Microservice with report of QEMU-KVM server (VirtualMachines, Storage, Network). Also demo of Linq, Lambda, Inheritance model.
Full code of this API I have publish to https://github.com/Alex-1347/ParseServerResponse/tree/main.
This is MySql structure of this project. Database not fit to strong relation 1:1, 1:M, M:1, M:M, for example in this project FinancialBroker - MDI application with EF code first database., because information about KVM collection be various unrelated Linux console command. Result of each command usually create one table. Therefore relation of table is confused.
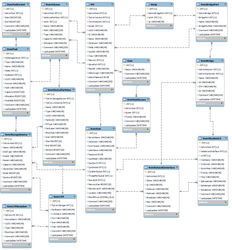
But result of this API is clear and describe state of each QEMU-KVM servers - KvmReport.json.
This is main part of my code. First part is simple buffer to read raw table from SQL.
1: Imports MySqlConnector
2:
3: Partial Module Program
4: Public Class AdmKvmBridge
5: Property i As Integer
6: Property toKvmNetwork As Nullable(Of Integer)
7: Property toKvmVlanSwitch As Nullable(Of Integer)
8: Property Name As String
9: Property Id As String
10: Property STP As String
11: Property Ip As String
12: Property Comment As String
13: Property LastUpdate As DateTime
14: End Class
15: Public Class AdmKvmDevicePartition
16: Property i As Integer
17: Property ToKvmStorageDevice As Nullable(Of Integer)
18: Property ToKvmLvmDevice As Nullable(Of Integer)
19: Property Name As String
20: Property Type As String
21: Property UUID As String
22: Property PartUUID As String
23: Property PtType As String
24: Property PartLabel As String
25: Property BlockSize As String
26: Property Size As String
27: Property Start As Long
28: Property [End] As Long
29: Property Sectors As Long
30: Property Comment As String
31: Property LastUpdate As DateTime
32: End Class
33: Public Class AdmKvmHost
34: Property i As Integer
35: Property ServerName As String
36: Property OsVersion As String
37: Property KvmVersion As String
38: Property UserName As String
39: Property Password As String
40: Property CpuModel As String
41: Property CpuCount As Integer
42: Property CpuSocket As Integer
43: Property CorePerSocket As Integer
44: Property ThreadPerSocket As Integer
45: Property NumaCell As Integer
46: Property MemorySize As Long
47: Property MainServerIP As String
48: Property Location As String
49: Property MonthPrice As Decimal
50: Property Comment As String
51: Property LastUpdate As DateTime
52: End Class
53: Public Class AdmKvmHostAccess
54: Property i As Integer
55: Property toKvmHost As Integer
56: Property toUser As Integer
57: Property FromIp As String
58: Property Comment As String
59: Property LastUpdate As DateTime
60: End Class
61: Public Class AdmKvmLVM
62: Property i As Integer
63: Property ToKvmStorage As Integer
64: Property PartName As String
65: Property LvmName As String
66: Property Fmt As String
67: Property Size As String
68: Property Free As String
69: Property Comment As String
70: Property LastUpdate As DateTime
71: End Class
72: Public Class AdmKvmLVMmember
73: Property i As Integer
74: Property toKvmLVM As String
75: Property DeviceName As String
76: Property UUID As String
77: Property Type As String
78: Property BlockSize As String
79: Property Comment As String
80: Property LastUpdate As DateTime
81: End Class
82: Public Class AdmKvmNetworkInterface
83: Property i As Integer
84: Property toKvmHost As Integer
85: Property Name As String
86: Property Ip As String
87: Property Gateway As String
88: Property Netmask As String
89: Property Broadcast As String
90: Property Mac As String
91: Property Comment As String
92: Property LastUpdate As DateTime
93: End Class
94: Public Class AdmKvmPool
95: Property i As Integer
96: Property toStorageDevice As Integer
97: Property Type As String
98: Property Name As String
99: Property State As Integer
100: Property Autostart As Integer
101: Property UUID As String
102: Property Format As String
103: Property Path As String
104: Property Capacity As Long
105: Property Allocation As Long
106: Property Available As Long
107: Property Comment As String
108: Property LastUpdate As DateTime
109: End Class
110: Public Class AdmKvmStorageDevice
111: Property i As Integer
112: Property ToKvmHost As Integer
113: Property Name As String
114: Property DiskType As String
115: Property DiskId As String
116: Property Model As String
117: Property Capacity As String
118: Property SectorSize As String
119: Property Bytes As Long
120: Property Sectors As Long
121: Property Comment As String
122: Property LastUpdate As DateTime
123: End Class
124: Public Class AdmKvmPoolExtent
125: Property i As Integer
126: Property toKvmPool As Integer
127: Property Start As Long
128: Property [End] As Long
129: Property Comment As String
130: Property LastUpdate As DateTime
131: End Class
132: Public Class AdmKvmVlanSwitch
133: Property i As Integer
134: Property toKvmHost As Integer
135: Property toNetworkInterface As Integer
136: Property id As Integer
137: Property VlanName As String
138: Property VirtSwitch As String
139: Property SwitchMac As String
140: Property FromIp As String
141: Property ToIp As String
142: Property IpBroadcast As String
143: Property IpNetmask As String
144: Property IpGateway As String
145: Property Comment As String
146: Property LastUpdate As DateTime
147: End Class
148: Public Class AdmKvmVolume
149: Property i As Integer
150: Property toKvmPool As Nullable(Of Integer)
151: Property toDevicePartition As Nullable(Of Integer)
152: Property toVm As Nullable(Of Integer)
153: Property Name As String
154: Property Path As String
155: Property Type As String
156: Property Capacity As String
157: Property Allocation As String
158: Property Comment As String
159: Property LastUpdate As DateTime
160: End Class
161: Public Class AdmUser
162: Property i As Integer
163: Property Name As String
164: Property Comment As String
165: Property LastUpdate As DateTime
166: End Class
167: Public Class AdmVM
168: Property i As Integer
169: Property toKvmHost As Integer
170: Property ToVmNetwork As Integer
171: Property toUser As Integer
172: Property UUID As String
173: Property Id As String
174: Property Name As String
175: Property OsVersion As String
176: Property State As String
177: Property CpuSet As String
178: Property Vcpu As String
179: Property Memory As Integer
180: Property SpicePort As Integer
181: Property MacAdr As String
182: Property AdminLogin As String
183: Property AdminPassword As String
184: Property Comment As String
185: Property LastUpdate As DateTime
186: End Class
187:
188: Public Class AdmKvmBridgePort
189: Property i As Integer
190: Property toKvmBridge As Integer
191: Property BridgePort As Nullable(Of Integer)
192: Property Name As String
193: Property BridgePortMac As String
194: Property Comment As String
195: Property LastUpdate As DateTime
196: End Class
197:
198: Public Class AdmVmIp
199: Property i As Integer
200: Property toKvmBridgePort As Integer
201: Property toVm As Integer
202: Property Ip As String
203: Property Comment As String
204: Property LastUpdate As DateTime
205: End Class
206:
207: Function ReadAdmKvmBridgeList(ByRef CN As MySqlConnection) As List(Of AdmKvmBridge)
208: Dim AdmKvmBridgeList As New List(Of AdmKvmBridge)
209: Dim CMD1 As MySqlCommand
210: Try
211: CMD1 = New MySqlCommand($"select * from `cryptochestmax`.`KvmBridge`;", CN)
212: Dim RDR1 As MySqlDataReader = CMD1.ExecuteReader
213: While RDR1.Read
214: AdmKvmBridgeList.Add(New AdmKvmBridge With {
215: .i = CInt(RDR1("i")),
216: .toKvmNetwork = If(IsDBNull(RDR1("toKvmNetwork")), Nothing, RDR1("toKvmNetwork")),
217: .toKvmVlanSwitch = If(IsDBNull(RDR1("toKvmVlanSwitch")), Nothing, RDR1("toKvmVlanSwitch")),
218: .Name = RDR1("Name"),
219: .Id = RDR1("Id"),
220: .STP = RDR1("STP"),
221: .Ip = RDR1("Ip"),
222: .Comment = If(IsDBNull(RDR1("Comment")), "", RDR1("Comment")),
223: .LastUpdate = CDate(RDR1("LastUpdate"))
224: })
225: End While
226: RDR1.Close()
227: Catch ex As Exception
228: Console.WriteLine(ex.Message & CMD1.CommandText)
229: CN.Close()
230: ReOpenMySQL(CN)
231: End Try
232: Return AdmKvmBridgeList
233: End Function
....
Second part is model to be serialised to JSON.
1: Imports Newtonsoft.Json
2:
3: Partial Module Program
4: Public Class KvmReport
5: Public Property KvmHostList As List(Of KvmHostReport)
6: End Class
7:
8: Public Class KvmHostReport
9: Inherits AdmKvmHost
10:
11: <JsonProperty(Order:=100)>
12: Property VM As List(Of VmReport)
13: <JsonProperty(Order:=101)>
14: Property Network As List(Of KvmNetworkReport)
15: <JsonProperty(Order:=102)>
16: Property Storage As List(Of KvmStorageReport)
17: <JsonProperty(Order:=103)>
18: Property VirtualStorage As List(Of KvmPoolReport)
19: <JsonProperty(Order:=104)>
20: Property Access As List(Of KvmHostAccessReport)
21: End Class
22:
23: Public Class KvmHostAccessReport
24: Inherits AdmKvmHostAccess
25: <JsonProperty(Order:=100)>
26: Property User As List(Of String)
27: End Class
28:
29: Public Class KvmStorageReport
30: Inherits AdmKvmStorageDevice
31: <JsonProperty(Order:=100)>
32: Property LvmStorage As List(Of KvmLVMReport)
33: <JsonProperty(Order:=101)>
34: Property PartitionStorage As List(Of KvmDevicePartitionReport)
35: End Class
36:
37: Public Class KvmLVMReport
38: Inherits AdmKvmLVM
39: <JsonProperty(Order:=100)>
40: Property LvmMember As List(Of AdmKvmLVMmember)
41: End Class
42:
43: Public Class KvmDevicePartitionReport
44: Inherits AdmKvmDevicePartition
45: End Class
46:
47: Public Class KvmNetworkReport
48: Inherits AdmKvmBridge
49: <JsonProperty(Order:=100)>
50: Property NetworkInterface As List(Of AdmKvmNetworkInterface)
51:
52: <JsonProperty(Order:=102)>
53: Property Bridge As KvmBridgeReport
54: End Class
55:
56: Public Class KvmBridgeReport
57: Property VlanSwitch As List(Of AdmKvmVlanSwitch)
58: <JsonProperty(Order:=100)>
59: Property Bridge As AdmKvmBridge
60: <JsonProperty(Order:=101)>
61: Property Port As List(Of KvmBridgePortReport)
62: End Class
63:
64: Public Class KvmBridgePortReport
65: Inherits AdmKvmBridgePort
66: <JsonProperty(Order:=100)>
67: Property IP As List(Of String)
68: End Class
69:
70: Public Class KvmPoolReport
71: Inherits AdmKvmPool 'this is ref to KvmStorageReport
72: <JsonProperty(Order:=100)>
73: Property Volume As List(Of AdmKvmVolume)
74: <JsonProperty(Order:=101)>
75: Property Extent As List(Of AdmKvmPoolExtent)
76: End Class
77:
78: Public Class VmReport
79: Inherits AdmVM
80:
81: <JsonProperty(Order:=100)>
82: Property Ip As List(Of String)
83: <JsonProperty(Order:=101)>
84: Property VmNetworkName As List(Of String) 'ref to VmNetwork from KvmNetworkReport
85: <JsonProperty(Order:=102)>
86: Property StorageDeviceName As List(Of Tuple(Of String, String))
87: <JsonProperty(Order:=103)>
88: Property UserName As String 'ref to User KvmHostAccessReport
89: End Class
90:
91: End Module
And third main part of this API is function to fill model from raw SQL-tables.
1: Imports MySqlConnector
2: Imports Newtonsoft.Json
3: Imports Newtonsoft.Json.Linq
4:
5: Partial Module Program
6: Function CreateServerReport(CN As MySqlConnection) As String
7: Dim AdmKvmReport As New KvmReport
8: Dim AdmKvmDevicePartitionList As List(Of AdmKvmDevicePartition) = ReadAdmKvmDevicePartitionList(CN)
9: Dim AdmKvmHostList As List(Of AdmKvmHost) = ReadAdmKvmHostList(CN)
10: Dim AdmKvmHostAccessList As List(Of AdmKvmHostAccess) = ReadAdmKvmHostAccessList(CN)
11: Dim AdmKvmLVMList As List(Of AdmKvmLVM) = ReadAdmKvmLVMList(CN)
12: Dim AdmKvmLVMmemberList As List(Of AdmKvmLVMmember) = ReadAdmKvmLVMmemberList(CN)
13: Dim AdmKvmNetworkInterfaceList As List(Of AdmKvmNetworkInterface) = ReadAdmKvmNetworkInterfaceList(CN)
14: Dim AdmKvmPoolList As List(Of AdmKvmPool) = ReadAdmKvmPoolList(CN)
15: Dim AdmKvmStorageDeviceList As List(Of AdmKvmStorageDevice) = ReadAdmKvmStorageDeviceList(CN)
16: Dim AdmKvmPoolExtentList As List(Of AdmKvmPoolExtent) = ReadAdmKvmPoolExtentList(CN)
17: Dim AdmKvmVlanList As List(Of AdmKvmVlanSwitch) = ReadAdmKvmVlanSwitchList(CN)
18: Dim AdmKvmVolumeList As List(Of AdmKvmVolume) = ReadAdmKvmVolumeList(CN)
19: Dim AdmUserList As List(Of AdmUser) = ReadAdmUserList(CN)
20: Dim AdmVMList As List(Of AdmVM) = ReadAdmVMList(CN)
21: Dim AdmKvmBridgeList As List(Of AdmKvmBridge) = ReadAdmKvmBridgeList(CN)
22: Dim AdmKvmBridgePortList As List(Of AdmKvmBridgePort) = ReadAdmKvmBridgePortList(CN)
23: Dim AdmVmIpList As List(Of AdmVmIp) = ReadAdmVmIpList(CN)
24:
25: AdmKvmReport.KvmHostList = New List(Of KvmHostReport)
26: For I As Integer = 0 To AdmKvmHostList.Count - 1
27: AdmKvmReport.KvmHostList.Add(New KvmHostReport With {
28: .i = AdmKvmHostList(I).i,
29: .Access = New List(Of KvmHostAccessReport),
30: .Storage = New List(Of KvmStorageReport),
31: .Network = New List(Of KvmNetworkReport),
32: .VirtualStorage = New List(Of KvmPoolReport),
33: .VM = New List(Of VmReport),
34: .OsVersion = AdmKvmHostList(I).OsVersion,
35: .ServerName = AdmKvmHostList(I).ServerName,
36: .KvmVersion = AdmKvmHostList(I).KvmVersion,
37: .UserName = AdmKvmHostList(I).UserName,
38: .Password = AdmKvmHostList(I).Password,
39: .CpuModel = AdmKvmHostList(I).CpuModel,
40: .CpuCount = AdmKvmHostList(I).CpuCount,
41: .CpuSocket = AdmKvmHostList(I).CpuSocket,
42: .CorePerSocket = AdmKvmHostList(I).CorePerSocket,
43: .ThreadPerSocket = AdmKvmHostList(I).ThreadPerSocket,
44: .NumaCell = AdmKvmHostList(I).NumaCell,
45: .MemorySize = AdmKvmHostList(I).MemorySize,
46: .MainServerIP = AdmKvmHostList(I).MainServerIP,
47: .MonthPrice = AdmKvmHostList(I).MonthPrice,
48: .Location = AdmKvmHostList(I).Location,
49: .Comment = AdmKvmHostList(I).Comment,
50: .LastUpdate = AdmKvmHostList(I).LastUpdate
51: })
52: Next
53: For I As Integer = 0 To AdmKvmHostList.Count - 1
54: 'Access - OK
55: For j As Integer = 0 To AdmKvmHostAccessList.Count - 1
56: Dim KvmHostAccessReport1 = New KvmHostAccessReport With {
57: .User = New List(Of String),
58: .i = AdmKvmHostAccessList(j).i,
59: .toKvmHost = AdmKvmHostAccessList(j).toKvmHost,
60: .toUser = AdmKvmHostAccessList(j).toUser,
61: .FromIp = AdmKvmHostAccessList(j).FromIp,
62: .Comment = AdmKvmHostAccessList(j).Comment,
63: .LastUpdate = AdmKvmHostAccessList(j).LastUpdate
64: }
65: KvmHostAccessReport1.User = New List(Of String)
66: AdmUserList.Where(Function(X) X.i = AdmKvmHostAccessList(j).toUser).ToList.ForEach(Sub(Y) KvmHostAccessReport1.User.Add(Y.Name))
67: AdmKvmReport.KvmHostList(I).Access.Add(KvmHostAccessReport1)
68: Next
69: For j As Integer = 0 To AdmKvmStorageDeviceList.Count - 1
70: Dim KvmStorageReport1 = New KvmStorageReport With {
71: .i = AdmKvmStorageDeviceList(j).i,
72: .LvmStorage = New List(Of KvmLVMReport),
73: .PartitionStorage = New List(Of KvmDevicePartitionReport),
74: .ToKvmHost = AdmKvmStorageDeviceList(j).ToKvmHost,
75: .Name = AdmKvmStorageDeviceList(j).Name,
76: .DiskType = AdmKvmStorageDeviceList(j).DiskType,
77: .DiskId = AdmKvmStorageDeviceList(j).DiskId,
78: .Model = AdmKvmStorageDeviceList(j).Model,
79: .Capacity = AdmKvmStorageDeviceList(j).Capacity,
80: .SectorSize = AdmKvmStorageDeviceList(j).SectorSize,
81: .Bytes = AdmKvmStorageDeviceList(j).Bytes,
82: .Sectors = AdmKvmStorageDeviceList(j).Sectors,
83: .Comment = AdmKvmStorageDeviceList(j).Comment,
84: .LastUpdate = AdmKvmStorageDeviceList(j).LastUpdate
85: }
86: AdmKvmLVMList.Where(Function(Y) Y.ToKvmStorage = KvmStorageReport1.i).ToList.
87: ForEach(Sub(X) KvmStorageReport1.LvmStorage.Add(
88: New KvmLVMReport With {
89: .LvmMember = New List(Of AdmKvmLVMmember),
90: .i = X.i,
91: .ToKvmStorage = X.ToKvmStorage,
92: .PartName = X.PartName,
93: .LvmName = X.LvmName,
94: .Fmt = X.Fmt,
95: .Size = X.Size,
96: .Free = X.Free,
97: .Comment = X.Comment,
98: .LastUpdate = X.LastUpdate
99: }))
100: Dim LvmPresent = KvmStorageReport1.LvmStorage.Where(Function(X) X IsNot Nothing).Select(Function(Y) New With {Y.LvmMember, Y.i}).ToList
101: For Each OneLvm In LvmPresent
102: For Each OneLvmMember In AdmKvmLVMmemberList
103: If OneLvm.i = OneLvmMember.toKvmLVM Then
104: OneLvm.LvmMember.Add(New AdmKvmLVMmember With {
105: .i = OneLvmMember.i,
106: .toKvmLVM = OneLvmMember.toKvmLVM,
107: .DeviceName = OneLvmMember.DeviceName,
108: .UUID = OneLvmMember.UUID,
109: .Type = OneLvmMember.Type,
110: .BlockSize = OneLvmMember.BlockSize,
111: .Comment = OneLvmMember.Comment,
112: .LastUpdate = OneLvmMember.LastUpdate
113: })
114: End If
115: Next
116: Next
117: Dim Partitions = AdmKvmDevicePartitionList.Where(Function(Y) Y.ToKvmStorageDevice IsNot Nothing)
118: Partitions.Where(Function(Y) Y.ToKvmStorageDevice = KvmStorageReport1.i).ToList.
119: ForEach(Sub(X) KvmStorageReport1.PartitionStorage.Add(New KvmDevicePartitionReport With {
120: .i = X.i,
121: .ToKvmStorageDevice = X.ToKvmStorageDevice,
122: .ToKvmLvmDevice = X.ToKvmLvmDevice,
123: .Name = X.Name,
124: .Type = X.Type,
125: .UUID = X.UUID,
126: .PartUUID = X.PartUUID,
127: .PtType = X.PtType,
128: .PartLabel = X.PartLabel,
129: .BlockSize = X.BlockSize,
130: .Size = X.Size,
131: .Start = X.Start,
132: .[End] = X.[End],
133: .Sectors = X.Sectors,
134: .Comment = X.Comment,
135: .LastUpdate = X.LastUpdate
136: }))
137: AdmKvmReport.KvmHostList(I).Storage.Add(KvmStorageReport1)
138: Next
139: For j As Integer = 0 To AdmKvmPoolList.Count - 1
140: Dim KvmPoolReport1 = New KvmPoolReport With {
141: .Volume = New List(Of AdmKvmVolume),
142: .Extent = New List(Of AdmKvmPoolExtent),
143: .i = AdmKvmPoolList(j).i,
144: .toStorageDevice = AdmKvmPoolList(j).toStorageDevice,
145: .Type = AdmKvmPoolList(j).Type,
146: .Name = AdmKvmPoolList(j).Name,
147: .State = AdmKvmPoolList(j).State,
148: .Autostart = AdmKvmPoolList(j).Autostart,
149: .UUID = AdmKvmPoolList(j).UUID,
150: .Format = AdmKvmPoolList(j).Format,
151: .Path = AdmKvmPoolList(j).Path,
152: .Capacity = AdmKvmPoolList(j).Capacity,
153: .Allocation = AdmKvmPoolList(j).Allocation,
154: .Available = AdmKvmPoolList(j).Available,
155: .Comment = AdmKvmPoolList(j).Comment,
156: .LastUpdate = AdmKvmPoolList(j).LastUpdate
157: }
158: Dim CurVulume = AdmKvmVolumeList.Where(Function(X) X.toKvmPool = KvmPoolReport1.i).ToList
159: CurVulume.ForEach(Sub(X) KvmPoolReport1.Volume.Add(X))
160: Dim CurExtent = AdmKvmPoolExtentList.Where(Function(X) X.toKvmPool = KvmPoolReport1.i).ToList
161: CurExtent.ForEach(Sub(X) KvmPoolReport1.Extent.Add(X))
162: AdmKvmReport.KvmHostList(I).VirtualStorage.Add(KvmPoolReport1)
163: Next
164: 'Network - ok
165: For j As Integer = 0 To AdmKvmBridgeList.Count - 1
166: Dim KvmNetworkReport1 = New KvmNetworkReport With {
167: .NetworkInterface = New List(Of AdmKvmNetworkInterface),
168: .Bridge = New KvmBridgeReport,
169: .i = AdmKvmBridgeList(j).i,
170: .toKvmNetwork = AdmKvmBridgeList(j).toKvmNetwork,
171: .toKvmVlanSwitch = AdmKvmBridgeList(j).toKvmVlanSwitch,
172: .Name = AdmKvmBridgeList(j).Name,
173: .Id = AdmKvmBridgeList(j).Id,
174: .STP = AdmKvmBridgeList(j).STP,
175: .Ip = AdmKvmBridgeList(j).Ip,
176: .Comment = AdmKvmBridgeList(j).Comment,
177: .LastUpdate = AdmKvmBridgeList(j).LastUpdate
178: }
179:
180: AdmKvmNetworkInterfaceList.Where(Function(X) X.toKvmHost = KvmNetworkReport1.i).ToList.
181: ForEach(Sub(X) KvmNetworkReport1.NetworkInterface.Add(New AdmKvmNetworkInterface With {
182: .i = X.i,
183: .toKvmHost = X.toKvmHost,
184: .Name = X.Name,
185: .Ip = X.Ip,
186: .Gateway = X.Gateway,
187: .Netmask = X.Netmask,
188: .Broadcast = X.Broadcast,
189: .Mac = X.Mac,
190: .Comment = X.Comment,
191: .LastUpdate = X.LastUpdate
192: }))
193: If KvmNetworkReport1.toKvmVlanSwitch IsNot Nothing Then
194: For k As Integer = 0 To AdmKvmBridgeList.Count - 1
195: If AdmKvmBridgeList(k).toKvmVlanSwitch IsNot Nothing Then
196: KvmNetworkReport1.Bridge = New KvmBridgeReport With {
197: .Bridge = AdmKvmBridgeList.Where(Function(X) X.toKvmVlanSwitch IsNot Nothing).Where(Function(X) X.toKvmVlanSwitch = KvmNetworkReport1.toKvmVlanSwitch).FirstOrDefault,
198: .VlanSwitch = New List(Of AdmKvmVlanSwitch),
199: .Port = New List(Of KvmBridgePortReport)}
200: AdmKvmBridgePortList.Where(Function(X) KvmNetworkReport1.Bridge.Bridge IsNot Nothing).Where(Function(X) X.toKvmBridge = KvmNetworkReport1.Bridge.Bridge.i).ToList.ForEach(Sub(X) KvmNetworkReport1.Bridge.Port.Add(New KvmBridgePortReport With {
201: .IP = AdmVmIpList.Where(Function(Z) Z.toKvmBridgePort = X.i).Select(Function(Z) Z.Ip).ToList,
202: .i = X.i,
203: .toKvmBridge = X.toKvmBridge,
204: .BridgePort = X.BridgePort,
205: .Name = X.Name,
206: .BridgePortMac = X.BridgePortMac,
207: .Comment = X.Comment,
208: .LastUpdate = X.LastUpdate
209: }))
210: KvmNetworkReport1.Bridge.VlanSwitch = AdmKvmVlanList.Where(Function(X) X.i = KvmNetworkReport1.Bridge.Bridge.toKvmVlanSwitch).ToList
211: End If
212: Next
213: End If
214:
215:
216: AdmKvmReport.KvmHostList(I).Network.Add(KvmNetworkReport1)
217: Next
218: 'VLAN-OK
219: For j As Integer = 0 To AdmVMList.Count - 1
220: Dim VmReport1 As New VmReport With {
221: .VmNetworkName = New List(Of String),
222: .Ip = New List(Of String),
223: .StorageDeviceName = New List(Of Tuple(Of String, String)),
224: .i = AdmVMList(j).i,
225: .toKvmHost = AdmVMList(j).toKvmHost,
226: .ToVmNetwork = AdmVMList(j).ToVmNetwork,
227: .toUser = AdmVMList(j).toUser,
228: .UUID = AdmVMList(j).UUID,
229: .Id = AdmVMList(j).Id,
230: .Name = AdmVMList(j).Name,
231: .OsVersion = AdmVMList(j).OsVersion,
232: .State = AdmVMList(j).State,
233: .CpuSet = AdmVMList(j).CpuSet,
234: .Vcpu = AdmVMList(j).Vcpu,
235: .Memory = AdmVMList(j).Memory,
236: .SpicePort = AdmVMList(j).SpicePort,
237: .MacAdr = AdmVMList(j).MacAdr,
238: .AdminLogin = AdmVMList(j).AdminLogin,
239: .AdminPassword = AdmVMList(j).AdminPassword,
240: .Comment = AdmVMList(j).Comment,
241: .LastUpdate = AdmVMList(j).LastUpdate
242: }
243: VmReport1.UserName = AdmUserList.Where(Function(X) X.i = VmReport1.toUser).FirstOrDefault.Name 'ref to User KvmHostAccessReport
244: VmReport1.VmNetworkName = AdmKvmBridgeList.Where(Function(X) X.i = VmReport1.ToVmNetwork).Select(Of String)(Function(X) X.Name).ToList 'ref to VmNetwork from KvmNetworkReport
245: VmReport1.Ip = AdmVmIpList.Where(Function(X) X.toVm = VmReport1.i).Select(Of String)(Function(X) X.Ip).ToList 'ref to VmNetwork from KvmNetworkReport
246: For Each One In AdmKvmVolumeList
247: If One.toVm IsNot Nothing Then
248: If One.toVm = VmReport1.i Then
249: VmReport1.StorageDeviceName.Add(New Tuple(Of String, String)(One.Path, One.Capacity))
250: End If
251:
252: End If
253: Next
254: AdmKvmReport.KvmHostList(I).VM.Add(VmReport1)
255: Next
256: Next
257: '
258: Dim JAdmKvmReport As JObject = JToken.FromObject(AdmKvmReport)
259: Dim AdmKvmStringResult As String = JsonConvert.SerializeObject(JAdmKvmReport, New JsonSerializerSettings With {.Formatting = Formatting.Indented})
260: Return AdmKvmStringResult
261: End Function
262: End Module

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |