TypeScript 2.x for Angular Developers Harness the capabilities of TypeScript to build cutting-edge web apps with Angular
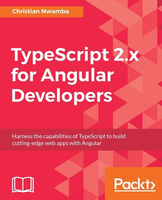
Preface
What this book covers What you need for this book Who this book is for Conventions Reader feedback Customer support Downloading the example code Downloading the color images of this book Errata Piracy QuestionsFrom Loose Types to Strict Types
Term definitions Implications of loose types The problem Mitigating loose-type problems The typeof operator The toString method Final NoteGetting Started with TypeScript
Setting up TypeScript Hello World Type annotation in TypeScript ES6 and beyond User story Final notesTypescript Native Types and Features
Basic types Strings Numbers Boolean Arrays Void Any Tuple Enums Functions and function types Function parameters Function return value Optional parameters Interfaces Interfaces for JavaScript object types Optional properties Read-only properties Interfaces as contracts Decorators Decorating methods Decorating classes Decorator factoriesUp and Running with Angular and TypeScript
Setting up Angular and TypeScript Creating a new Angular project Project structure Generating files Basics concepts Components Templates Component styles Modules Unit testingAdvanced Custom Components with TypeScript
Lifecycle hooks DOM manipulation ElementRef Hooking into content initialization Handling DOM events View encapsulation Emulated Native NoneComponent Composition with TypeScript
Component composability Hierarchical composition Component communication Parent-child flow Intercepting property changes Child–parent flow Accessing properties and methods of a child via a parent component Accessing child members with ViewChildSeparating Concerns with Typed Services
Dependency injection Data in components Data class services Component interaction with services Services as utilitiesBetter Forms and Event Handling with TypeScript
Creating types for forms The form module Two-way binding More form fields Validating the form and form fields Submitting forms Handling events Mouse events Keyboard eventsWriting Modules, Directives, and Pipes with TypeScript
Directives Attribute directives Handling events in directives Dynamic attribute directives Structural directives Why the difference? The deal with asterisks Creating structural directives Pipes Creating pipes Passing arguments to pipes ModulesClient-Side Routing for SPA
RouterModule Router directives Master-details view with routes Data source Blog service Creating routes Listing posts in the home component Reading an article with the post componentWorking with Real Hosted Data
Observables The HTTP module Building a simple todo demo app Project setup Building the API Installing diskdb Updating API endpoints Creating an Angular component Creating application routes Creating a todos service Connecting the service with our todos component Implementing the view Build a user directory with Angular Create a new Angular app Create a Node server Install diskDB Create a new component Create a service Updating user.component.ts Updating user.component.html Running the appTesting and Debugging
Angular 2 testing tools Jasmine Main concepts of Jasmine Karma Protractor Angular testing platform Using Karma (with Jasmine) Creating a new project Installing the Karma CLI Configuring Karma Testing the components Testing services Testing using HTTP Testing using MockBackend Testing a directive Testing a pipe Debugging Augury Augury features Component tree Router tree Source map
Comments (
)

<00>
<01>
<02>
<03>
<04>
<05>
<06>
<07>
<08>
<09>
<10>
<11>
<12>
<13>
<14>
<15>
<16>
<17>
<18>
<19>
<20>
<21>
<22>
<23>
Link to this page:
http://www.vb-net.com/AngularStart2/8.htm
<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |