Tutorial: Getting Started with SignalR 2
This tutorial shows how to use SignalR to create a real-time chat application. You will add SignalR to an empty ASP.NET web application and create an HTML page to send and display messages.
Software versions used in the tutorial
- Visual Studio 2013
- .NET 4.5
- SignalR version 2
Using Visual Studio 2012 with this tutorial
To use Visual Studio 2012 with this tutorial, do the following:
- Update your Package Manager to the latest version.
- Install the Web Platform Installer.
- In the Web Platform Installer, search for and install ASP.NET and Web Tools 2013.1 for Visual Studio 2012. This will install Visual Studio templates for SignalR classes such as Hub.
- Some templates (such as OWIN Startup Class) will not be available; for these, use a Class file instead.
Tutorial versions
For information about earlier versions of SignalR, see SignalR Older Versions.
Questions and comments
Please leave feedback on how you liked this tutorial and what we could improve in the comments at the bottom of the page. If you have questions that are not directly related to the tutorial, you can post them to the ASP.NET SignalR forum or StackOverflow.com.
Overview
This tutorial introduces SignalR development by showing how to build a simple browser-based chat application. You will add the SignalR library to an empty ASP.NET web application, create a hub class for sending messages to clients, and create an HTML page that lets users send and receive chat messages. For a similar tutorial that shows how to create a chat application in MVC 5 using an MVC view, see Getting Started with SignalR 2 and MVC 5.
[!NOTE] This tutorial demonstrates how to create SignalR applications in version 2. For details on changes between SignalR 1.x and 2, see Upgrading SignalR 1.x Projects and Visual Studio 2013 Release Notes.
SignalR is an open-source .NET library for building web applications that require live user interaction or real-time data updates. Examples include social applications, multiuser games, business collaboration, and news, weather, or financial update applications. These are often called real-time applications.
SignalR simplifies the process of building real-time applications. It includes an ASP.NET server library and a JavaScript client library to make it easier to manage client-server connections and push content updates to clients. You can add the SignalR library to an existing ASP.NET application to gain real-time functionality.
The tutorial demonstrates the following SignalR development tasks:
- Adding the SignalR library to an ASP.NET web application.
- Creating a hub class to push content to clients.
- Creating an OWIN startup class to configure the application.
- Using the SignalR jQuery library in a web page to send messages and display updates from the hub.
The following screen shot shows the chat application running in a browser. Each new user can post comments and see comments added after the user joins the chat.
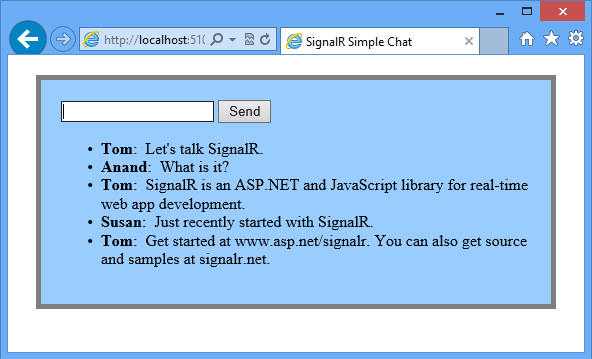
Sections:
Set up the Project
This section shows how to use Visual Studio 2013 and SignalR version 2 to create an empty ASP.NET web application, add SignalR, and create the chat application.
Prerequisites:
- Visual Studio 2013. If you do not have Visual Studio, see ASP.NET Downloads to get the free Visual Studio 2013 Express Development Tool.
The following steps use Visual Studio 2013 to create an ASP.NET Empty Web Application and add the SignalR library:
In Visual Studio, create an ASP.NET Web Application.
In the New ASP.NET Project window, leave Empty selected and click Create Project.
In Solution Explorer, right-click the project, select Add | SignalR Hub Class (v2). Name the class ChatHub.cs and add it to the project. This step creates the ChatHub class and adds to the project a set of script files and assembly references that support SignalR.
[!NOTE] You can also add SignalR to a project by opening the Tools | Library Package Manager | Package Manager Console and running a command:
install-package Microsoft.AspNet.SignalR
If you use the console to add SignalR, create the SignalR hub class as a separate step after you add SignalR.
[!NOTE] If you are using Visual Studio 2012, the SignalR Hub Class (v2) template will not be available. You can add a plain Class called
ChatHub
instead.- In Solution Explorer, expand the Scripts node. Script libraries for jQuery and SignalR are visible in the project.
Replace the code in the new ChatHub class with the following code.
[!code-csharpMain]1: using System;
2: using System.Web;
3: using Microsoft.AspNet.SignalR;
4: namespace SignalRChat
5: {
6: public class ChatHub : Hub
7: {
8: public void Send(string name, string message)
9: {
10: // Call the broadcastMessage method to update clients.
11: Clients.All.broadcastMessage(name, message);
12: }
13: }
14: }
In Solution Explorer, right-click the project, then click Add | OWIN Startup Class. Name the new class
Startup
and click OK.[!NOTE] If you are using Visual Studio 2012, the OWIN Startup Class template will not be available. You can add a plain Class called
Startup
instead.Change the contents of the new Startup class to the following.
[!code-csharpMain]1: using Microsoft.Owin;
2: using Owin;
3: [assembly: OwinStartup(typeof(SignalRChat.Startup))]
4: namespace SignalRChat
5: {
6: public class Startup
7: {
8: public void Configuration(IAppBuilder app)
9: {
10: // Any connection or hub wire up and configuration should go here
11: app.MapSignalR();
12: }
13: }
14: }
- In Solution Explorer, right-click the project, then click Add | HTML Page. Name the new page
Index.html
. >[!NOTE] >You might need to change the version numbers for the references to JQuery and SignalR libraries - In Solution Explorer, right-click the HTML page you just created and click Set as Start Page.
Replace the default code in the HTML page with the following code.
[!NOTE] A later version of the SignalR scripts may be installed by the package manager. Verify that the script references below correspond to the versions of the script files in the project (they will be different if you added SignalR using NuGet rather than adding a hub.)
1: <!DOCTYPE html>
2: <html>
3: <head>
4: <title>SignalR Simple Chat</title>
5: <style type="text/css">
6: .container {
7: background-color: #99CCFF;
8: border: thick solid #808080;
9: padding: 20px;
10: margin: 20px;
11: }
12: </style>
13: </head>
14: <body>
15: <div class="container">
16: <input type="text" id="message" />
17: <input type="button" id="sendmessage" value="Send" />
18: <input type="hidden" id="displayname" />
19: <ul id="discussion">
20: </ul>
21: </div>
22: <!--Script references. -->
23: <!--Reference the jQuery library. -->
24: <script src="Scripts/jquery-3.1.1.min.js" ></script>
25: <!--Reference the SignalR library. -->
26: <script src="Scripts/jquery.signalR-2.2.1.min.js"></script>
27: <!--Reference the autogenerated SignalR hub script. -->
28: <script src="signalr/hubs"></script>
29: <!--Add script to update the page and send messages.-->
30: <script type="text/javascript">
31: $(function () {
32: // Declare a proxy to reference the hub.
33: var chat = $.connection.chatHub;
34: // Create a function that the hub can call to broadcast messages.
35: chat.client.broadcastMessage = function (name, message) {
36: // Html encode display name and message.
37: var encodedName = $('<div />').text(name).html();
38: var encodedMsg = $('<div />').text(message).html();
39: // Add the message to the page.
40: $('#discussion').append('<li><strong>' + encodedName
41: + '</strong>: ' + encodedMsg + '</li>');
42: };
43: // Get the user name and store it to prepend to messages.
44: $('#displayname').val(prompt('Enter your name:', ''));
45: // Set initial focus to message input box.
46: $('#message').focus();
47: // Start the connection.
48: $.connection.hub.start().done(function () {
49: $('#sendmessage').click(function () {
50: // Call the Send method on the hub.
51: chat.server.send($('#displayname').val(), $('#message').val());
52: // Clear text box and reset focus for next comment.
53: $('#message').val('').focus();
54: });
55: });
56: });
57: </script>
58: </body>
59: </html>
Save All for the project.
Run the Sample
Press F5 to run the project in debug mode. The HTML page loads in a browser instance and prompts for a user name.
- Enter a user name.
- Copy the URL from the address line of the browser and use it to open two more browser instances. In each browser instance, enter a unique user name.
In each browser instance, add a comment and click Send. The comments should display in all browser instances.
[!NOTE] This simple chat application does not maintain the discussion context on the server. The hub broadcasts comments to all current users. Users who join the chat later will see messages added from the time they join.
The following screen shot shows the chat application running in three browser instances, all of which are updated when one instance sends a message:
In Solution Explorer, inspect the Script Documents node for the running application. There is a script file named hubs that the SignalR library dynamically generates at runtime. This file manages the communication between jQuery script and server-side code.
Examine the Code
The SignalR chat application demonstrates two basic SignalR development tasks: creating a hub as the main coordination object on the server, and using the SignalR jQuery library to send and receive messages.
SignalR Hubs
In the code sample the ChatHub class derives from the Microsoft.AspNet.SignalR.Hub class. Deriving from the Hub class is a useful way to build a SignalR application. You can create public methods on your hub class and then access those methods by calling them from scripts in a web page.
In the chat code, clients call the ChatHub.Send method to send a new message. The hub in turn sends the message to all clients by calling Clients.All.broadcastMessage.
The Send method demonstrates several hub concepts :
- Declare public methods on a hub so that clients can call them.
- Use the Microsoft.AspNet.SignalR.Hub.Clients dynamic property to access all clients connected to this hub.
Call a function on the client (such as the
broadcastMessage
function) to update clients.[!code-csharpMain]
1: public class ChatHub : Hub
2: {
3: public void Send(string name, string message)
4: {
5: // Call the broadcastMessage method to update clients.
6: Clients.All.broadcastMessage(name, message);
7: }
8: }
SignalR and jQuery
The HTML page in the code sample shows how to use the SignalR jQuery library to communicate with a SignalR hub. The essential tasks in the code are declaring a proxy to reference the hub, declaring a function that the server can call to push content to clients, and starting a connection to send messages to the hub.
The following code declares a reference to a hub proxy.
[!code-javascriptMain]
1: var chat = $.connection.chatHub;
[!NOTE] In JavaScript the reference to the server class and its members is in camel case. The code sample references the C# ChatHub class in JavaScript as chatHub.
The following code is how you create a callback function in the script. The hub class on the server calls this function to push content updates to each client. The two lines that HTML encode the content before displaying it are optional and show a simple way to prevent script injection.
[!code-htmlMain]
1: chat.client.broadcastMessage = function (name, message) {
2: // Html encode display name and message.
3: var encodedName = $('<div />').text(name).html();
4: var encodedMsg = $('<div />').text(message).html();
5: // Add the message to the page.
6: $('#discussion').append('<li><strong>' + encodedName
7: + '</strong>: ' + encodedMsg + '</li>');
8: };
The following code shows how to open a connection with the hub. The code starts the connection and then passes it a function to handle the click event on the Send button in the HTML page.
[!NOTE] This approach ensures that the connection is established before the event handler executes.
[!code-javascriptMain]
1: $.connection.hub.start().done(function () {
2: $('#sendmessage').click(function () {
3: // Call the Send method on the hub.
4: chat.server.send($('#displayname').val(), $('#message').val());
5: // Clear text box and reset focus for next comment.
6: $('#message').val('').focus();
7: });
8: });
Next Steps
You learned that SignalR is a framework for building real-time web applications. You also learned several SignalR development tasks: how to add SignalR to an ASP.NET application, how to create a hub class, and how to send and receive messages from the hub.
For a walkthrough on how to deploy the sample SignalR application to Azure, see Using SignalR with Web Apps in Azure App Service. For detailed information about how to deploy a Visual Studio web project to a Windows Azure Web Site, see Create an ASP.NET web app in Azure App Service.
To learn more advanced SignalR developments concepts, visit the following sites for SignalR source code and resources:

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |