The simplest way to fill catalog in DB from VB.NET
Microsoft expand Visual Studio and .Framework functionality in most unnecessary and unexpected way. For example, in Entity Framework is appear technology of deploy DB from code. For full understanding and using this new technology need to read dozens of documentation pages and number of times try to implement MS method to reach a positive result. At common Microsoft way I describe in page - Entity Framework missing FAQ (Part 4).
But in this page I describe oldest and simplest method to deploy catalogue data from VB code to DB - use XML capability of Visual Studio in VB.
For deploy data to empty DB I use code below, this is a simplest way as possible:
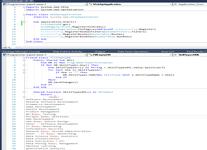
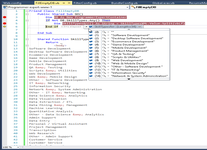
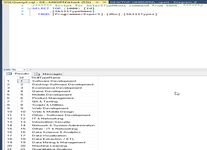
1: Friend Class FillEmptyDB
2: Public Shared Sub GO()
3: Dim DB As New ProgrammerExpertContainer
4: If Not DB.SkillTypes.Any() Then
5: Dim SkillTypesArr() As String = SkillTypesXML.Value.Split(vbLf)
6: For Each One In SkillTypesArr
7: If One <> "" Then
8: DB.SkillTypes.Add(New SkillType With {.SkillTypeName = One})
9: End If
10: Next
11: DB.SaveChanges()
12: End If
13: End Sub
14:
15: Shared Function SkillTypesXML() As XElement
16: Return _
17: <body>
18: Software Development
...
44: Technical Support
45: </body>
46: End Function
For the same way with using XML I follow to generate gibberish for site debugging. Below you may see MVC-helper extension function.
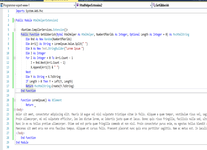
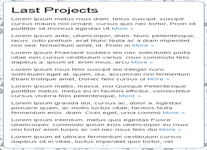
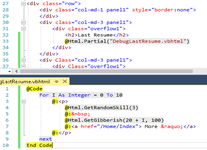
1: Imports System.Web.Mvc
2:
3: Public Module HtmlHelperExtension2
4:
5: <Runtime.CompilerServices.Extension()>
6: Public Function GetGibberish(ByVal htmlHelper As HtmlHelper, NumberOfWorlds As Integer, Optional Length As Integer = 0) As MvcHtmlString
7: Dim RandomInt As Integer = CInt((Now.Ticks And UInt32.MaxValue) >> 4)
8: Dim Rnd As New Random(RandomInt / NumberOfWorlds)
9: Dim Arr1() As String = LoremIpsum.Value.Split(" ")
10: Dim X As New Text.StringBuilder("Lorem Ipsum ")
11: Dim J As Integer
12: For I As Integer = 0 To Arr1.Count - 1
13: J = Rnd.Next(Arr1.Count - 1)
14: X.Append(Arr1(J) & " ")
15: Next
16: Dim Y As String = X.ToString
17: If Length > 0 Then Y = Left(Y, Length)
18: Return MvcHtmlString.Create(Y.ToString)
19: End Function
20:
21: Function LoremIpsum() As XElement
22: Return _
23: <body>
24: dolor sit amet, ...
25: Proin ullamcorper...
26: Nunc in ex eu ...
27: Maecenas sit amet ...
28: </body>
30: End Module
Moreover, in Seed method, where migration is doing as VisualStudio command "Update-Database", there are some alternative way to seed data. Microsoft way you may see in screen below.
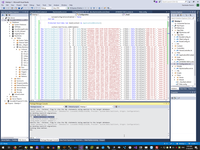
But in this way there are more traditional way - is use SQL stored procedures from separate .SQL files, for example see screen below.
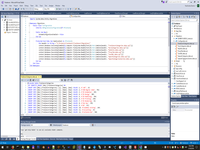
1: Imports System.Data.Entity.Migrations
2:
3: Namespace Migrations
4: Public Class Configuration
5: Inherits DbMigrationsConfiguration(Of EFContext)
6:
7: Public Sub New()
8: AutomaticMigrationsEnabled = False
9: End Sub
10:
11: Protected Overrides Sub Seed(context As EFContext)
12: Dim baseDir As String = "E:\Projects\Freelancer\Freelancer\Migrations\"
13: context.Database.ExecuteSqlCommand(My.Computer.FileSystem.ReadAllText(IO.Path.Combine(baseDir, "FreelancerCategories.data.sql")))
14: context.Database.ExecuteSqlCommand(My.Computer.FileSystem.ReadAllText(IO.Path.Combine(baseDir, "GuruCategories.data.sql")))
15: context.Database.ExecuteSqlCommand(My.Computer.FileSystem.ReadAllText(IO.Path.Combine(baseDir, "MySkills.data.sql")))
16: context.Database.ExecuteSqlCommand(My.Computer.FileSystem.ReadAllText(IO.Path.Combine(baseDir, "ProxyTab.data.sql")))
17: context.Database.ExecuteSqlCommand(My.Computer.FileSystem.ReadAllText(IO.Path.Combine(baseDir, "TestURL.data.sql")))
18: context.Database.ExecuteSqlCommand(My.Computer.FileSystem.ReadAllText(IO.Path.Combine(baseDir, "UpworkCategories.data.sql")))
19: context.Database.ExecuteSqlCommand(My.Computer.FileSystem.ReadAllText(IO.Path.Combine(baseDir, "UpworkCategoriesSection.data.sql")))
20: End Sub
21: End Class
22: End Namespace
Additionally disadvantage of Microsoft way (doing Seed from VS Command Line) is undefined class Httpcontext. This cause do impossible to copy file for relative site path. For example, in order to initialization DB I have to copy some files from resource folder to "~/App_Data/UserGuid/" folder.
1: Imports Programmer_expert_www_1.Model
2:
33:
34: Public Shared Function ImageToByteArray(imageIn As System.Drawing.Image, Format As Drawing.Imaging.ImageFormat) As Byte()
35: Dim ms As New IO.MemoryStream()
36: imageIn.Save(ms, Format)
37: Return ms.ToArray()
38: End Function
510: Public Shared Sub AddResume1()
511: Dim UserRootDir As String = HttpContext.Current.Server.MapPath("~/App_Data/" & "95CB5769-5ECF-4B42-9101-711A7BD26B51" & "/")
512: If Not My.Computer.FileSystem.DirectoryExists(UserRootDir) Then
513: My.Computer.FileSystem.CreateDirectory(UserRootDir)
514: End If
515: My.Computer.FileSystem.WriteAllBytes(IO.Path.Combine(UserRootDir, "Ava-1.gif"), ImageToByteArray(My.Resources.Ava_1, Drawing.Imaging.ImageFormat.Gif), False)
516: My.Computer.FileSystem.WriteAllBytes(IO.Path.Combine(UserRootDir, "Resume-AlexEv.pdf"), My.Resources.Resume_AlexEv, False)
517:
518: Dim Resume1 As ResumeRow = New ResumeRow With {
519: .Id = Guid.Parse("95CB5769-5ECF-4B42-9101-711A7BD26B51"),
520: .CrDate = Now(),
span class="kwrd">Sub
675:
676: End Class
But if you want to copy files in Seed and call Seed in untradiotional microsoft way (from Visual Studio Command Line) these resource copy operation is impossible.
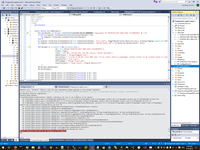

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |