(back) Events vs Delegates (back)
Delegates refer to methods and described as type-safe function pointers (contains type safe AddressOf).
Unlike function pointers, Visual Basic delegates are a reference type based on the class System.Delegate
The AddressOf statement implicitly creates an instance of a delegate that refers to a specific procedure
1: AddHandler Button1.Click, New EventHandler(AddressOf Button1_Click) ' the same as line below
2: AddHandler Button1.Click, AddressOf Me.Button1_Click ' the same as line above
3: 'EventHandler(AddressOf Button1_Click) - delegate
1: 'Delegate Example 1
2: Dim inOrder As New InOrderClass
3: inOrder.Num1 = 5
4: inOrder.Num2 = 4
5:
6: 'Use AddressOf to send a reference to the comparison function you want to use.
7: inOrder.ShowInOrder(AddressOf GreaterThan)
8: inOrder.ShowInOrder(AddressOf LessThan)
9:
10: 'Use lambda expressions to do the same thing.
11: inOrder.ShowInOrder(Function(m, n) m > n)
12: inOrder.ShowInOrder(Function(m, n) m < n)
13: 'Function(m, n) m < n - Delegate
14:
15:
16: Function GreaterThan(ByVal num1 As Integer, ByVal num2 As Integer) As Boolean
17: Return num1 > num2
18: End Function
19:
20: Function LessThan(ByVal num1 As Integer, ByVal num2 As Integer) As Boolean
21: Return num1 < num2
22: End Function
23:
24: Class InOrderClass
25:
26: 'Define the delegate function for the comparisons.
27: Delegate Function CompareNumbers(ByVal num1 As Integer, ByVal num2 As Integer) As Boolean
28:
29: 'Display properties in ascending or descending order.
30: Sub ShowInOrder(ByVal compare As CompareNumbers)
31: If compare(_num1, _num2) Then
32: Console.WriteLine(_num1 & " " & _num2)
33: Else
34: Console.WriteLine(_num2 & " " & _num1)
35: End If
36: End Sub
37: Property Num1() As Integer
38: Property Num2() As Integer
39: End Class
1: 'Delegate Example 2 - invoke delegate
2:
3: Delegate Function MathOperator(ByVal x As Double, ByVal y As Double) As Double
4: Function AddNumbers(ByVal x As Double, ByVal y As Double) As Double
5: Return x + y
6: End Function
7: Function SubtractNumbers(ByVal x As Double, ByVal y As Double) As Double
8: Return x - y
9: End Function
10:
11: Sub DelegateCall(ByVal x As Double, ByVal op As MathOperator, ByVal y As Double)
12: Dim ret As Double
13: ret = op.Invoke(x, y) ' Call the method.
14: MsgBox(ret)
15: End Sub
16:
17: Protected Sub Test()
18: DelegateCall(5, AddressOf AddNumbers, 3)
19: DelegateCall(9, AddressOf SubtractNumbers, 3)
20: End Sub
An Event statement implicitly defines a delegate class named
1: Delegate Sub DelegateType()
2: Event AnEvent As DelegateType
1: 'Event Example 1
2:
3: 'Declare a WithEvents variable.
4: Dim WithEvents EClass As New EventClass
5:
6: 'Declare an event handler that handles multiple events.
7: Sub EClass_EventHandler() Handles EClass.XEvent, EClass.YEvent
8: MsgBox("Received Event.")
9: End Sub
10:
11: Class EventClass
12: Public Event XEvent()
13: Public Event YEvent()
14: 'RaiseEvents raises both events.
15: Sub FlashEvents()
16: RaiseEvent XEvent()
17: RaiseEvent YEvent()
18: End Sub
19: End Class
20:
21: EClass.FlashEvents()
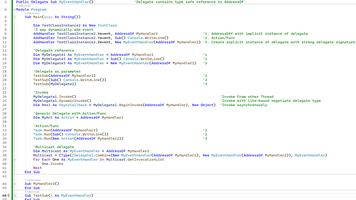
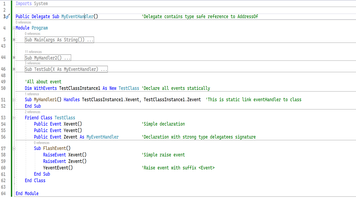
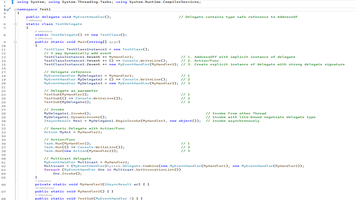
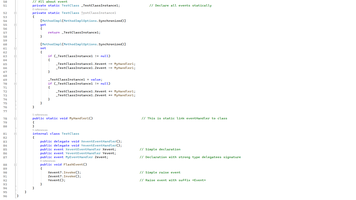
C#
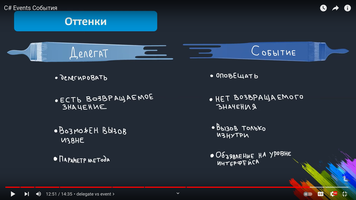
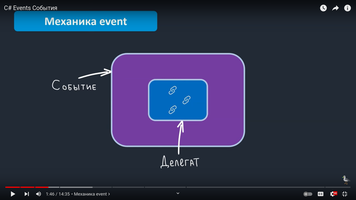
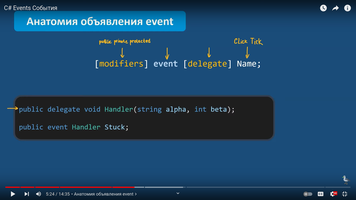
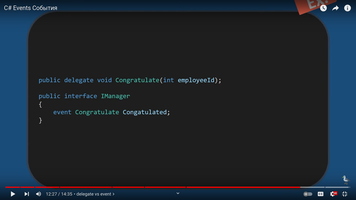
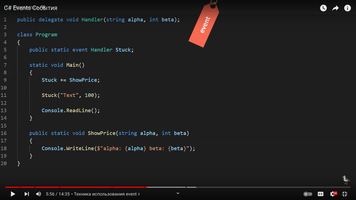
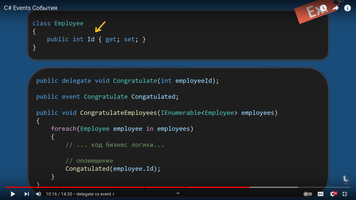
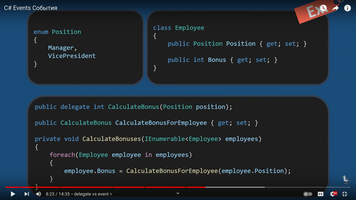
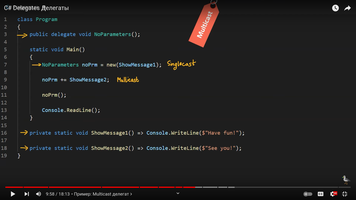
Using delegates in multithreading.
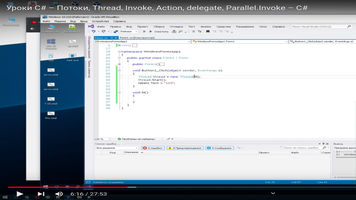
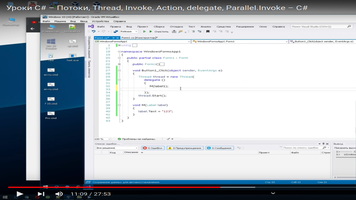
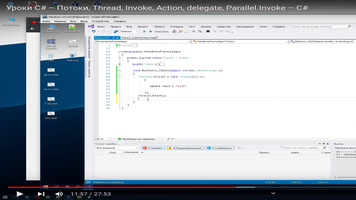
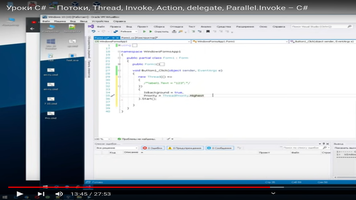
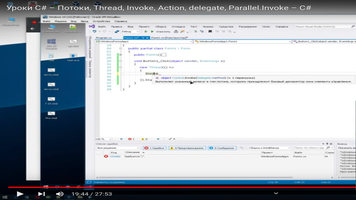
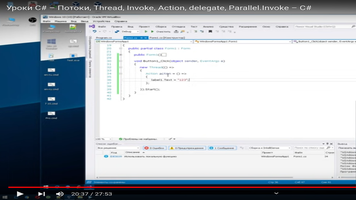
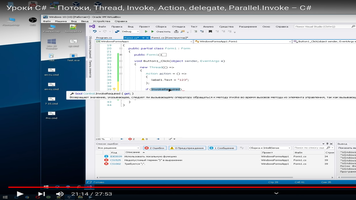
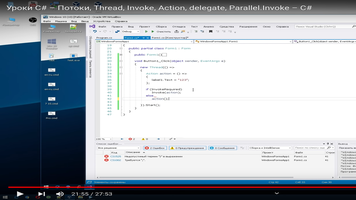
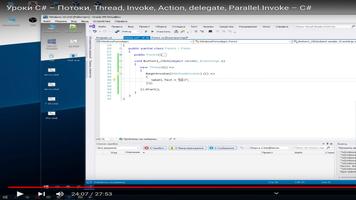
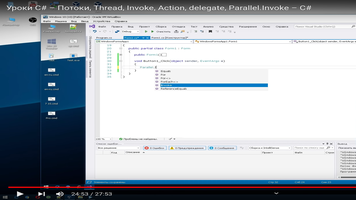
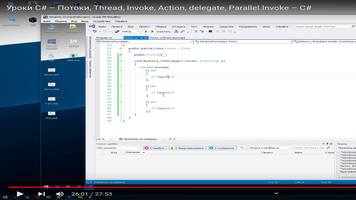
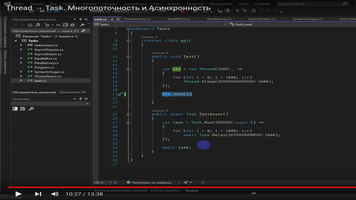
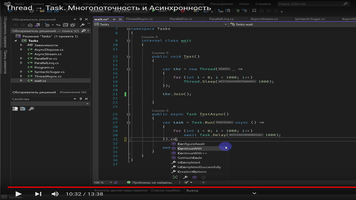
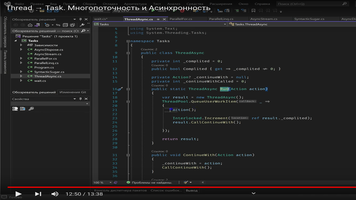
- More about events and delegates
- Events/Delegates in VB.NET (Static/Dynamic declaration, AddressOf/RaiseEvent/AddHandler/WithEvents/Handles statement,SingleCast/MultiCast/InvocationList, Invoke/BeginInvoke/DynamicInvoke/AsyncInvoke, Automatically instantiate.
- MS: Delegates and Events (VB.NET)
Comments (
)

<00>
<01>
<02>
<03>
<04>
<05>
<06>
<07>
<08>
<09>
<10>
<11>
<12>
<13>
<14>
<15>
<16>
<17>
<18>
<19>
<20>
<21>
<22>
<23>
Link to this page:
//www.vb-net.com/QuickHelp/Csharp6/Index.htm
<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |