Create a Web API with ASP.NET Core MVC and Visual Studio Code on Linux, macOS, and Windows
By Rick Anderson and Mike Wasson
In this tutorial, you’ll build a web API for managing a list of “to-do” items. You won’t build a UI.
There are 3 versions of this tutorial:
- macOS, Linux, Windows: Web API with Visual Studio Code (This tutorial)
- macOS: (xref:)Web API with Visual Studio for Mac
- Windows: (xref:)Web API with Visual Studio for Windows
[!INCLUDEtemplate files]
Set up your development environment
Download and install: - .NET Core 2.0.0 SDK or later. - Visual Studio Code - Visual Studio Code C# extension
Create the project
From a console, run the following commands:
mkdir TodoApi
cd TodoApi
dotnet new webapi
Open the TodoApi folder in Visual Studio Code (VS Code) and select the Startup.cs file.
- Select Yes to the Warn message “Required assets to build and debug are missing from ‘TodoApi’. Add them?”
- Select Restore to the Info message “There are unresolved dependencies”.
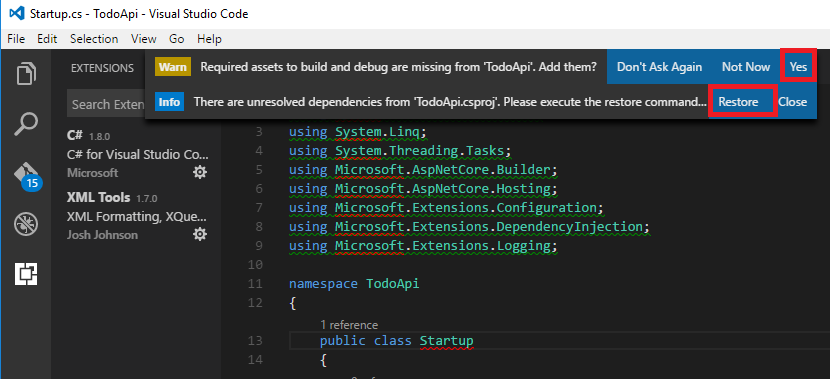
Press Debug (F5) to build and run the program. In a browser navigate to http://localhost:5000/api/values . The following is displayed:
["value1","value2"]
See Visual Studio Code help for tips on using VS Code.
Add support for Entity Framework Core
Edit the TodoApi.csproj file to install the Entity Framework Core InMemory database provider. This database provider allows Entity Framework Core to be used with an in-memory database.
[!code-xmlMain]
1: <Project Sdk="Microsoft.NET.Sdk.Web">
2: <PropertyGroup>
3: <TargetFramework>netcoreapp1.1</TargetFramework>
4: </PropertyGroup>
5: <ItemGroup>
6: <Folder Include="wwwroot\" />
7: </ItemGroup>
8: <ItemGroup>
9: <PackageReference Include="Microsoft.AspNetCore" Version="1.1.1" />
10: <PackageReference Include="Microsoft.AspNetCore.Mvc" Version="1.1.2" />
11: <PackageReference Include="Microsoft.Extensions.Logging.Debug" Version="1.1.1" />
12: <PackageReference Include="Microsoft.EntityFrameworkCore.InMemory" Version="1.1.1" />
13: </ItemGroup>
14: </Project>
Run dotnet restore
to download and install the EF Core InMemory DB provider. You can run dotnet restore
from the terminal or enter ⌘⇧P
(macOS) or Ctrl+Shift+P
(Linux) in VS Code and then type .NET. Select .NET: Restore Packages.
Add a model class
A model is an object that represents the data in your application. In this case, the only model is a to-do item.
Add a folder named Models. You can put model classes anywhere in your project, but the Models folder is used by convention.
Add a TodoItem
class with the following code:
[!code-csharpMain]
1: namespace TodoApi.Models
2: {
3: public class TodoItem
4: {
5: public long Id { get; set; }
6: public string Name { get; set; }
7: public bool IsComplete { get; set; }
8: }
9: }
The database generates the Id
when a TodoItem
is created.
Create the database context
The database context is the main class that coordinates Entity Framework functionality for a given data model. You create this class by deriving from the Microsoft.EntityFrameworkCore.DbContext
class.
Add a TodoContext
class in the Models folder:
[!code-csharpMain]
1: using Microsoft.EntityFrameworkCore;
2:
3: namespace TodoApi.Models
4: {
5: public class TodoContext : DbContext
6: {
7: public TodoContext(DbContextOptions<TodoContext> options)
8: : base(options)
9: {
10: }
11:
12: public DbSet<TodoItem> TodoItems { get; set; }
13:
14: }
15: }
[!INCLUDERegister the database context]
Add a controller
In the Controllers folder, create a class named TodoController
. Add the following code:
[!INCLUDEcode and get todo items]
Launch the app
In VS Code, press F5 to launch the app. Navigate to http://localhost:5000/api/todo (The Todo
controller we just created).
[!INCLUDElast part of web API]
Visual Studio Code help
[!INCLUDEnext steps]