Windows service example
I was written a lot of various WinServices, but still have no page with description how to do this type of application. In this page I wan to discuss about WinService. So we can start from WinService template:
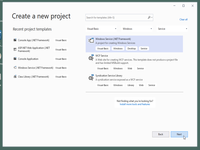
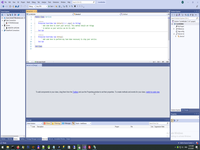
and type this common service template:
1: Imports System.Threading
2: Imports System.Timers
3: Imports Timer = System.Timers.Timer
4: Imports System.IO
5: Imports System.Text
6:
7: Public Class PromocodeCacheCreater
8: Private Timer As Timer
9: Protected Overrides Async Sub OnStart(ByVal args() As String)
10: Try
11: ReStart:
12: Timer = New Timer(CInt(My.Settings.UpdateIntervalMinutes) * 60 * 1000) 'milliseconds
13: AddHandler Timer.Elapsed, AddressOf TimerElapsed
14: Timer.Start()
15: Await BuildCache()
16: Catch ex As Exception
17: EventLog.WriteEntry("PromocodeCacheCreater", "Exception in OnStart " & IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace)
18: My.Computer.FileSystem.WriteAllText(My.Settings.OutPathRoot & "Index.htm", "Exception in OnStart " & IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace, False)
19: WriteLog($"Exception in OnStart & {IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace} {vbCrLf}")
20: GoTo ReStart
21: End Try
22: End Sub
23: Private Async Sub TimerElapsed(Sender As Object, E As ElapsedEventArgs)
24: Await BuildCache()
25: End Sub
26:
27: Protected Overrides Async Sub OnStop()
28: Try
29: If Timer IsNot Nothing AndAlso Timer.Enabled Then
30: Timer.Stop()
31: WriteLog($"{Now} ReStart {vbCrLf}")
32: Timer.Start()
33: Await BuildCache()
34: End If
35: Catch Ex As Exception
36: EventLog.WriteEntry("PromocodeCacheCreater", "Exception in OnStop " & IIf(String.IsNullOrWhiteSpace(Ex.Message), "Empty", Ex.Message) & Environment.NewLine & Ex.StackTrace)
37: My.Computer.FileSystem.WriteAllText(My.Settings.OutPathRoot & "Index.htm", "Exception in OnStop " & IIf(String.IsNullOrWhiteSpace(Ex.Message), "Empty", Ex.Message) & Environment.NewLine & Ex.StackTrace, False)
38: WriteLog($"Exception in OnStop & {IIf(String.IsNullOrWhiteSpace(Ex.Message), "Empty", Ex.Message) & Environment.NewLine & Ex.StackTrace} {vbCrLf}")
39: End Try
40: End Sub
41:
42: Public Shared TargetServerPath As String
43: Public Shared TargetServerRoot As String
44: Public Shared UrlList As List(Of String)
45: Public Shared IsUrlCollected As Boolean = False
46:
47: Async Function BuildCache() As Task
48: WriteLog($"{Now} Start {vbCrLf}")
49: Dim Pos2 As Integer = My.Settings.CollectURL.LastIndexOf("/")
50: TargetServerPath = My.Settings.CollectURL.Substring(0, Pos2)
51: Dim TargetURI As Uri = New Uri(My.Settings.CollectURL)
52: TargetServerRoot = $"{TargetURI.Scheme}://{TargetURI.Host}"
53: Try
54: Dim HTML As String = Await ReadHtml(My.Settings.MainPage)
55: If String.IsNullOrEmpty(HTML) Then Return
56: UrlList = New List(Of String)
57: IsUrlCollected = False
58: Await Task.Run(Sub() ProcessingHTML(HTML))
59: My.Computer.FileSystem.WriteAllText(My.Settings.OutPathRoot & "Index.htm", HTML, False)
60: WriteLog($"{Now} cache created {My.Settings.OutPathRoot & "Index.htm"} ({HTML.Length} chars){vbCrLf}")
61: Catch ex As Exception
62: My.Computer.FileSystem.WriteAllText(My.Settings.OutPathRoot & "Index.htm", "Exception in BuildCache " & My.Settings.CollectURL & " " & IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace, False)
63: WriteLog($"Exception in BuildCache {My.Settings.CollectURL} {IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace} {vbCrLf}")
64: End Try
65: '
66: Try
67: Dim HTML As String = Await ReadHtml(My.Settings.CollectURL)
68: If String.IsNullOrEmpty(HTML) Then Return
69: UrlList = New List(Of String)
70: IsUrlCollected = True
71: Await Task.Run(Sub() ProcessingHTML(HTML))
72: Catch ex As Exception
73: WriteLog($"Exception in BuildCache {My.Settings.CollectURL} {IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace} {vbCrLf}")
74: End Try
75: '
76: UrlList.Sort()
77: WriteLog($"{Now} fount {UrlList.Count} URLs " & vbCrLf & $"{String.Join(",", UrlList)}" & vbCrLf)
78: '
79: Dim IISvDir As New List(Of String)
80: Dim PhysPath As New List(Of String)
81: IsUrlCollected = False
82: Dim J As Integer = 0
83: For Each OneLink As String In UrlList
84: If OneLink.StartsWith("/") And OneLink.Trim <> "/" And OneLink.Trim <> "#" And Not OneLink.Contains(vbCr) And Not OneLink.Contains(vbTab) And Not OneLink.Contains(vbLf) Then
85: ' ++ debug
86: 'If J < 10 Then
87: ' J += 1
88: 'Else
89: ' Exit For
90: 'End If
91: ' ++
92: Dim CurLink As String = (My.Settings.MainPage & OneLink).Replace("//", "/").Replace("https:/", "https://").Replace("http:/", "http://")
93: Dim CurDir As String = (My.Settings.OutPathRoot & OneLink.Replace("/", "\")).Replace("\\", "\")
94: IISvDir.Add(OneLink)
95: PhysPath.Add(CurDir)
96: Debug.Print(CurLink)
97: Try
98: Dim HTML1 As String = Await ReadHtml(CurLink)
99: Await Task.Run(Sub() ProcessingHTML(HTML1))
100: If Not My.Computer.FileSystem.DirectoryExists(CurDir) Then
101: My.Computer.FileSystem.CreateDirectory(CurDir)
102: End If
103: My.Computer.FileSystem.WriteAllText(CurDir & "Index.htm", HTML1, False)
104: WriteLog($"{Now} cache created {CurDir & "Index.htm"} ({HTML1.Length} chars){vbCrLf}")
105: HTML1 = Nothing
106: Thread.Sleep(CInt(My.Settings.DelaySec) * 1000)
107: Catch ex As Exception
108: My.Computer.FileSystem.WriteAllText(CurDir & "Index.htm", "Exception in BuildCache " & CurLink & " " & IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace, False)
109: WriteLog($"Exception in BuildCache {CurLink} {IIf(String.IsNullOrWhiteSpace(ex.Message), "Empty", ex.Message) & Environment.NewLine & ex.StackTrace} {vbCrLf}")
110: Continue For
111: End Try
112: End If
113: Next
114: '
... '
135:
136: Sub WriteLog(Msg As String)
137: Dim Log As String = My.Computer.FileSystem.ReadAllText(My.Settings.LogFile)
138: Dim KolLines As Integer = Log.Count(Function(x) x = vbCr)
139: If KolLines > My.Settings.LogSize Then
140: Dim LastLinePos As Integer = Log.LastIndexOf(vbCr)
141: My.Computer.FileSystem.WriteAllText(My.Settings.LogFile, Log.Remove(0, LastLinePos), False)
142: End If
143: Log = Nothing
144: My.Computer.FileSystem.WriteAllText(My.Settings.LogFile, Msg, True)
145: End Sub
146:
147: End Class
There are a couple of trick for working with services. First is debugging. Directly debugging is impossible, there are need to replaces some code inside initialization.
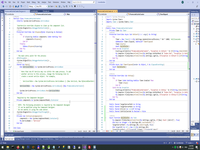
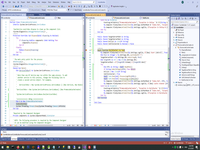
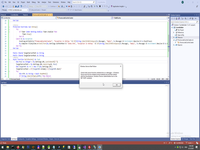
Second trick is adding a two special service to run own code as service.
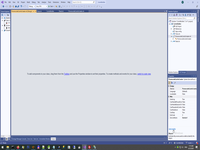
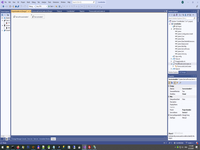
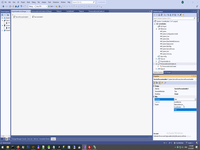
And last trick for working with service is installer service to system.
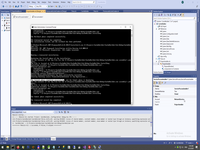
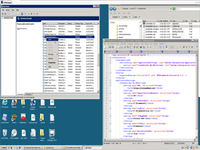
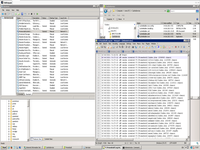
Example of windows service has been published to github https://github.com/Alex-1347/WindowsServiceExample
Comments (
)

<00>
<01>
<02>
<03>
<04>
<05>
<06>
<07>
<08>
<09>
<10>
<11>
<12>
<13>
<14>
<15>
<16>
<17>
<18>
<19>
<20>
<21>
<22>
<23>
Link to this page:
//www.vb-net.com/WindowsService/Index.htm
<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |