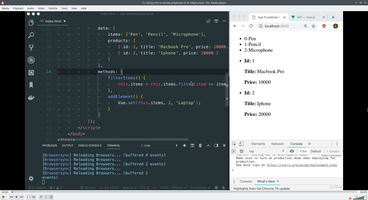
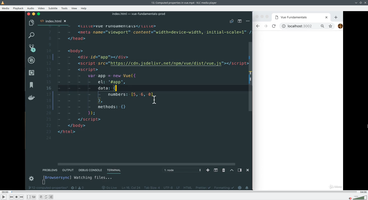
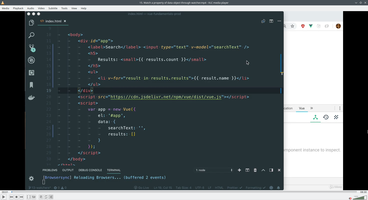
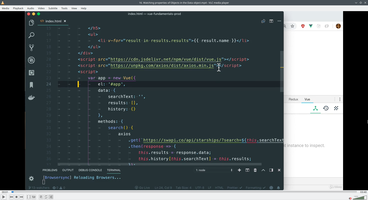
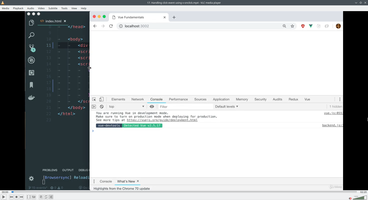
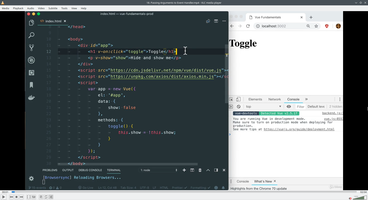
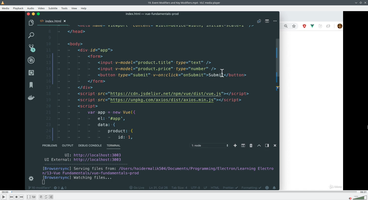
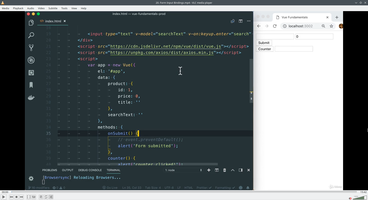
4. Master Electron Framework And Build 5 Advanced Desktop Apps
- 1. Introduction
- 2. Setup
- 1. What is Node JS
- 2. Installing Node JS on macOS and Linux
- 3. Installing Node JS on Windows
- 4. Summary
- 3. Project 1 Electro Editor
- 1. Demo of Electro Editor
- 2. Installing Electron v5 With npm
- 3. Main.js And Running Electron
- 4. Adding our Renderer Process
- 5. Introduction to Photon UI Kit
- 6. Building Renderer With Photon Kit
- 7. Building Renderer With Photon Kit (Continuation)
- 8. Increasing and Decreasing The Font Size
- 9. Getting The Text Logged
- 10. All About Inter Process Communication (IPC)
- 11. Sending Data to The Main Process
- 12. Node Integration - Watch Out!!!
- 13. Writing Content to File
- 14. Adding The Save Dialog
- 15. Showing The Save Dialog First Time Only
- 16. Sending Data Back to The Renderer Process
- 17. Adding a Save Status Alert to Renderer
- 18. Native Menu in Electron - The Template
- 19. Making Menu Compatible with macOS
- 20. Finishing Up Our Menu
- 21. Adding Menu Keyboard Shortcut
- 22. Wrap Up With Electro Editor Desktop App
- 4. Project 2 Music Player
- 1. Demo of Electron Music Player
- 2. Init npm And Install Electron v5
- 3. Setup Main and Renderer Process
- 4. Building The Renderer
- 5. Button Click to Choose Songs
- 6. Get Songs and Intro to mm Package
- 7. Using The mm Package to Get Data From Songs
- 8. Displaying All Songs in Our Renderer Process
- 9. Playing The Song
- 10. Showing Current Playing Song Title
- 11. Making Our Play-Pause Button Work
- 12. The Previous And Next Song Buttons
- 13. Showing The Current Song Time and Total Duration
- 14. Let's Clear The Playlist
- 15. Wrap Up With Music Player
- 16. NEW!!! Adding Volume Control
- 17. NEW!!! Style Your App Challenge
- 5. Project 3 Tray Player
- 1. Introduction To Tray Applications
- 2. Demo of Tray Player
- 3. Tray in The Docs and Setup
- 4. Adding The Tray Icon
- 5. Fix Disappearing Icon and Remove App Frame
- 6. Hide App on Blur and Remove From Dock
- 7. Icon And Application Bounds
- 8. Adding a Context Menu and Tooltip
- 9. We're Almost There
- 10. DESKTOP NOTIFICATIONS!!! - Adding notification to our App
- 6. Project 4 Electro News
- 1. Demo of Electro News App
- 2. npm Init and Install Electron
- 3. Starting The Electron Process
- 4. Building The Renderer Process
- 5. Intro and Installing NewsAPI
- 6. Getting Top Headlines From NewsAPI
- 7. Showing All News In Renderer
- 8. Reading Articles
- 9. Let's Add News Categories
- 10. Some Highlight to The Active Category
- 11. Let's Not Forget The News Search
- 12. Wrap Up With Electro News App
- 7. Project 5 Electro Radio
- 1. Demo of Electro Radio
- 2. Let's Setup
- 3. Main and Renderer Process
- 4. Finish Renderer and Intro to FM API
- 5. Getting All Radio Stations
- 6. Showing all Stations in the Renderer
- 7. Streaming The Radio Stations
- 8. Some Highlight to Current Station
- 9. Wrap Up With Electro Radio App
- 8. Packaging Electron Apps
- 1. Let's Package Our Music Player
- 2. Requirements for Windows Operating System
- 3. Requirements for macOS and Linux
- 4. Packaging Electron Apps for Windows Operating System
- 5. Packaging Electron Apps for macOS
- 6. Packaging Electron Apps for Linux
- 7. Shortcuts to Make Everything Simpler
- 9. Outro (Bonus)
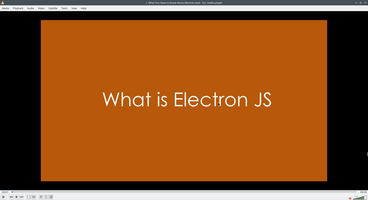
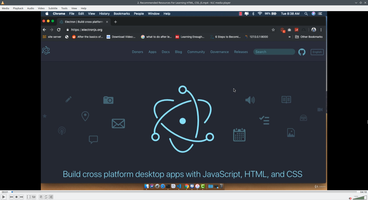
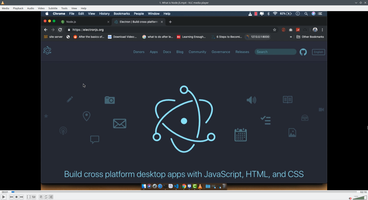
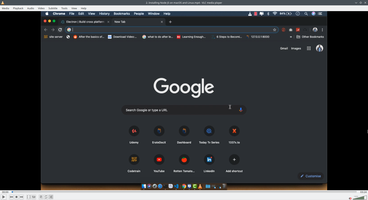
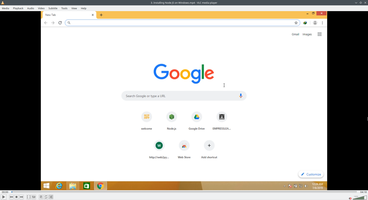
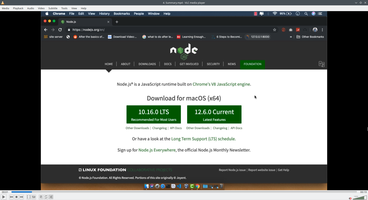
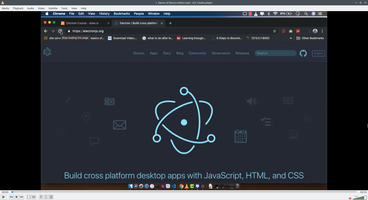
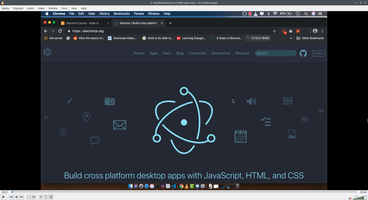
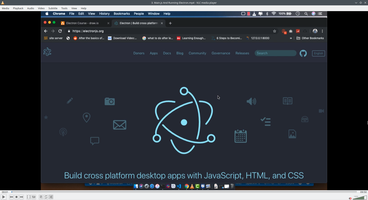
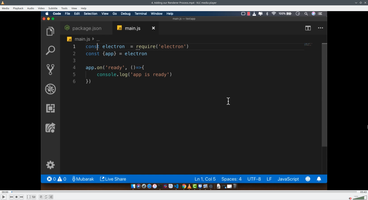
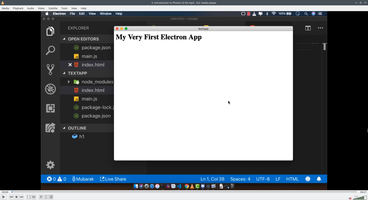
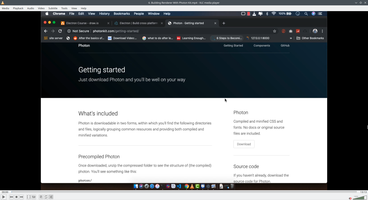
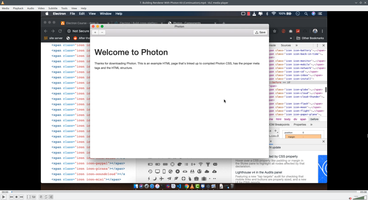
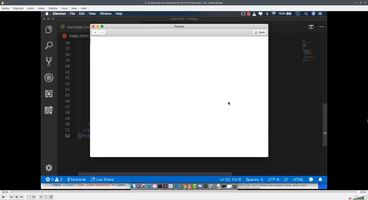
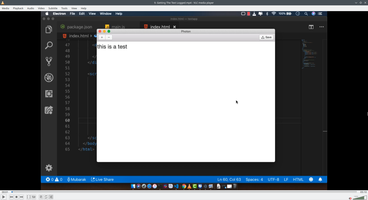
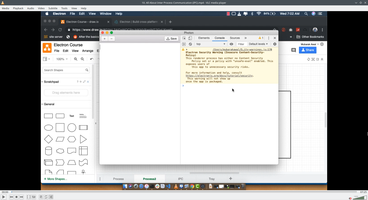
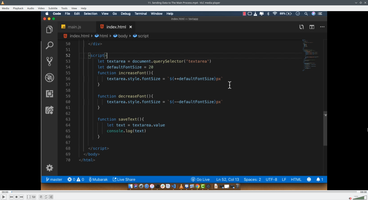
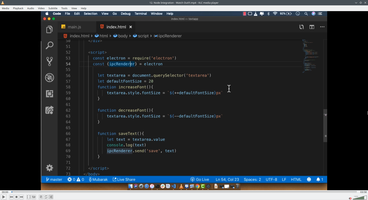
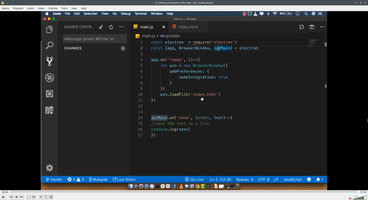
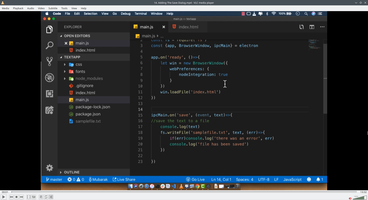
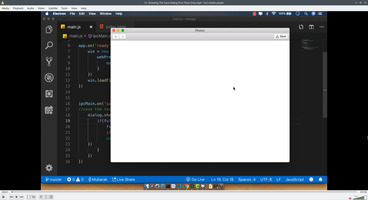
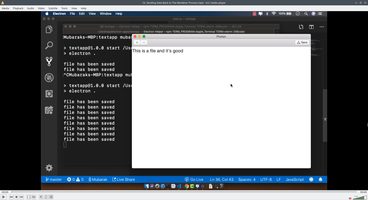
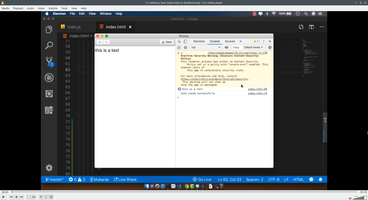
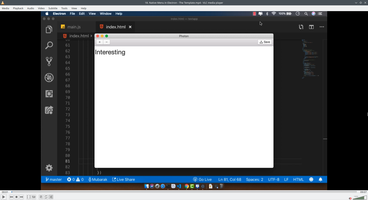
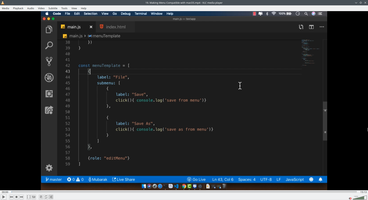
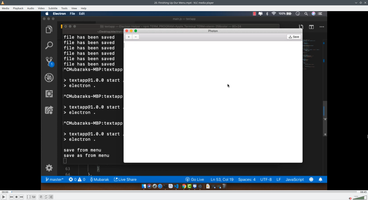
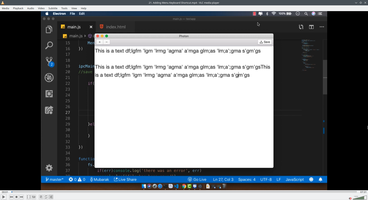
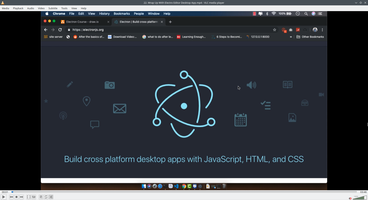
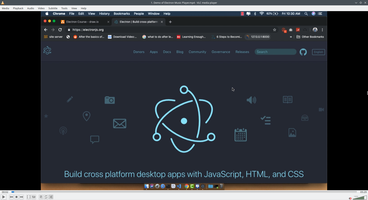
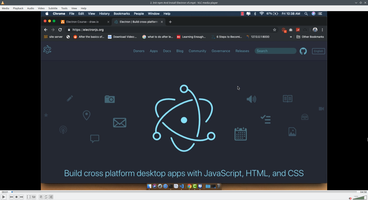
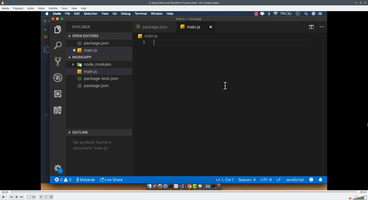
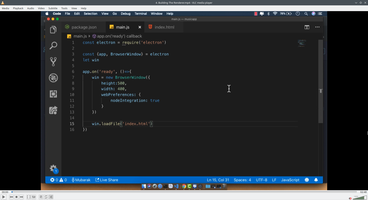
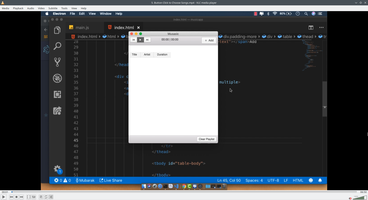
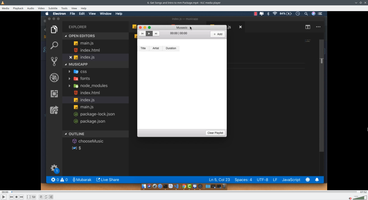
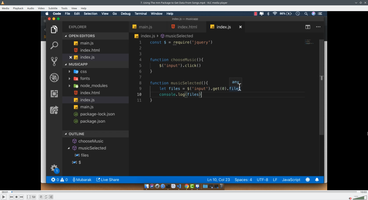
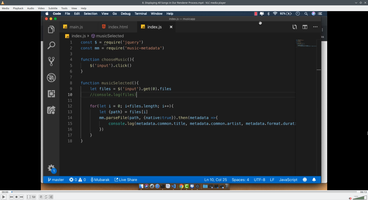
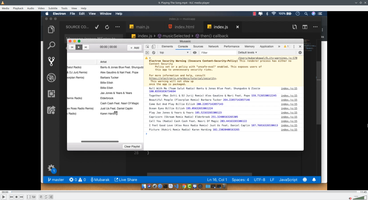
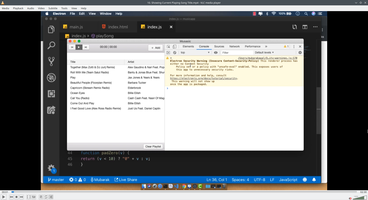
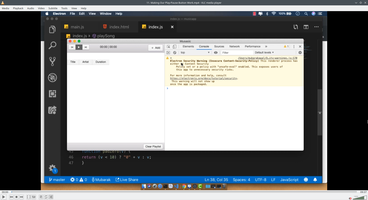
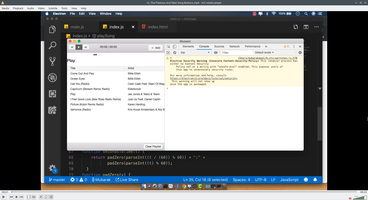
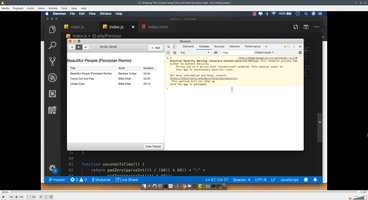
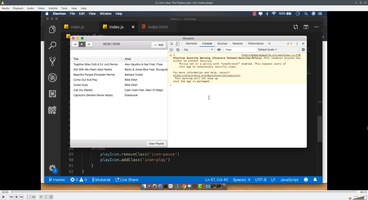
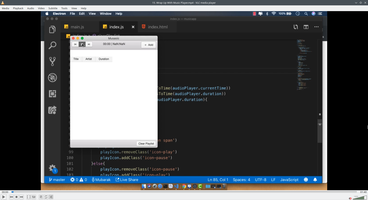
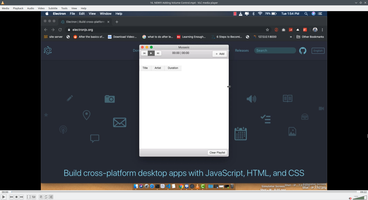
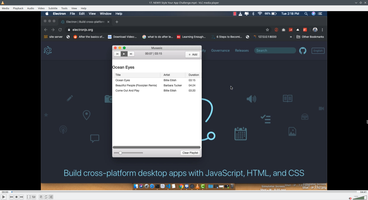
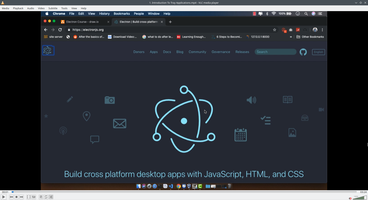
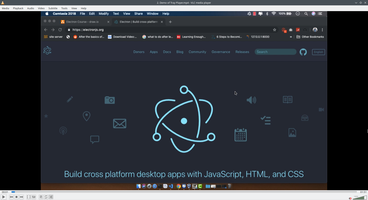
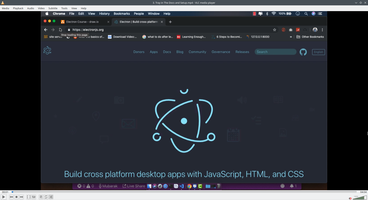
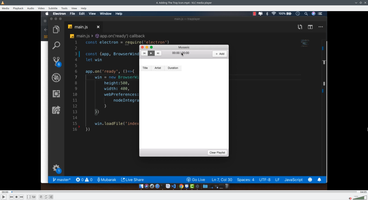
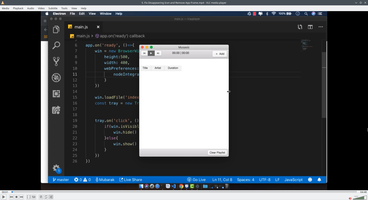
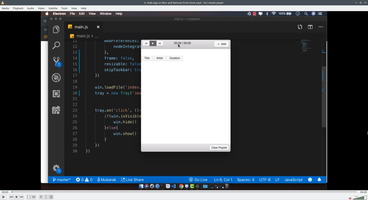
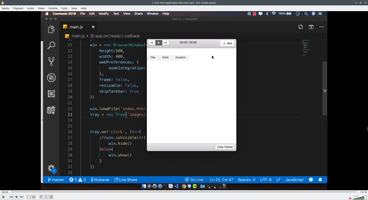
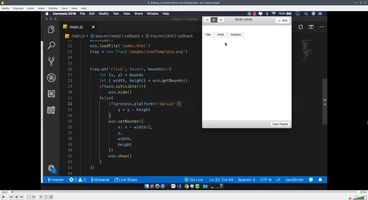
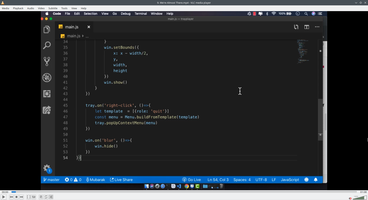
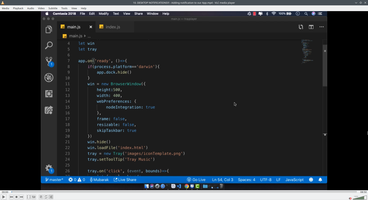
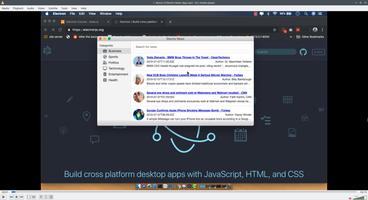
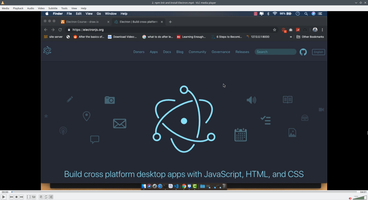
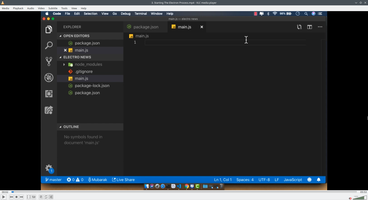
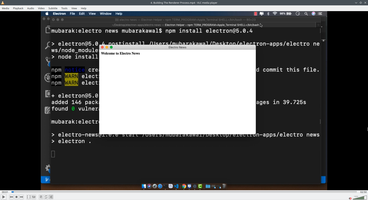
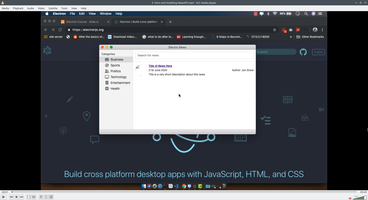
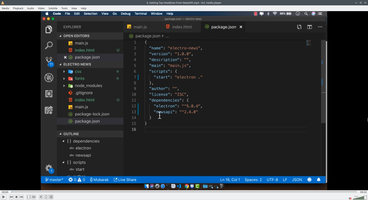
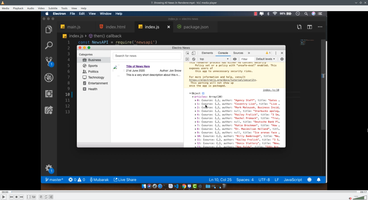
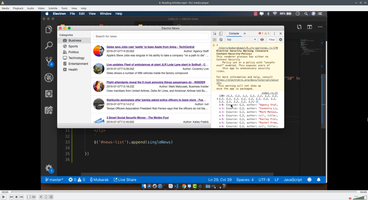
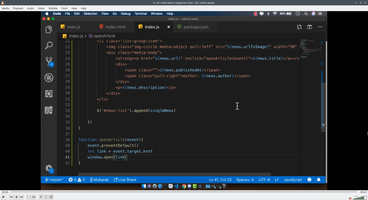
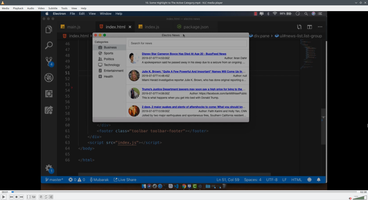
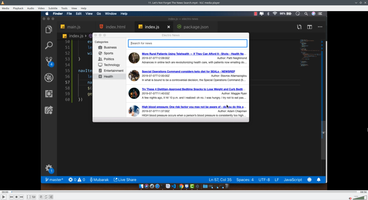
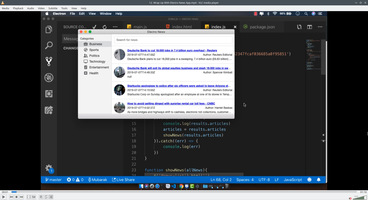
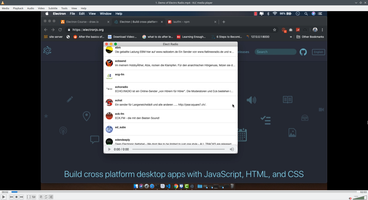
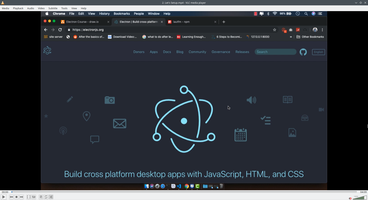
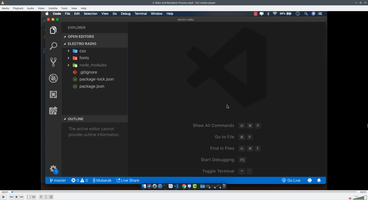
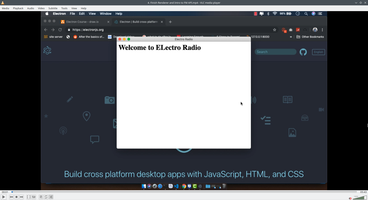
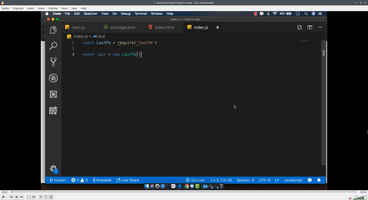
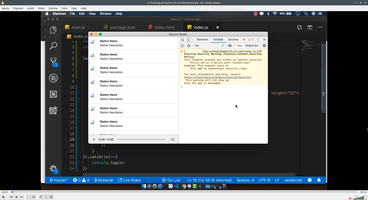
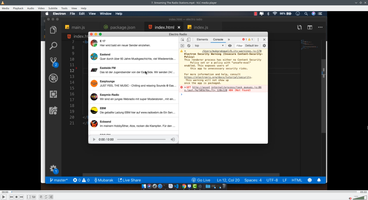
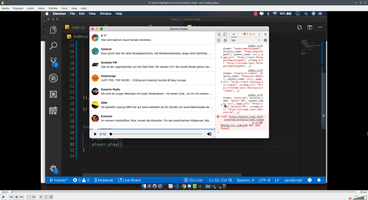
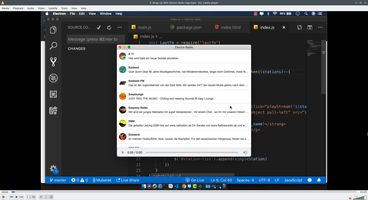
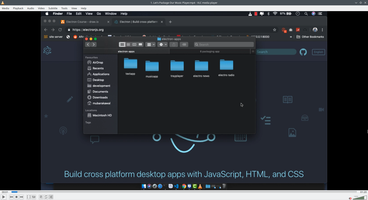
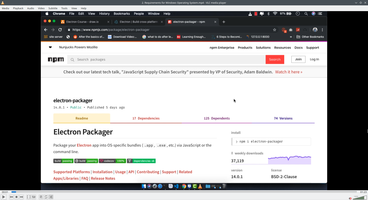
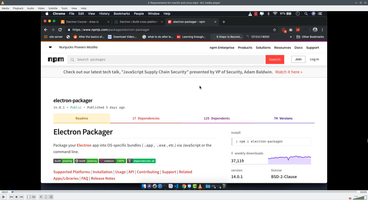
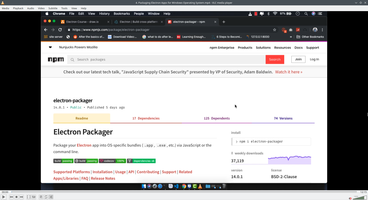
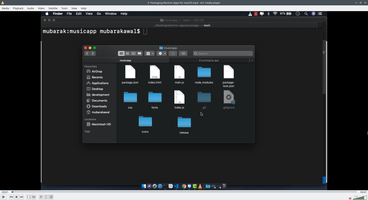
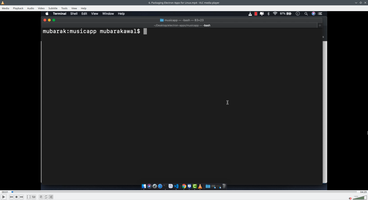
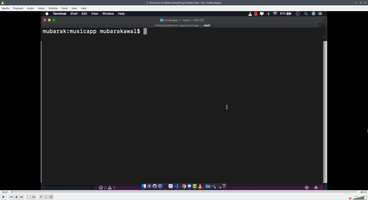
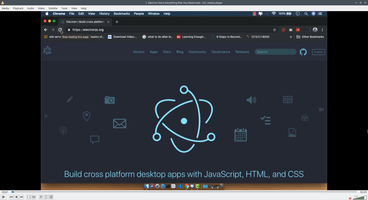
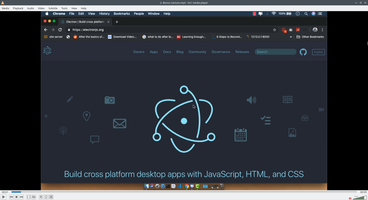
5. Yoris A. Getting started with Electron.js 2022
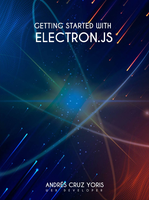
Related page:
- (2023) CloudflareWorker and Supabase
- (2022) NativeScript, AndroidBooks
- (2022) JS, Css
- (2022) Typescript, Webpack
- (2022) Angular, RxJs, Firebase, MongoDb
- (2022) Node, NestJs, Electron, Pwa, Telegram
- (2022) React, Redux, GraphQL, NextJs
- (2022) Angular/Typescript, JS books
Comments (
)

<00>
<01>
<02>
<03>
<04>
<05>
<06>
<07>
<08>
<09>
<10>
<11>
<12>
<13>
<14>
<15>
<16>
<17>
<18>
<19>
<20>
<21>
<22>
<23>
Link to this page:
http://www.vb-net.com/ElectronLearning/Index.htm
<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |