Asp.Net Core 6 new initialization
Firstly i want to said why initialization application and configuring services is most difficult and most important step in NET CORE. This step is absolutely absent in ASP.NET Classic and ASP.NET MVC application. Some decision from Microsoft follow increase common price of any Microsoft software (and of course induce increase Microsoft stock in stock market).
- Refuse to support VB.NET, because VB.NET is more clear and more close to human mind, than any dumb languages from 60-x last century. I write about this turning point many times in my blog Why Microsoft vilified Visual Basic ?.
- Second huge decision is extension idea. You can adding any new functions to existing Namespace and place this function to separate library. This induce that programmer never knows place of needed functions and always must be connected to internet and searching-searching-searching.
- And last difficult is embed DI container to NET CORE engine with difficult and misunderstandable parameters to configure each services usually needed to programmer.
- Each half year Microsoft changing basic conceptions and important future of it's framework and refuse to support own old idea and own old software.
As a result price of software increasing at least 10 times comparing finished monolithic framework, from $3-4/hour to $30/hour, up to $100/hour for new technology. Nobody happy for this, except Microsoft. Price of software increasing, Microsoft capitalization increasing, investment to Microsoft is growing, owners of Microsoft has more and more money.
So, in this page I describe Net core 6 new initialization (new Hosting model), https://docs.microsoft.com/en-us/aspnet/core/migration/50-to-60-samples?view=aspnetcore-6.0. This is my practical implementation of this migration.
Before Net Core 6 we was have two initialization and configuring DI container files Program.vb and Startup.vb - (this two files applicable to Backend API). Instead two files NET Core 6 uses this one file:
- Frontend like this Split code and view to different projects with ASP.NET Core 6.
- Applicable to Backend only
1: Imports BackendAPI
2: Imports BackendAPI.Model
3: Imports BackendAPI.Notification
4: Imports BackendAPI.Services
5: Imports FrontEnd.Data
6: Imports Microsoft.AspNetCore.Builder
7: Imports Microsoft.AspNetCore.Hosting
8: Imports Microsoft.AspNetCore.Http.Connections
9: Imports Microsoft.AspNetCore.HttpOverrides
10: Imports Microsoft.AspNetCore.Identity
11: Imports Microsoft.EntityFrameworkCore
12: Imports Microsoft.Extensions.Configuration
13: Imports Microsoft.Extensions.DependencyInjection
14: Imports Microsoft.Extensions.FileProviders
15: Imports Microsoft.Extensions.Hosting
16: Imports Microsoft.Extensions.Logging
17: Imports Microsoft.OpenApi.Models
18: Imports System.Diagnostics
19:
20: Module Program
21:
22: Public Property Environment As IWebHostEnvironment
23: Public Property LoggerFactory As ILoggerFactory
24: Public Property Configuration As IConfiguration
25: Sub Main(Args() As String)
26: Dim Builder = WebApplication.CreateBuilder(Args)
27: 'Add services to the container.
28: Debug.WriteLine($"ContentRootPath: {Builder.Environment.ContentRootPath}, WebRootPath: {Builder.Environment.WebRootPath}, ContentRootPath: {Builder.Environment.ContentRootPath}, StaticFilesRoot: {Builder.Configuration("StaticFilesRoot")}, WebRootFileProvider: {Builder.Environment.WebRootFileProvider}, IsDevelopment: {Builder.Environment.IsDevelopment} ")
29:
30: Builder.Host.ConfigureLogging(Sub(hostingContext, logging)
31: logging.AddConfiguration(hostingContext.Configuration.GetSection("Logging"))
32: logging.AddConsole
33: logging.AddDebug
34: logging.AddFilter("Microsoft.AspNetCore.SignalR", LogLevel.Debug)
35: logging.AddFilter("Microsoft.AspNetCore.Http.Connections", LogLevel.Debug)
36: End Sub)
37:
38: Builder.WebHost.ConfigureKestrel(Sub(KestrelServerOptions)
39: KestrelServerOptions.ListenLocalhost(5157)
40: KestrelServerOptions.ListenAnyIP(7167, Function(X) X.UseHttps)
41: End Sub)
42: Environment = Builder.Environment
43: Configuration = Builder.Configuration
44:
45: Builder.Services.AddCors
46: Builder.Services.AddControllers(Sub(MvcOptions) MvcOptions.RespectBrowserAcceptHeader = True).AddControllersAsServices
47: Builder.Services.AddHttpContextAccessor
48:
49: Builder.Services.AddMvcCore(Sub(MvcOptions) MvcOptions.EnableEndpointRouting = False).
50: AddFormatterMappings
51:
52: Builder.Services.AddSwaggerGen(Sub(SwaggerGenOptions)
53: SwaggerGenOptions.SwaggerDoc("V2", New OpenApiInfo With {.Title = "Backend API", .Version = "V2"})
54: End Sub)
55:
56: Dim AES As New AesCryptor
57:
58: Builder.Services.AddDbContext(Of ApplicationDbContext)(Function(ByVal options As DbContextOptionsBuilder)
59: Return options.UseMySql(AES.DecryptSqlConnection(Builder.Configuration.GetConnectionString("DefaultConnection"), "XXXXXXXXXX"),
60: ServerVersion.Parse("10.5.9-MariaDB-1:10.5.9+maria~xenial"), 'SHOW VARIABLES LIKE "%version%";
61: Sub(ByVal mySqlOption As Microsoft.EntityFrameworkCore.Infrastructure.MySqlDbContextOptionsBuilder)
62: mySqlOption.CommandTimeout(10)
63: mySqlOption.EnableRetryOnFailure(10)
64: End Sub)
65: End Function, ServiceLifetime.Transient, ServiceLifetime.Transient)
66:
67: ' configure strongly typed settings object
68: Builder.Services.Configure(Of Jwt.JwtSettings)(Builder.Configuration.GetSection("JwtSetting"))
69:
70: 'configure DI for application services
71: Builder.Services.AddScoped(Of IUserService, UserService)
72: Builder.Services.AddSingleton(Of IAesCryptor, AesCryptor)
73: Builder.Services.AddSingleton(Of INotificationCacheService, NotificationCacheService)
74:
75: 'SignalR
76: Builder.Services.AddSignalR.
77: AddHubOptions(Of NotificationHub)(Sub(options)
78: options.EnableDetailedErrors = True
79: End Sub)
80:
81: Builder.Services.AddDatabaseDeveloperPageExceptionFilter
82: Builder.Services.AddDefaultIdentity(Of IdentityUser)(Sub(options) options.SignIn.RequireConfirmedAccount = True).AddEntityFrameworkStores(Of ApplicationDbContext)
83: Builder.Services.AddControllersWithViews
84:
85: Dim I As Integer = 0
86: Builder.Services.OrderBy(Function(Z) Z.Lifetime.ToString).ThenBy(Function(Z) Z.ServiceType.ToString).Distinct.ToList.ForEach(Sub(X)
87: I = I + 1
88: Debug.WriteLine($"{I}. {X.Lifetime} : {X.ServiceType}")
89: End Sub)
90: 'Scoped : one instance per web request
91: 'Transient : each time the service is requested, a new instance is created
92:
93: ' ******* Configure the HTTP request pipeline. *******
94: Dim App = Builder.Build
95: LoggerFactory = App.Services.GetRequiredService(Of ILoggerFactory)
96:
97: ' EF Code First migration
98: If App.Environment.IsDevelopment Then
99: 'The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
100: App.UseMigrationsEndPoint
101: Else
102: App.UseExceptionHandler("/Home/Error")
103: App.UseHsts 'HTTPS Redirection Middleware (UseHttpsRedirection) to redirect HTTP requests to HTTPS. HSTS Middleware (UseHsts) to send HTTP Strict Transport Security Protocol (HSTS) headers to clients.
104: End If
105:
106: App.UseForwardedHeaders(New ForwardedHeadersOptions With {
107: .ForwardedHeaders = ForwardedHeaders.XForwardedFor Or ForwardedHeaders.XForwardedProto
108: })
109:
110: If Builder.Environment.IsDevelopment Then App.UseDeveloperExceptionPage
111:
112: App.UseSwagger
113: App.UseSwaggerUI(Sub(x)
114: x.SwaggerEndpoint("/swagger/V2/swagger.json", "Backend API V2") ' Notice the lack of / making it relative
115: 'x.RoutePrefix = "CS" 'This Is the reverse proxy address
116: End Sub)
117: App.UseRouting
118:
119: App.UseHttpsRedirection
120: App.UseStaticFiles(New StaticFileOptions With {.FileProvider = New PhysicalFileProvider(Builder.Configuration("StaticFilesRoot"))})
121: App.UseStaticFiles(New StaticFileOptions With {.FileProvider = New PhysicalFileProvider(Builder.Configuration("StaticFilesRoot")), .RequestPath = "/Identity"})
122: App.UseAuthentication
123: App.UseAuthorization
124: App.MapControllerRoute(name:="default", pattern:="{controller=Home}/{action=Index}/{id?}")
125: App.MapRazorPages
126:
127: 'global cors policy
128: App.UseCors(Function(x)
129: Return x.
130: AllowAnyOrigin.
131: AllowAnyMethod.
132: AllowAnyHeader
133: End Function)
134:
135: App.UseEndpoints(Function(x) x.MapControllers)
136:
137: 'custom jwt auth middleware
138: App.UseMiddleware(Of Jwt.JwtMiddleware)
139:
140: 'SignalR
141: App.UseEndpoints(Sub(endpoints)
142: endpoints.MapHub(Of NotificationHub)("/NotificationHub", Sub(opt)
143: opt.Transports =
144: HttpTransportType.WebSockets Or
145: HttpTransportType.LongPolling
146: End Sub)
147: End Sub)
148:
149: App.Run()
150:
151: End Sub
152: End Module
And frontend project has one important parameters in appsetting.json
"StaticFilesRoot": "G:\\Projects\\FrontEndTst\\FrontEndRoot"
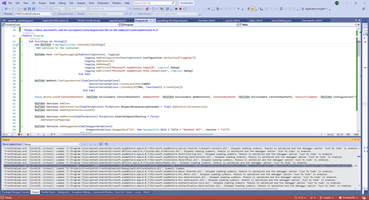
Red color mark automatically migration, of course you can doing migration manually in any projects. In this case this is ordinary migration MVC project to MsSQL - most unusable and idiotic project template in whole Microsoft templates and workflow.
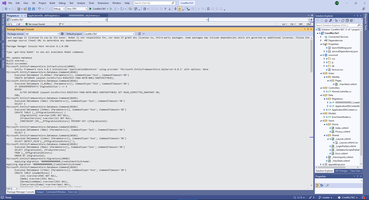
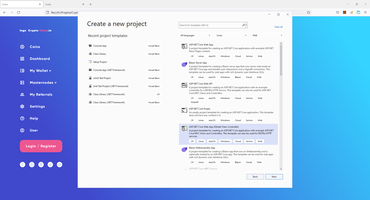
Why I mark Microsoft MVC project template as most idiotic project template as possible?
- Microsoft project templates bind to Microsoft SQL server. This is most idiotic solution as possible, because this is one SQL server in the world what is not free and need money to using.
- Microsoft project templates bind your solution to Windows, this is most idiotic solution as possible, because NET CORE specially designed with Linux. Linux most stable and absolutely free solution rather than Windows.
- Microsoft project templates mix Code and View to one project, this is most idiotic solution as possible, because we can split site to different programmers - frontend programmer and backend programmer.
- Mix Code and View to one project need to use only dumb old language C# instead improving C# contention in VB.NET.
- Microsoft project templates specially designed for learning and students. Because 99,99% solution working with existing data (database first) and EF Code First is not applicable to 99,99% real world projects.
Therefore I recommend my project template Split code and view to different projects with ASP.NET Core 6. instead idiotic Microsoft project template.

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |