(<< Back <<) Max Typescript Lecture (<< Back <<)
Maximilian Schwarzmüller Typescript Lecture notes.
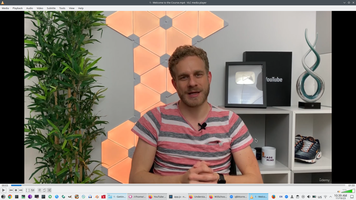
All TS future is accesible on Compilation time, on Runtime we receive just only JS We can create JS TypeOf TypeGuard just based on simple JS types, We can use JS TypeOf with Number or String, but don't use with Date, because JS DateType is missing JS Runtime not check variable type, On TS we can avoid to use type Any, any this is fallback TS intercept all possible issue in Compilation time - 1,2 + 1,2 = 2; 1,2 + 1.2 = 3.2; +'1.2' + 1.2 = 2.4; '1.2' + 1.2 = "1.21.2"; 1,2 + '1,2' = "21,2"; 1,2 + +'1.2'= 3.2; 1.2 + +'1,2'=NaN; 1.2 ++'1,2' = invalid increment/decrement operand; 1.2 || 1,2 = 2; 1.2 || '1,2' = 1.2; '1.2' || '1,2' = "1.2"; '1.2' && '1,2' = "1.2"; '1.2' && 1,2 = 2; '1.2' && 1.2 = 1.2; '1.2' && '1,2' = "1,2"; 1,2 ?? '1,2' = 2; 1.2 ?? 1.2 = 1.2; '1.2' ?? 1.2= "1.2"; +'1.2' ?? '1.2' = 1.2; '+' is opposite to ToString(), convert to Number We can use keyword 'Object' and '{}' for TS Object, Object type separate property with ';', but object with ',' Array, TS Array need type Any[] to support different type item, OR we can use Union Type String | Number Tuple declaration, Push to Tuples look strange Convention for Enum is started with UpperCase, We can start assign Enum from any value Literal type, Literal type (with Union type) Type Alias Void type, JS don't know what is Void, TS Void produced JS Undefined. Ts Undefined is not the same that TS Void TS Function type - reference to JS Function, use Function name without '()' On TS we omit type declaration, we use explicit type declaration instead implicit Definition Anonymous function and pass it as parameter, Definition Callback function as parameter, Pass Anonymous function as Callback TS Unknown is more restricted that TS Any, We can avoid Any and use Union type as possible TS Never type. We used Never usually if function finish with Throw
Class, On JS ES6 we see the same code as on TS, but on ES5 class implements as Prototypes Property, Default property value, Constructor, Methods (default is Public), Private value, This Instantiate with New and without New with {} Shortcut - declare private variables on Constructor Private constructor, Super Singleton, GetInstance Extends - Class Inheritance Static methods, Abstract class and Abstract some method - force user to implement that one method Interface look as Abstract class, but without implementation. Interface vs Custom type, Custom type is more flexible (we can use Union type), but we can share interface to many class and class can implement multiple interfaces. Interface definition can not be Public/Private (it's always public), but can be Readonly Class can implements many interface, Interface can extends one another Custom Type the same as interface. "!", and "?" - mark property and method as optional Alternate way to make parameter optional - set default value
"&" Type intersection operator, This will be the same intersection if we create two interface and Extends new interface from both of they Type Guard choice 1 (only for JS simple type) - TypeOf,W e can not use this approach to TS Types and Interface, because JS known nothing about its Type Guard choice 2 (check if property is exist) - IN Type Guard choice 3 (check if class instantiated) - InstanceOf, this way can not be used to TS Type and TS Interface, because JS know nothing about they Type Guard choice 4 - discriminated union, add implicit type as property. We working with "DOM" library on configuration, therefor we have access to any HtmlElement. First way of Type casing is cast by angular brackets <>. And we need to use "!" exclamation mark. Second way of Type casing is casting by AS (this way also working with React) Or we can delete exclamation mark with manually checking presentation Html element Indexed property - we don't need specify exactly property name, we will declare just property count and type. Interface X {[prop:string]:string]} Empty, or any property with string name."1" will be interpreted as string in this case. Also ee will declare property name with string array Function overloading One way in typecasting Result with AS Second way - create declaration for function overloading, function definition only without cali-brackets {}, Ts engine will be understand this overloading. Optional chaining operator - "?" We can check property one-by-one on this way (if job is undefined second part after "&&" never run) Or simple use optional chaining "||" Nullish coalescing operator - is JS future, but on JS this is "??" "''", "undefined", "null" look as False and variable with "||" assign with "Default" value. If we want to use exactly "null" or "undefined" (but not "''" empty) - we need to use "??" Nullish coalescing operator
If we use union type or complex types or less precise type we have a number of way to clarify type and use more precise type - this is type narrowing. 1. JS Typeof Guard 2. Truthiness guard (check by IF). These values {0, NaN, "" (the empty string), 0n (the bigint version of zero), null, undefined} - always get False on IF checking. Other get True on IF checking. 3. Equality narrowing. Comparing without transformation to one type (===, !==) or with transformation to one type (==, !=). 4. IN narrowing - check if object of prototype has property 5. InstanceOf narrowing - check prototype chain, and object created with New 6. Type predicates AS - TypeScript will narrow that variable to that specific type if the original type is compatible 7. Discriminated unions - store literal as one of property 8. Using Never type to receive exception if type is wrong 9. Also type can be checking by Assertion Functions, NodeJs generate AssertionError if checking by Assert function generate exception, JS has only Console.Assert function
Generic Array (with "<" ">" angular brackets) and Predefined Array of string Define empty array with square brackets "[" "]", we can initialize in this way any kind of array. But correct definition allow us working with type safe Array<string> We have no information what data return JS Promise, But we can define return datatype Promise<string> Object.Assign is JS method what allow copy all property from Source to Target object, But TS not sure that property exists on target object, We can type cast of result object with "AS" Or create Generic object Result have intersection of type U "&" T, because for this function input parameters "T & U" explicitly setup output type as interception "T & U" Constraint - limitation or restriction, we want to protect from call this function with simple value, because this function working only with Objects. Extends keyword help us. In this case function return tuple (value with description), Length property must bu exist, Also we can specify implicit output type KeyOf Constraint - this is simple JS function, but this is not working on TS, Because TS can not guarantee that key exist in object U extends KeyOf T - guarantee that U property exist in T object Generic utility types - Partial and Readonly - fix all object property of make it optional (locked ot open to modification) Partial mean that all property is optional, therefore we can create empty object firstly and than step-by-step adding property. And finally casting to clear type with "AS". How to make array locked? Readonly forbid changing any property Union types vs Generic types. Union types allow story mixed value, buy Generic types allow stre only one object type. Generic type allow lock one certain type.
Namespaces, Export, Export Default, /// reference, Html 'Script' tag with defer (async) (Type=amd on compiler options) with bundle all JS to one, Html 'Script' Type=Module (ES6 on compiler options), Import variation (omit {}, alias 'as', *)
install @types/xxx --save-dev, Lodash, Validators, using class-transformer and import 'reflect-metadata', declare external variables from Html level (setting)
TS development environment (Lite-server, tsc -w) default TS library var/const/let arrow function syntax (with variation, omit () and {}) '...' spread operator (4 type of usage) Destructuring assignment
@C @D class Person {} the same as C(D(class Person())). Allow decorator in TS config. Constructor:Function - this is decorator factory, Function return Function - in this case we can use Decorator as Function. Pass parameters to Decorator. Bind DIV ID from HTML template to Decorator function. "_" underscore parameters. Changing constructor type to Any allow to create new constructor. Use "!" exclamation mark to guarantee as HTML element present. By Decorator Angular framework to pass HTML template to code. Multiple decorator. Two types of decorators - Logger factory and Template factory. Decorator to Method Accessor, Class, Property, Method Parameter - only interface has difference. Common decorator interface (with Symbol). Order of multiple Decorator. Any Decorator working when JS engine faced with class, not when class instantiated. Class extended Constructor and we will used Super (MyBase). New Constructor function will replace original constructor function with new extra logic. Generic '<T extends { new (... args: any []): () } >' allow accept any parameters. Anonymous class with Anonymous method. Generic - decorated must have property Text. If we don't instantiate class, Template decorator don't working (because document not exists and don't rendered), When we call Super we save original function, and we save original class but we replace original class with new extra logic. Decorator can return anything, but TS will ignore what decorator return. We can change options of Method of Accessor, for example Configurable, Enumerable. JS Bind() and AutoBound decorator. This is different on EventListener and Class. Create decorator Antobind() what change context from Button.EventHandler to Class exemplar. Parameters of Method decorator, (1) Target is Prototype because we use Instance method, (2) MethodName or PropertyName, (3) ProertyDescriptor, Validation decorator. Text to Digital by '+. TS validations with decorators.
2. TS basic
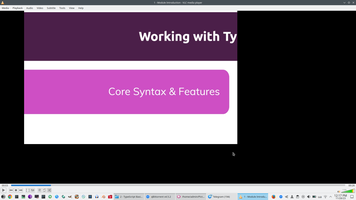
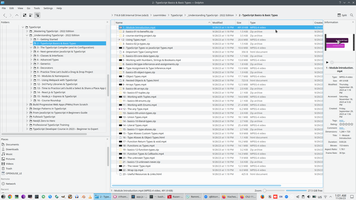
1 - Module Introduction.mp4 2 - Using Types.mp4 3 - TypeScript Types vs JavaScript Types.mp4 5 - Working with Numbers, Strings & Booleans.mp4 6 - Type Assignment & Type Inference.mp4 7 - Object Types.mp4 9 - Arrays Types.mp4 10 - Working with Tuples.mp4 11 - Working with Enums.mp4 12 - The any Type.mp4 13 - Union Types.mp4 14 - Literal Types.mp4 15 - Type Aliases Custom Types.mp4 17 - Function Return Types & void.mp4 18 - Functions as Types.mp4 19 - Function Types & Callbacks.mp4 20 - The unknown Type.mp4 21 - The never Type.mp4 22 - Wrap Up.mp4
Types supported on TS based on JS, all TS future is accesible on Compilation time, on Runtime we receive just only JS, we can create JS TypeOf TypeGuard just based on simple JS types
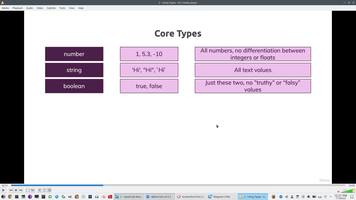
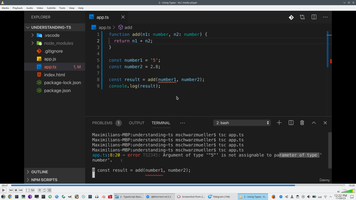
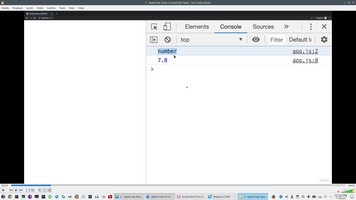
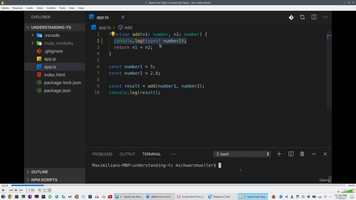
We can use JS TypeOf with Number or String, but don't use with Date, because JS DateType is missing
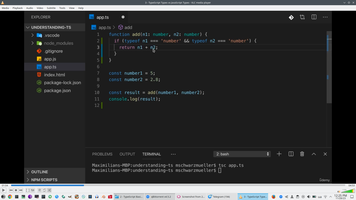
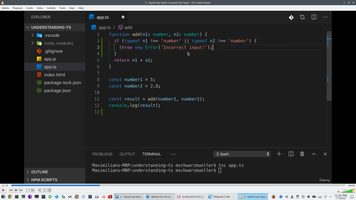
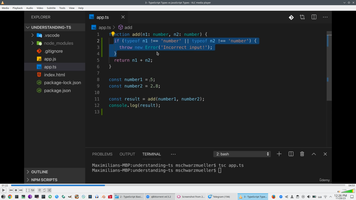
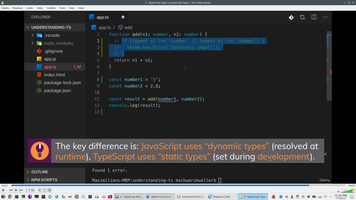
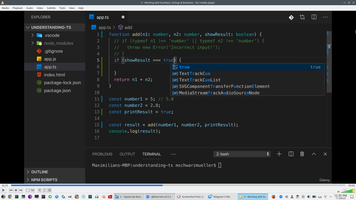
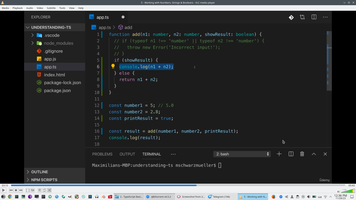
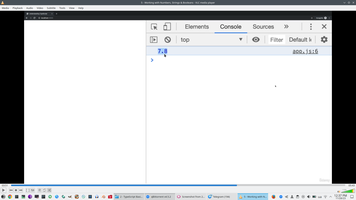
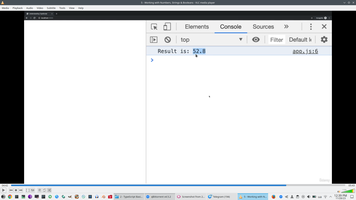
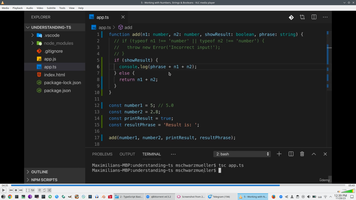
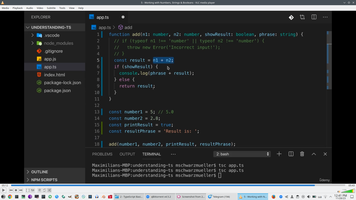
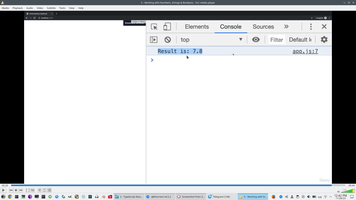
All these marvels ('7.8' / '53.8') happens because JS Runtime not check variable type.
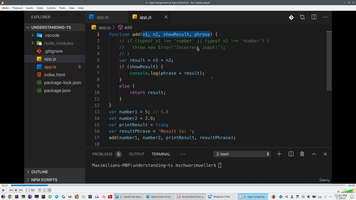
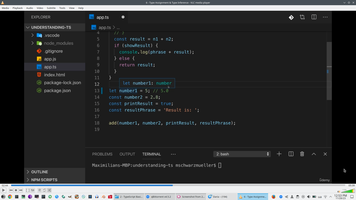
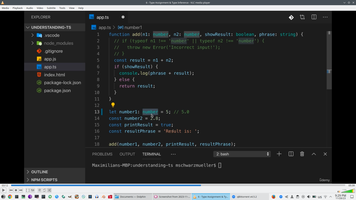
And TS intercept all possible issue in Compilation time
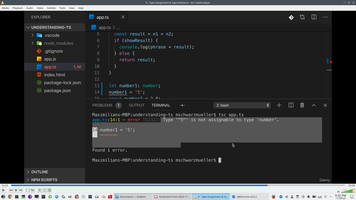
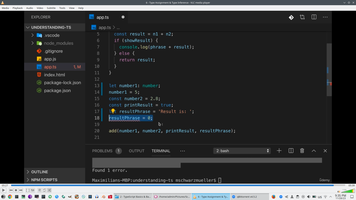
Object
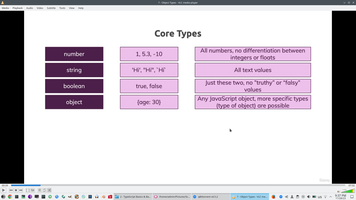
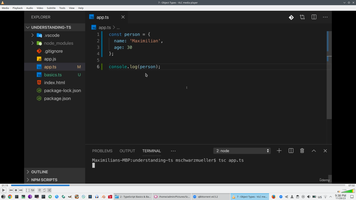
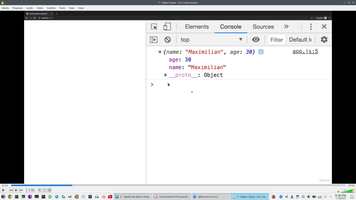
We can use keyword 'Object' and '{}' for TS Object
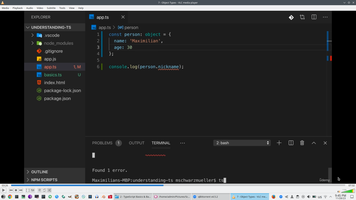
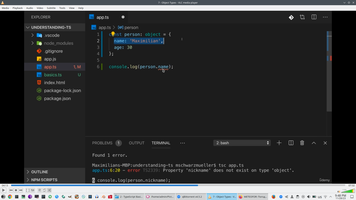
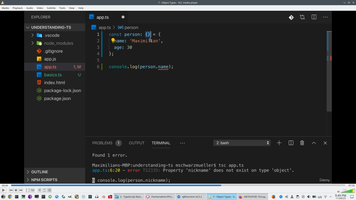
Object type separate property with ';', but object with ','
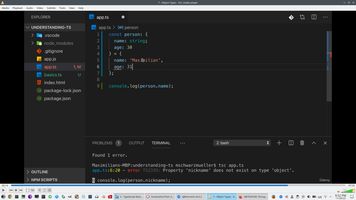
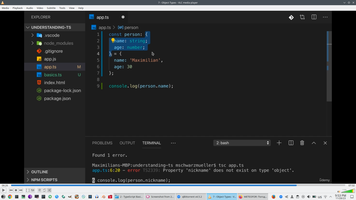
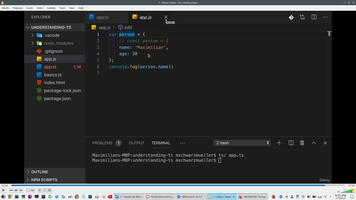
Array
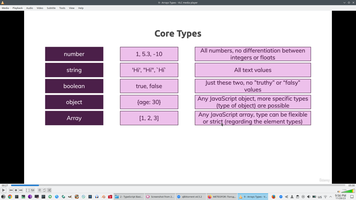
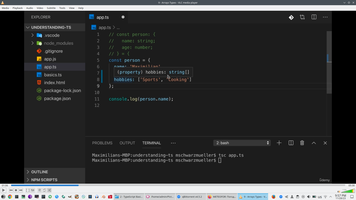
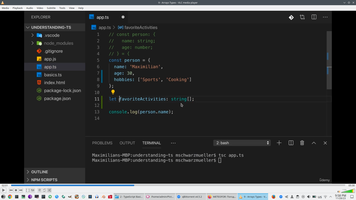
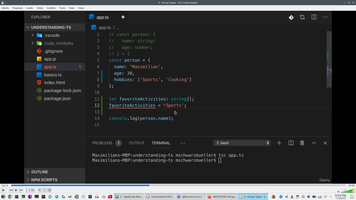
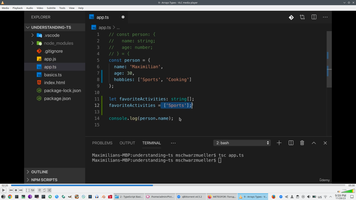
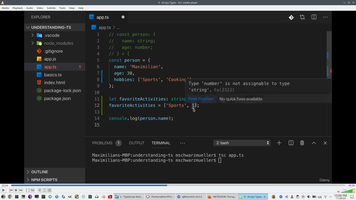
TS Array need type Any[] to support different type item
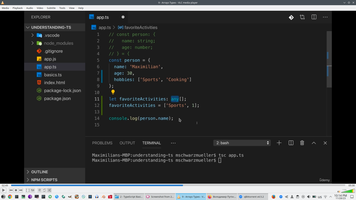
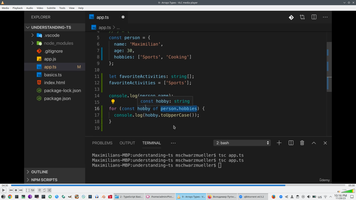
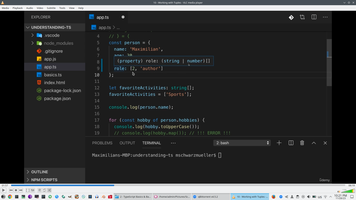
OR we can use Union Type String | Number
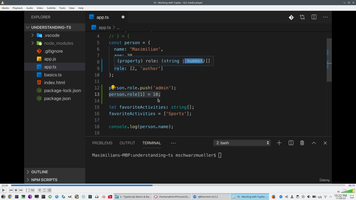
Tuple declaration
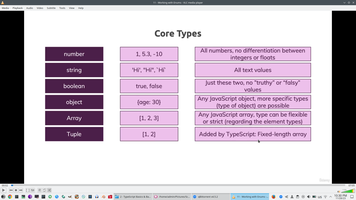
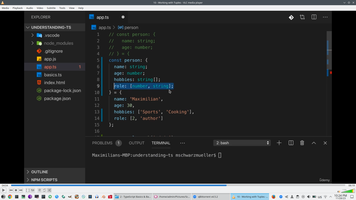
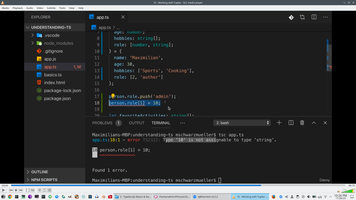
Push to Tuples look strange
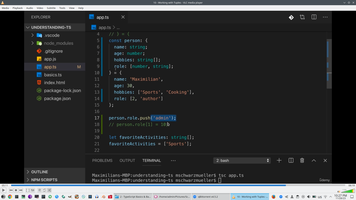
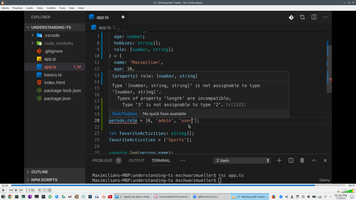
Convention for Enum is started with UpperCase
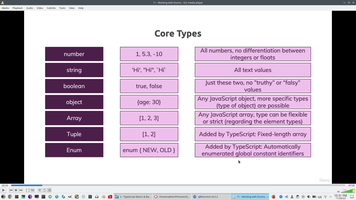
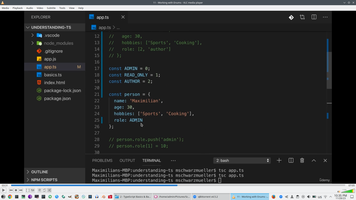
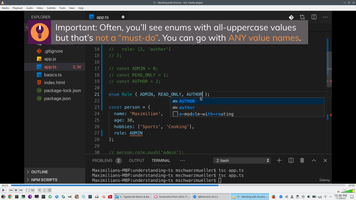
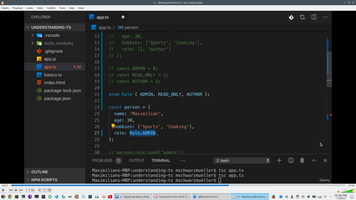
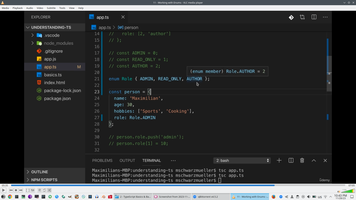
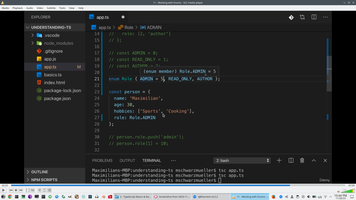
We can start assign Enum from any value
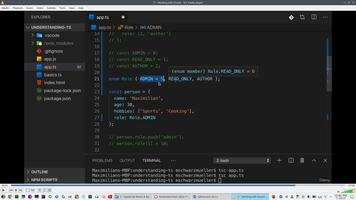
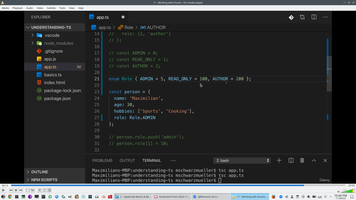
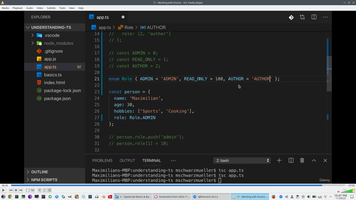
We can avoid to use type Any, any this is fallback
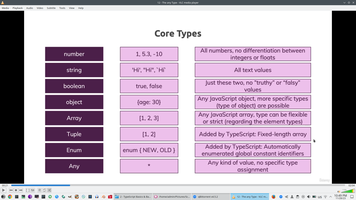
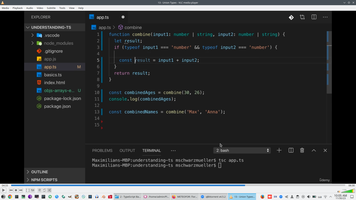
Literal type
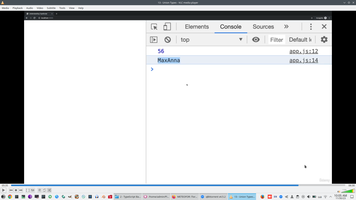
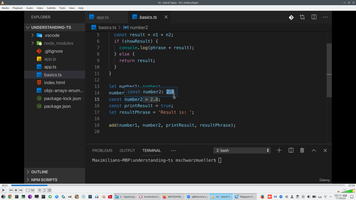
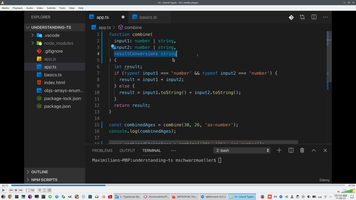
'+' is opposite to ToString(), convert to Number
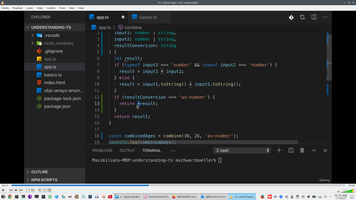
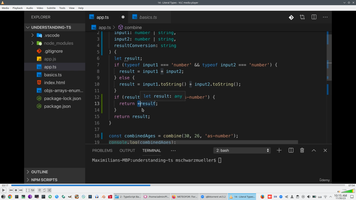
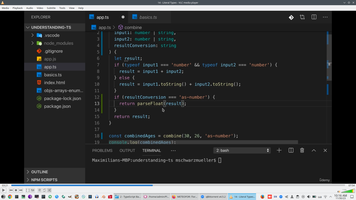
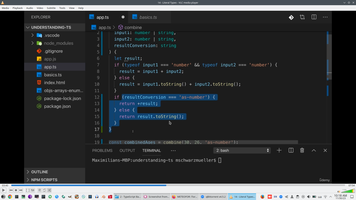
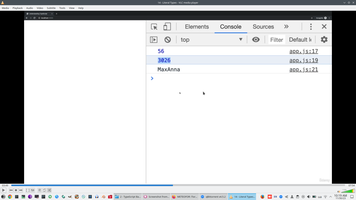
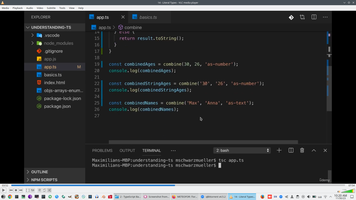
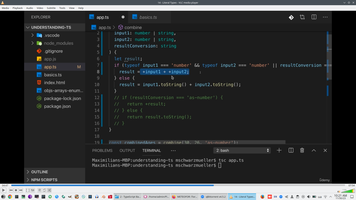
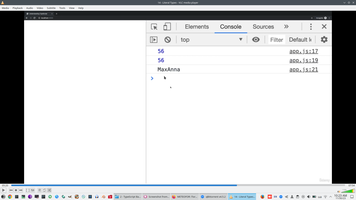
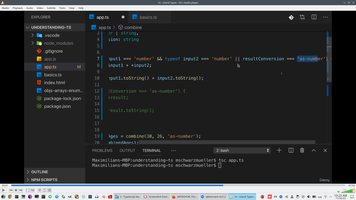
This is clear Literal type (on Union type)
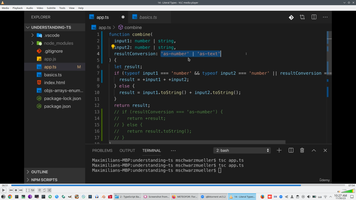
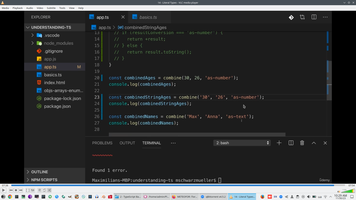
Type Alias (in this case empty, this idiotic idea because currently this is Number only).
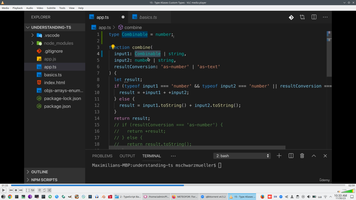
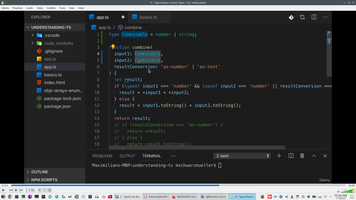
Literal type, pay attention that JS don't know anything about TS Types
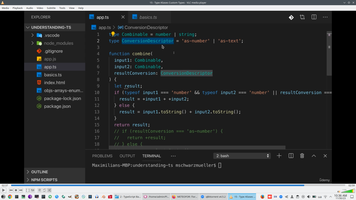
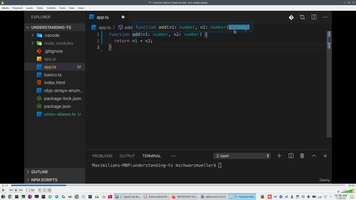
Void type, JS don't know what is Void, TS Void produced JS Undefined.
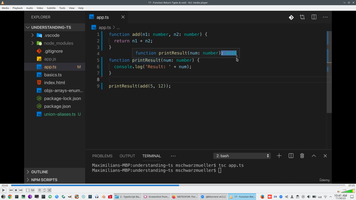
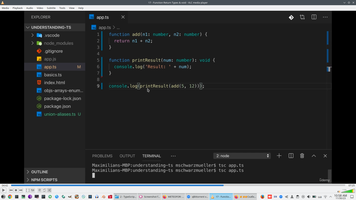
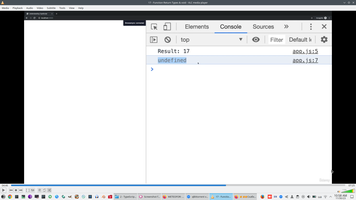
Ts Undefined is not the same that TS Void
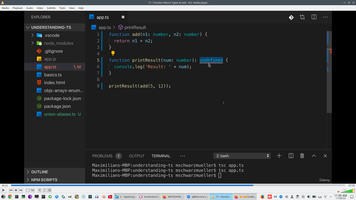
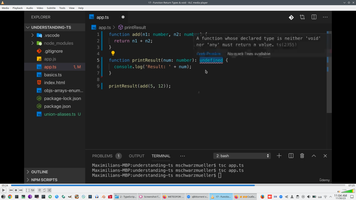
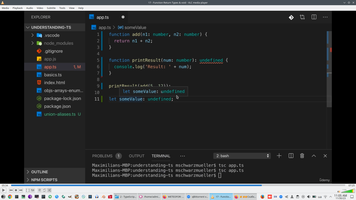
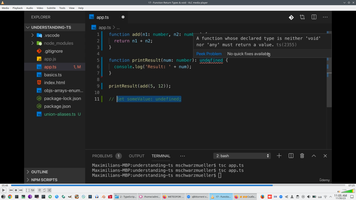
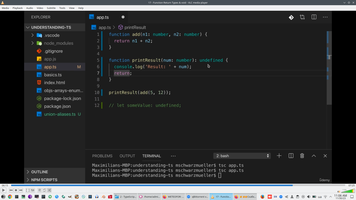
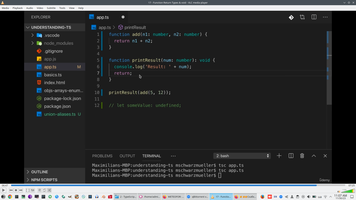
TS Function type - reference to JS Function, use Function name without '()'
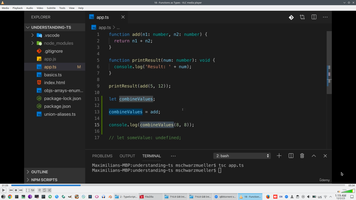
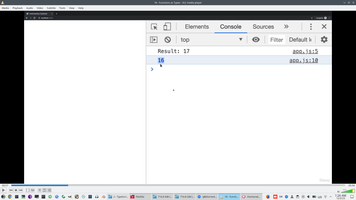
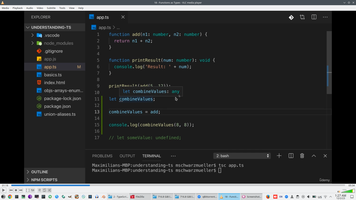
This is normal TS Code with normal compilation, but JS runtime give error, because we omit type declaration, we use explicit type declaration instead implicit and value 5 has no the same interface as JS function
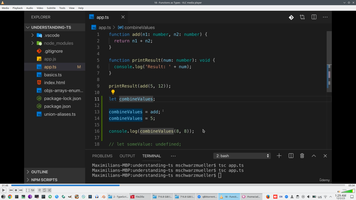
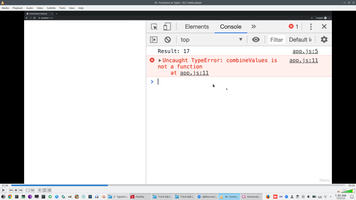
We can avoid this error with explicit declaration pointer to function
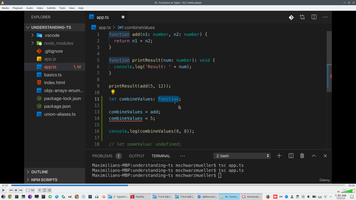
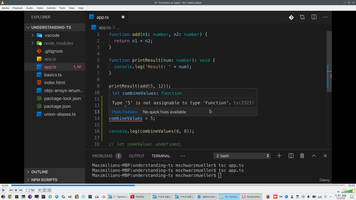
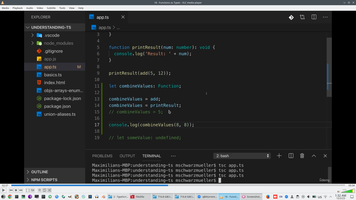
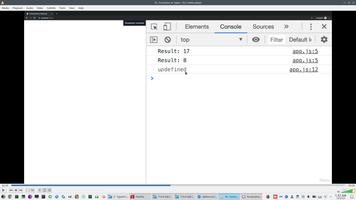
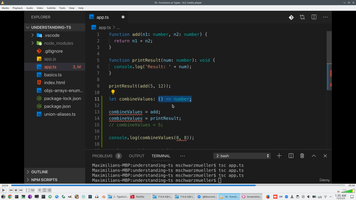
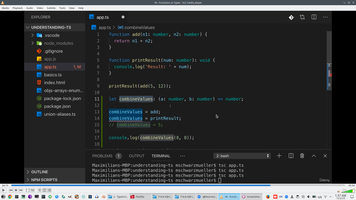
Definition Anonymous function and pass it as parameter
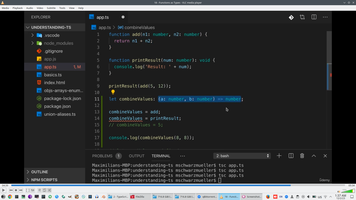
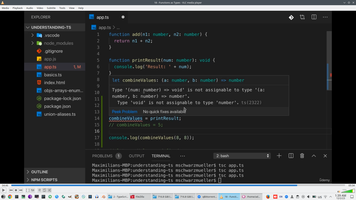
Definition Callback function as parameter
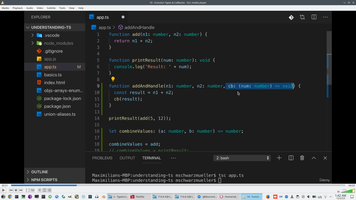
Pass Anonymous function as Callback
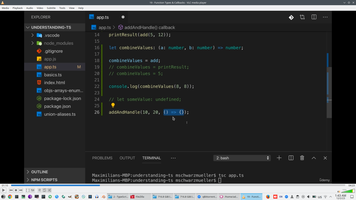
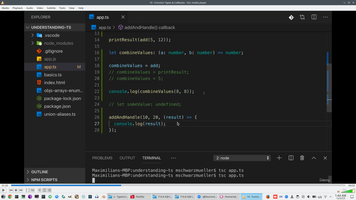
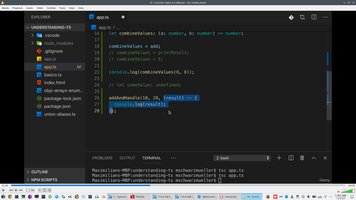
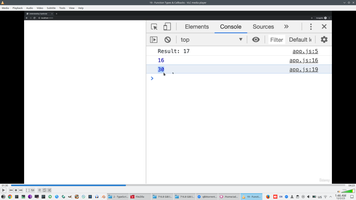
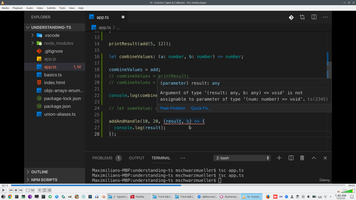
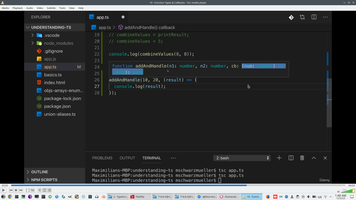
TS Unknown is more restricted that TS Any
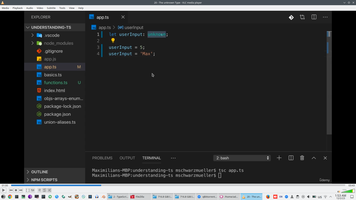
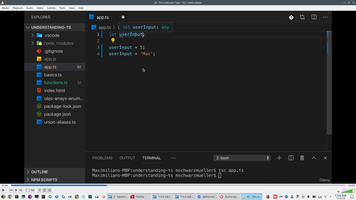
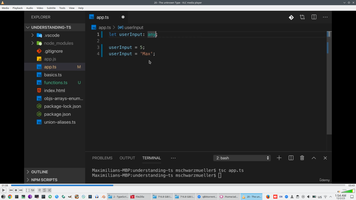
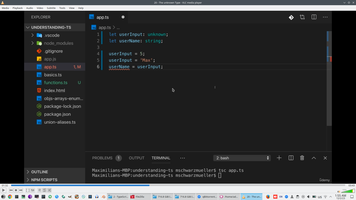
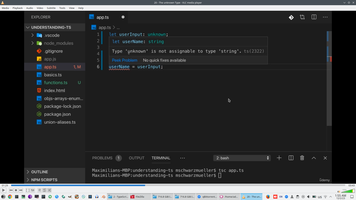
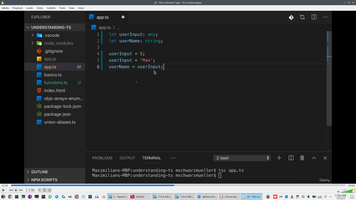
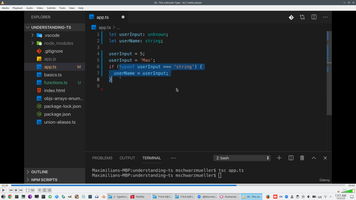
We can avoid Any and use Union type as possible
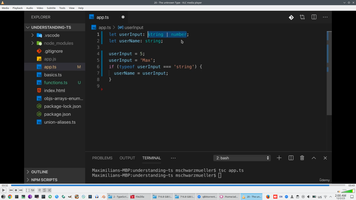
This function return TS Never type. We used Never usually if function finish with Throw
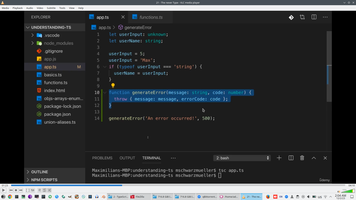
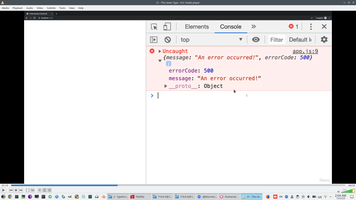
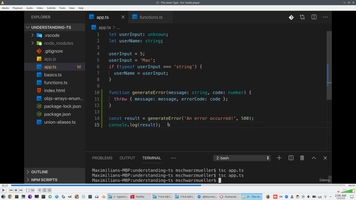
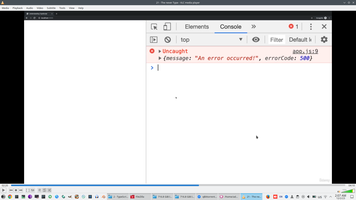
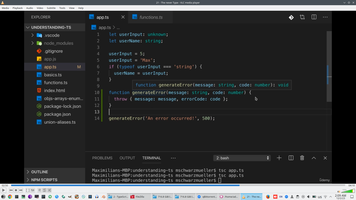
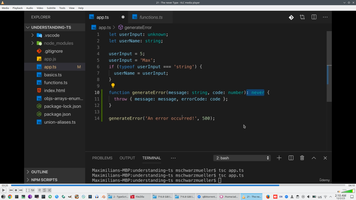
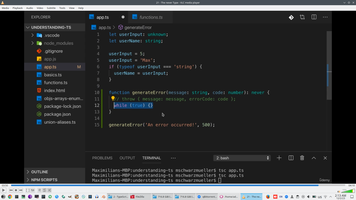
5. OOP
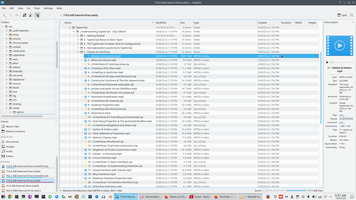
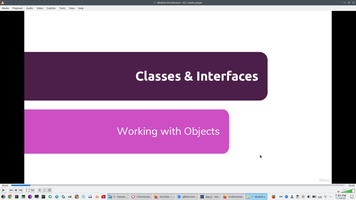
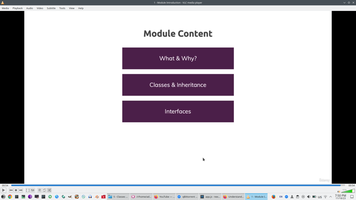
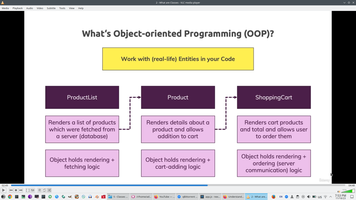
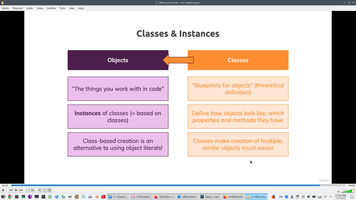
1 - Module Introduction.mp4 2 - What are Classes.mp4 3 - Creating a First Class.mp4 4 - Compiling to JavaScript.mp4 5 - Constructor Functions & The this Keyword.mp4 6 - private and public Access Modifiers.mp4 7 - Shorthand Initialization.mp4 8 - readonly Properties.mp4 9 - Inheritance.mp4 10 - Overriding Properties & The protected Modifier.mp4 11 - Getters & Setters.mp4 12 - Static Methods & Properties.mp4 13 - Abstract Classes.mp4 14 - Singletons & Private Constructors.mp4 15 - Classes - A Summary.mp4 16 - A First Interface.mp4 17 - Using Interfaces with Classes.mp4 18 - Why Interfaces.mp4 19 - Readonly Interface Properties.mp4 20 - Extending Interfaces.mp4 21 - Interfaces as Function Types.mp4 22 - Optional Parameters & Properties.mp4 23 - Compiling Interfaces to JavaScript.mp4 24 - Wrap Up.mp4
Class
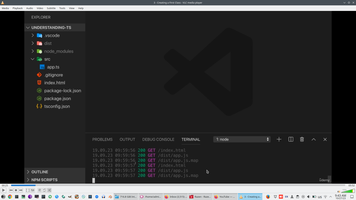
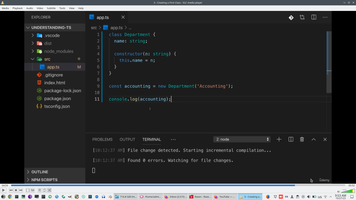
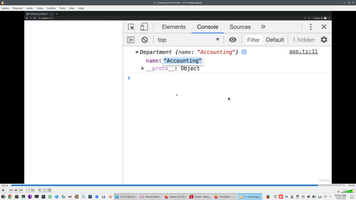
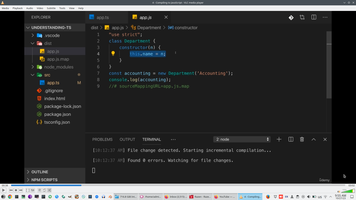
On JS ES6 we see the same code as on TS
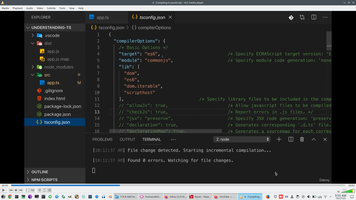
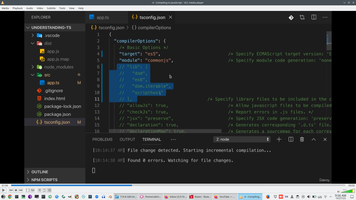
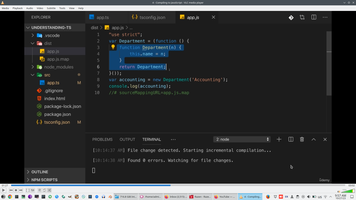
Example class compilation to JS ES5
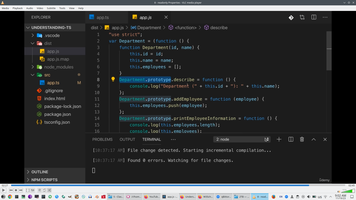
Property, Default property value, Constructor, Methods (default is Public), Private value, This
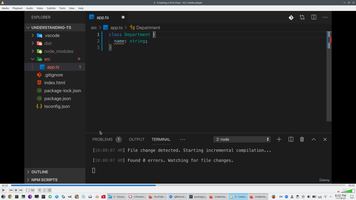
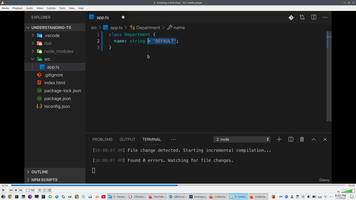
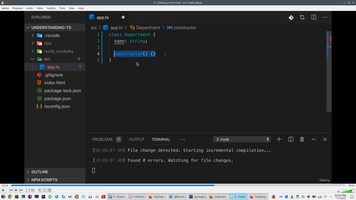
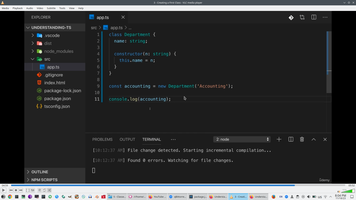
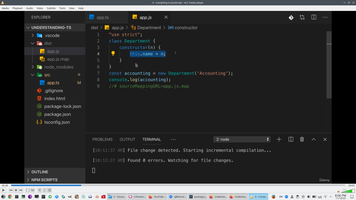
Instantiate with New and without New
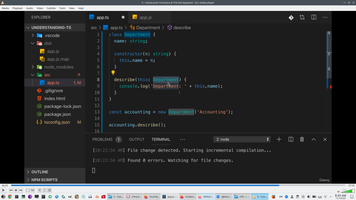
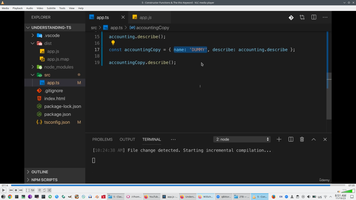
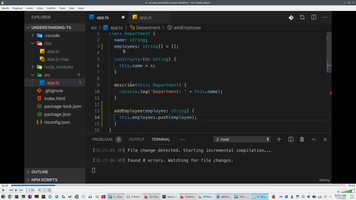
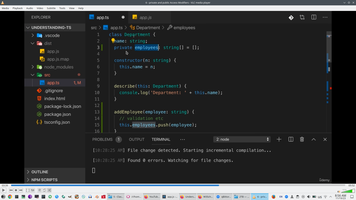
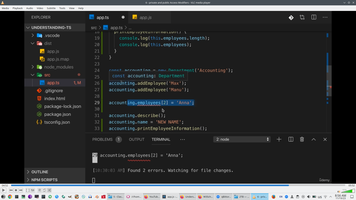
Shortcut - declare private variables on Constructor
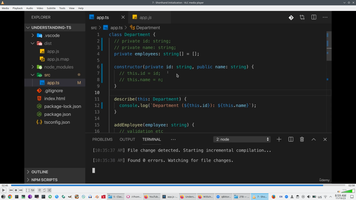
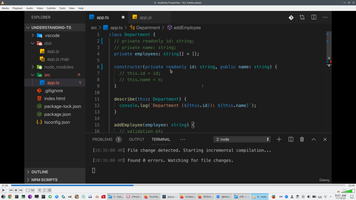
Private constructor, Super
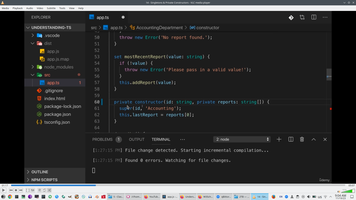
Singleton, GetInstance
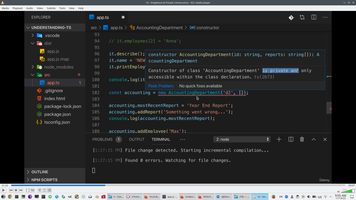
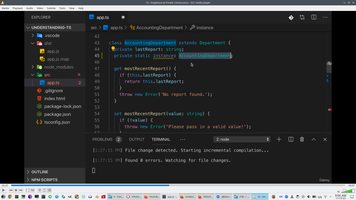
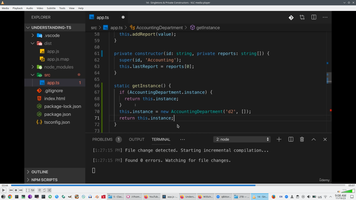
Extends - Class Inheritance
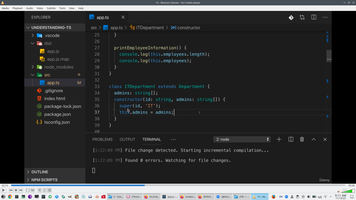
Static methods, Abstract class and Abstract some method - force user to implement that one method
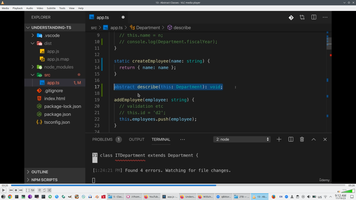
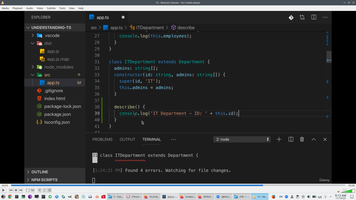
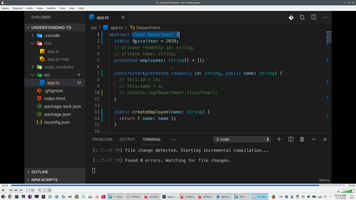
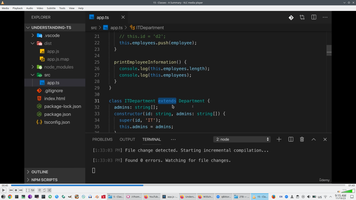
Interfaces not exist on JS level, this TS abstraction level, we can use Interface as type of object, Interface use ";" between property, but class "," between property. Interface look as Abstract class, but without implementation.
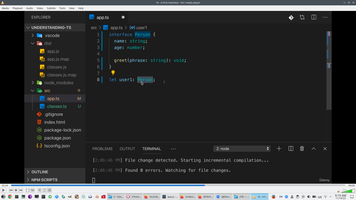
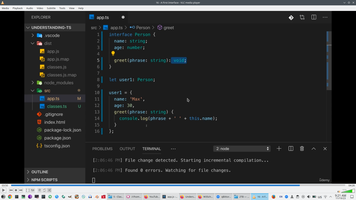
Interface vs Custom type, Custom type is more flexible (we can use Union type), but we can share interface to many class and class can implement multiple interfaces.
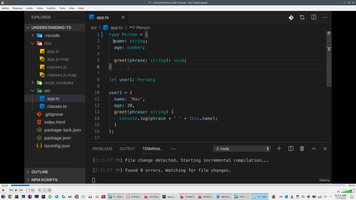
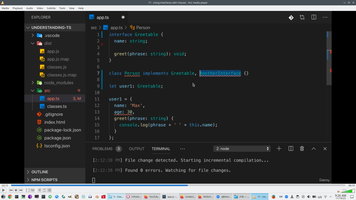
Interface definition can not be Public/Private (it's always public), but can be Readonly
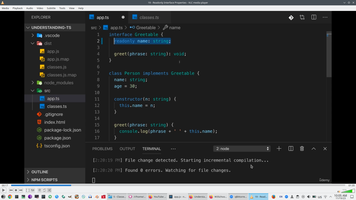
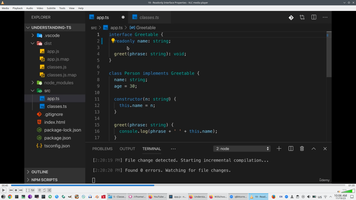
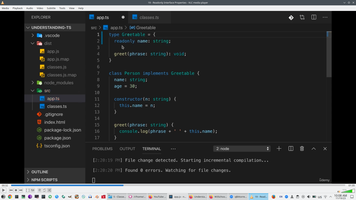
Class can implements many interface, Interface can extends one another
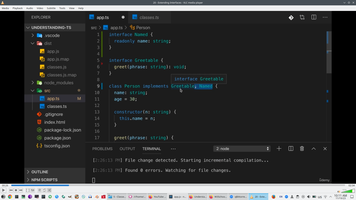
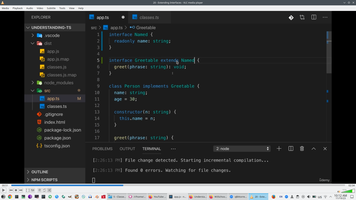
Custom Type the same as interface.
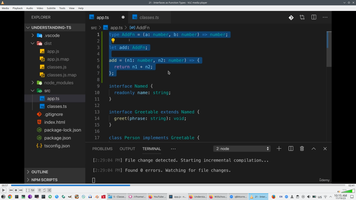
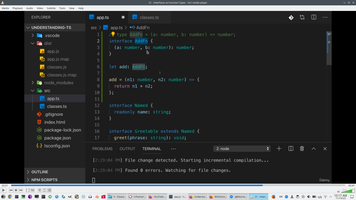
"!", and "?" - mark property and method as optional
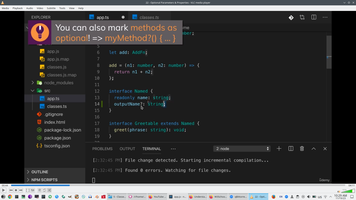
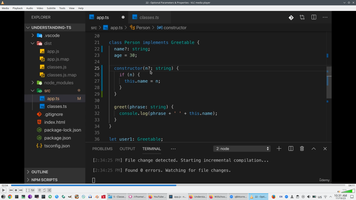
Alternate way to make parameter optional - set default value
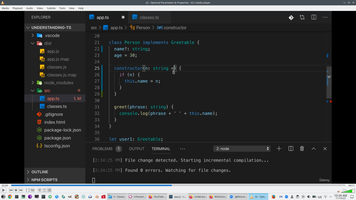
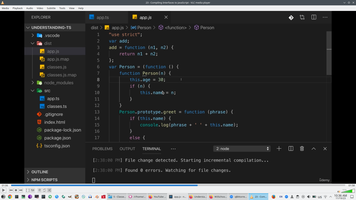
6. Types
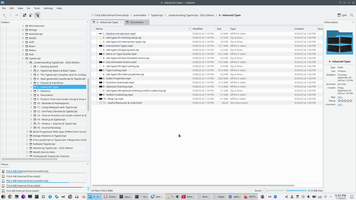
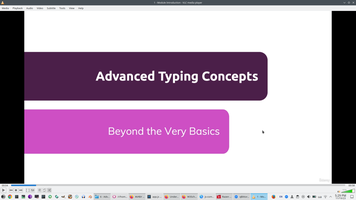
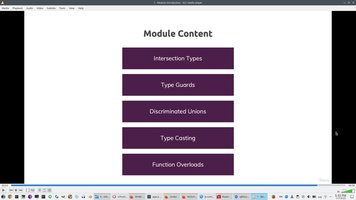
1 - Module Introduction.mp4 2 - Intersection Types.mp4 3 - More on Type Guards.mp4 4 - Discriminated Unions.mp4 5 - Type Casting.mp4 6 - Index Properties.mp4 7 - Function Overloads.mp4 8 - Optional Chaining.mp4
"&" Type intersection operator
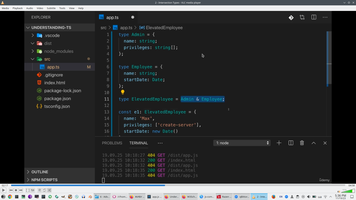
This will be the same intersection if we create two interface and Extends new interface from both of they
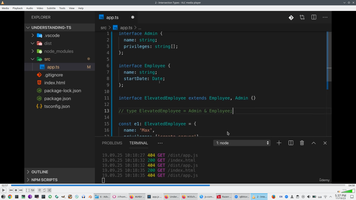
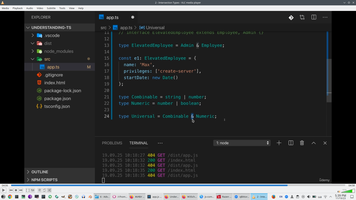
Type Guard choice 1 (only for JS simple type) - TypeOf
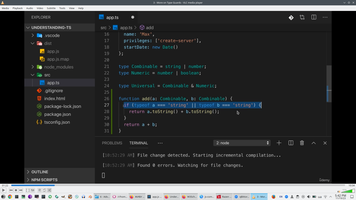
We can not use this approach to TS Types and Interface, because JS known nothing about its
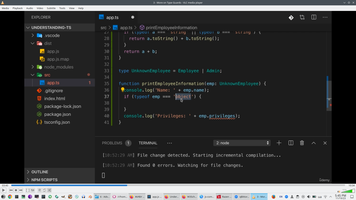
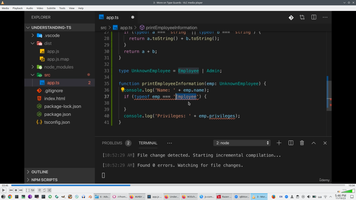
Type Guard choice 2 (check if property is exist) - IN
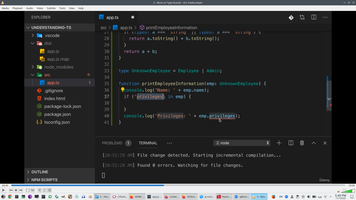
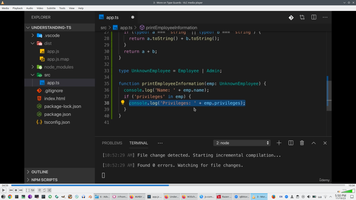
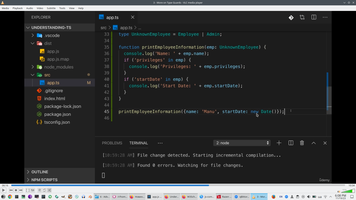
Type Guard choice 3 (check if class instantiated) - InstanceOf, this way can not be used to TS Type and TS Interface, because JS know nothing about they
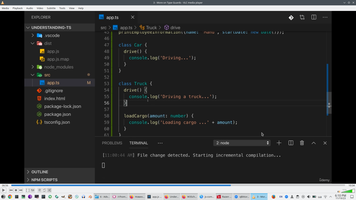
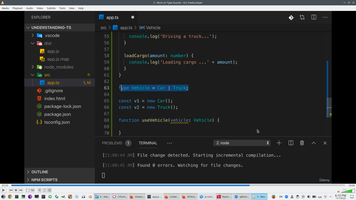
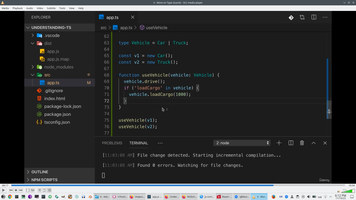
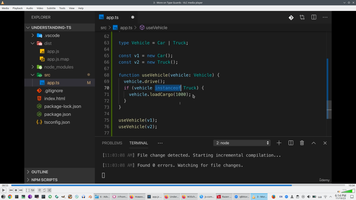
Type Guard choice 4 - discriminated union, add implicit type as property.
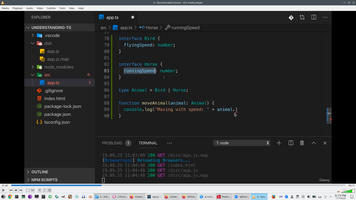
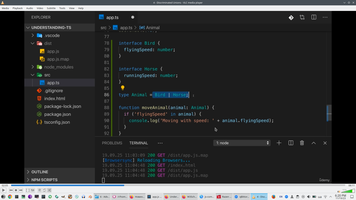
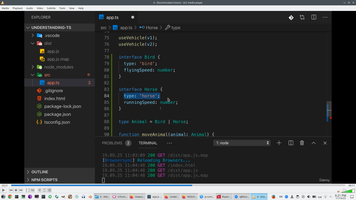
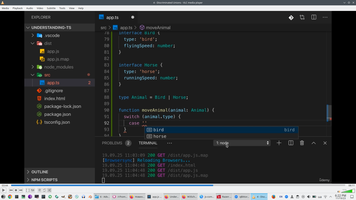
TS understanding than RunningSpead not exist on type Bird
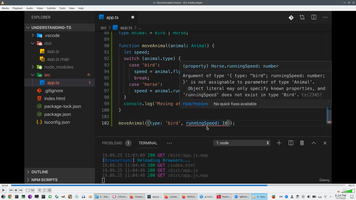
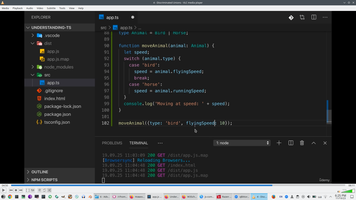
If we select simple Paragraph element we receive HTMLParagraphElement, by if we select Html by ID we will receive only common HtmlElement, and HtmlInput will has no Value.
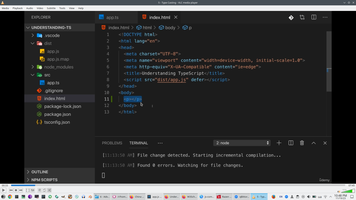
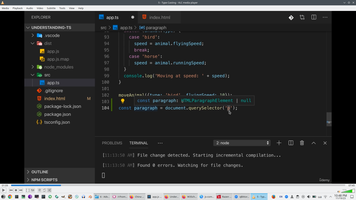
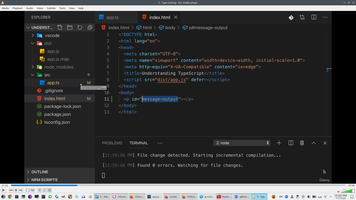
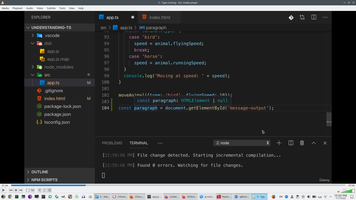
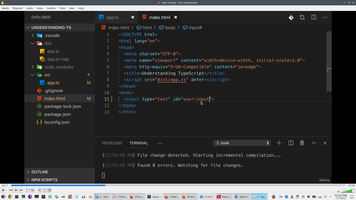
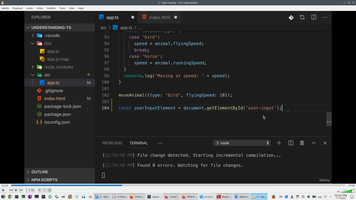
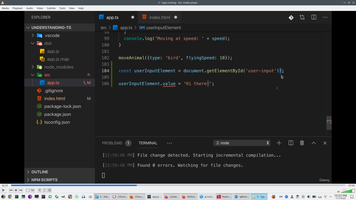
We working with "DOM" library on configuration, therefore we have access to any HtmlElement.
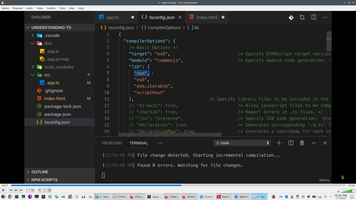
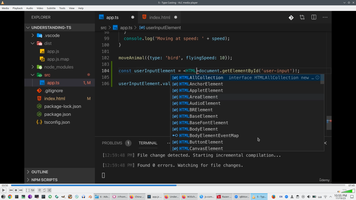
First way of Type casing is cast by angular brackets "< >". And we need to use "!" exclamation mark.
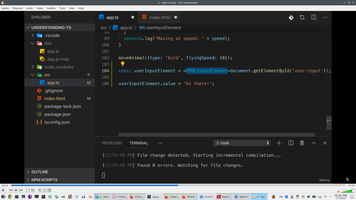
second way of Type casing is casting by AS (this way also working with React)
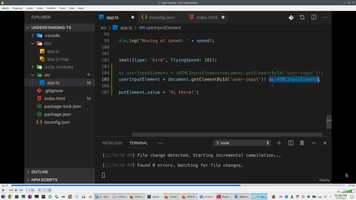
This is way to delete exclamation mark
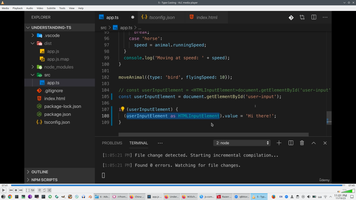
Indexed property - we don't need specify exactly property name, we will declare just property count and type.
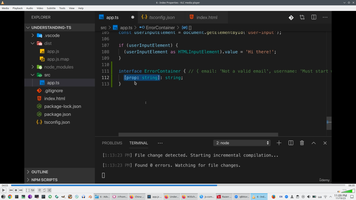
Empty, or any property with string name."1" will be interpreted as string in this case.
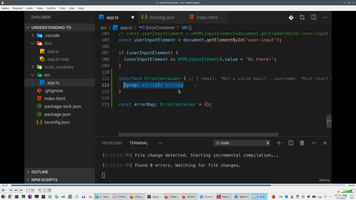
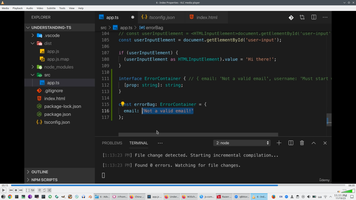
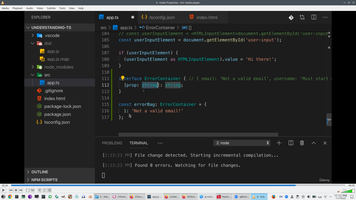
Another case - we can declare property with name as Number
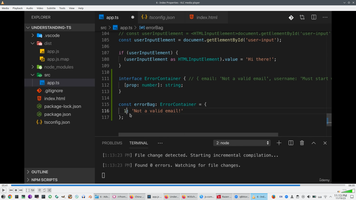
We will declare property name with string array
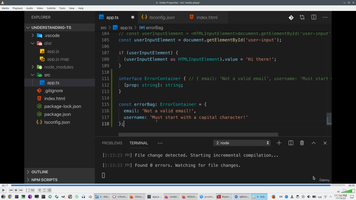
Function overloading - in this case even parameters is Number result is Combinable.
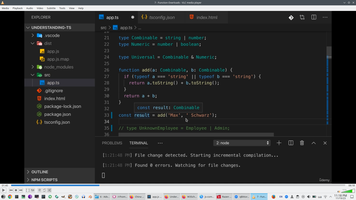
One way in typecasting Result with AS
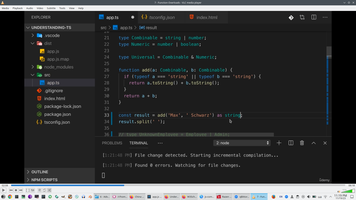
Second way - create declaration for function overloading, function definition only without cali-brackets {}, Ts engine will be understand this overloading.
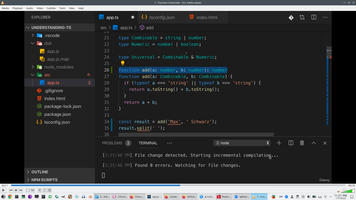
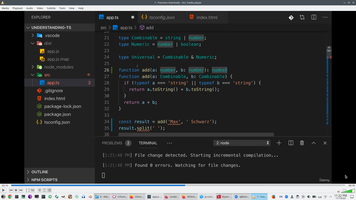
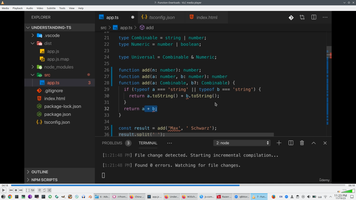
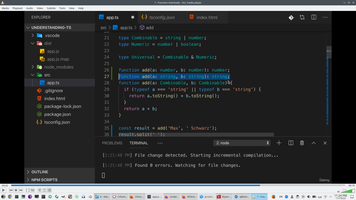
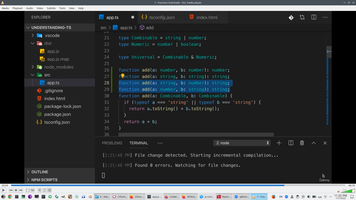
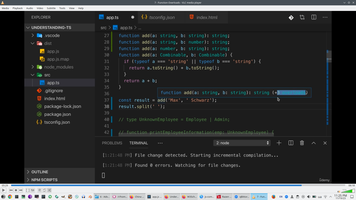
Optional chaining operator - "?"
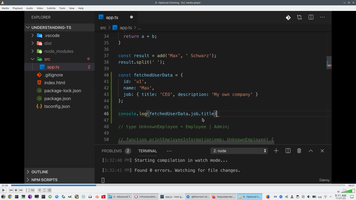
We can check property one-by-one on this way (if job is undefined second part after "&&" never run)
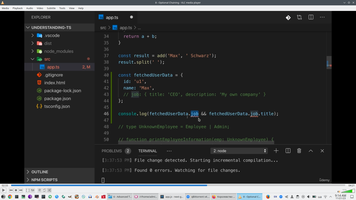
Or simple use optional chaining
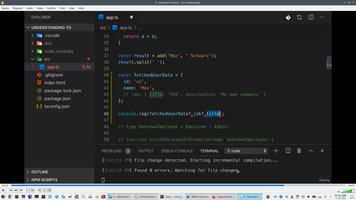
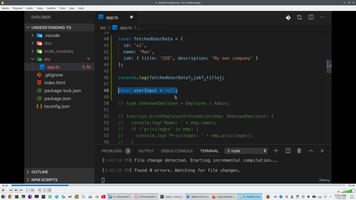
"||" Nullish coalescing operator - is JS future, but on JS this is "??" operator https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Nullish_coalescing
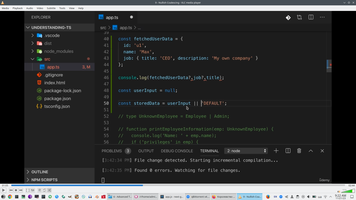
"''", "undefined", "null" look as False and variable with "||" assign with "Default" value.
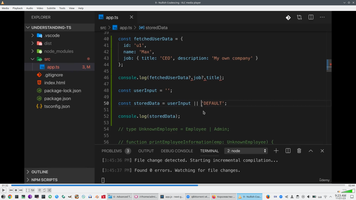
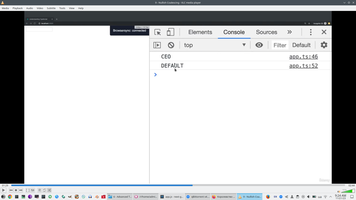
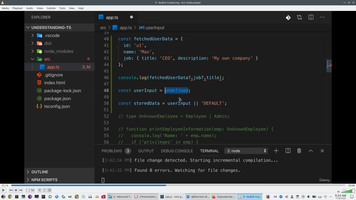
If we want to use exactly "null" or "undefined" (but not "''" empty) - we need to use "??" Nullish coalescing operator
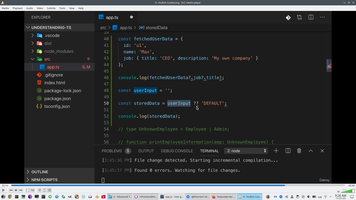
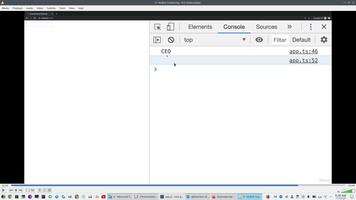
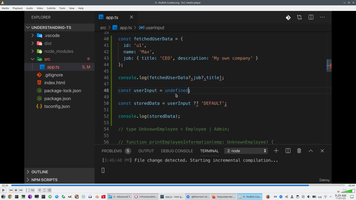
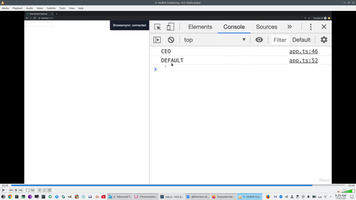
Type Narrowing - https://www.typescriptlang.org/docs/handbook/2/narrowing.html
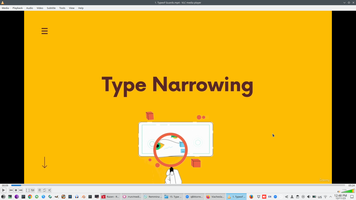
1. Typeof Guards.mp4 2. Truthiness Guards.mp4 3. Equality Narrowing.mp4 4. Narrowing With The In Operator.mp4 5. Instanceof Narrowing.mp4 6. Working With Type Predicates.mp4 7. Discriminated Unions.mp4 8. Exhaustiveness Checks With Never.mp4
If we use union type or complex types or less precise type we have a number of way to clarify type and use more precise type - this is type narrowing.
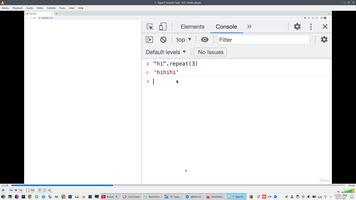
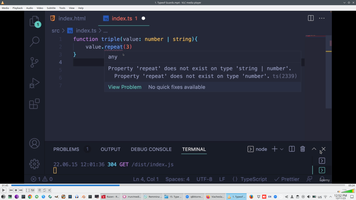
1. JS Typeof Guard
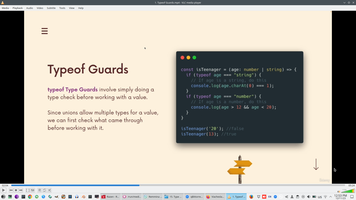
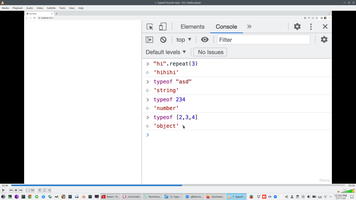
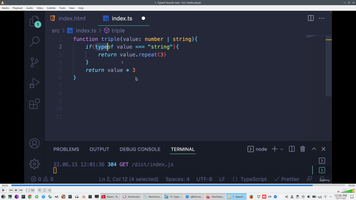
2. Truthiness guard (check by IF). These values {0, NaN, "" (the empty string), 0n (the bigint version of zero), null, undefined} - always get False on IF checking. Other get True on IF checking.
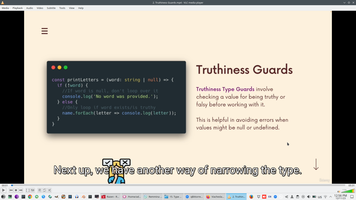
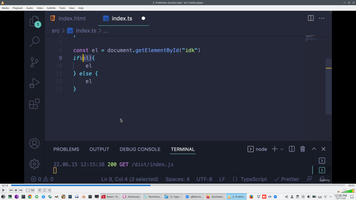
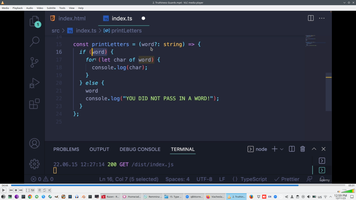
3. Equality narrowing. Comparing without transformation to one type (===, !==) or with transformation to one type (==, !=).
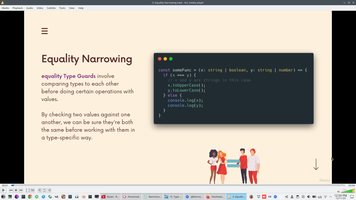
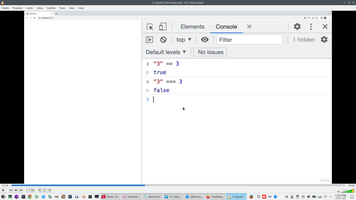
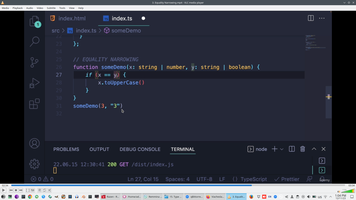
4. IN narrowing - check if object of prototype has property
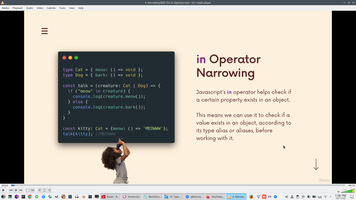
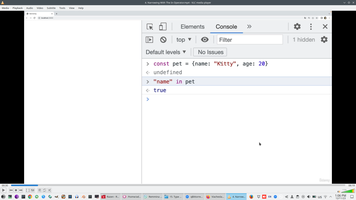
5. InstanceOf narrowing - check prototype chain, and object created with New
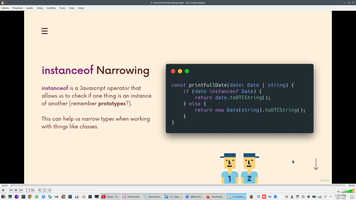
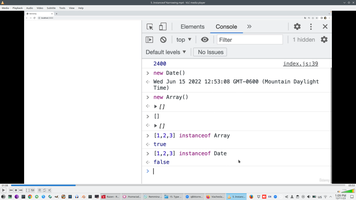
6. Type predicates AS - TypeScript will narrow that variable to that specific type if the original type is compatible
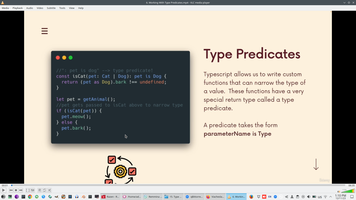
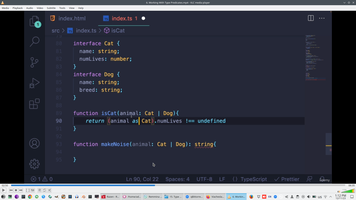
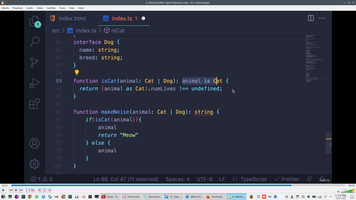
7. Discriminated unions - store literal as one of property
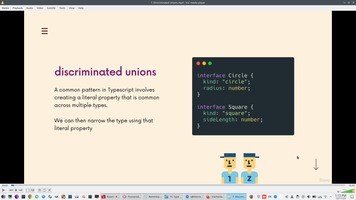
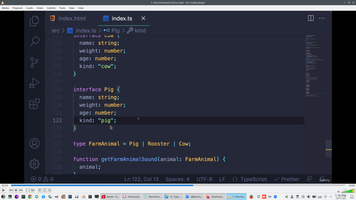
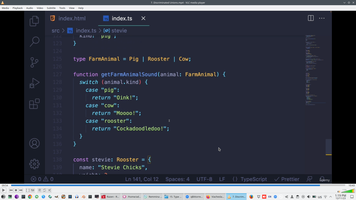
8. Using Never type to receive exception if type is wrong
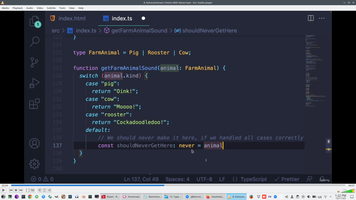
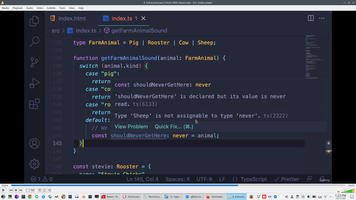
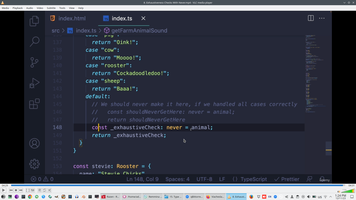
9. Also type can be checking by Assertion Functions, NodeJs generate AssertionError if checking by Assert function generate exception, JS has only Console.Assert function
7. Generics
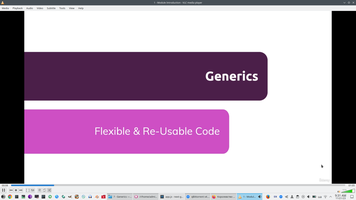
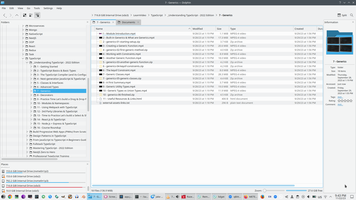
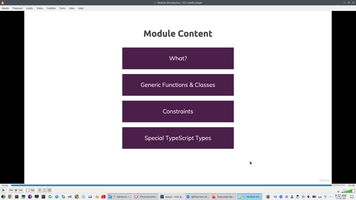
1 - Module Introduction.mp4 2 - Built-in Generics & What are Generics.mp4 3 - Creating a Generic Function.mp4 4 - Working with Constraints.mp4 5 - Another Generic Function.mp4 6 - The keyof Constraint.mp4 7 - Generic Classes.mp4 8 - A First Summary.mp4 9 - Generic Utility Types.mp4 10 - Generic Types vs Union Types.mp4
Generic Array (with "<" ">" angular brackets) and Predefined Array of string
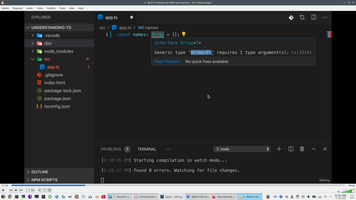
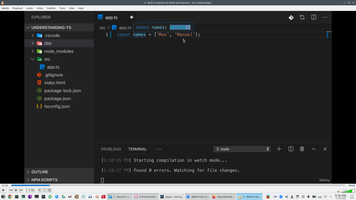
Define empty array with square brackets "[" "]", we can initialize in this way any kind of array.
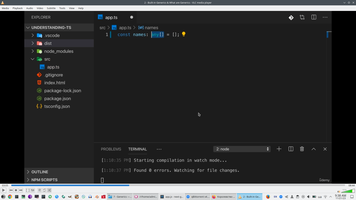
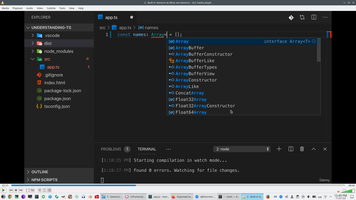
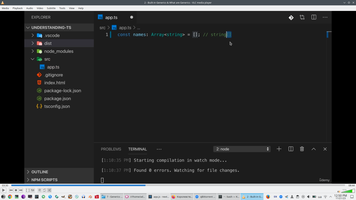
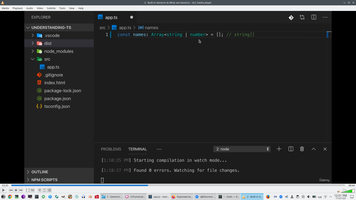
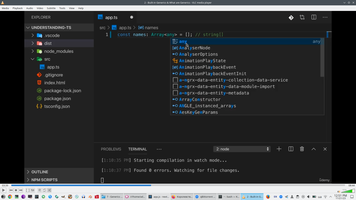
But correct definition allow us working with type safe.
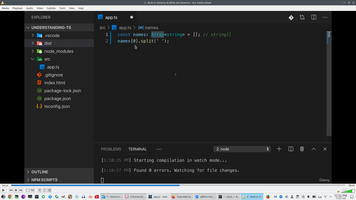
By default we have no information what data return JS Promise.
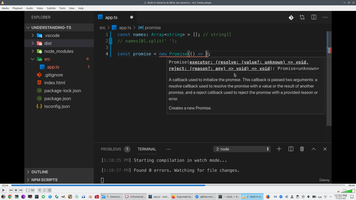
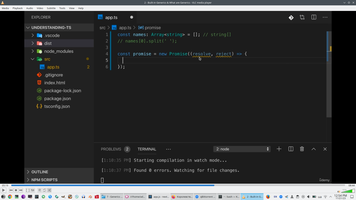
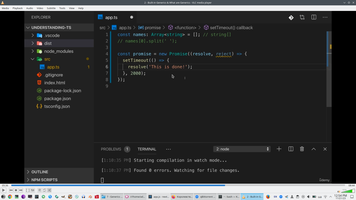
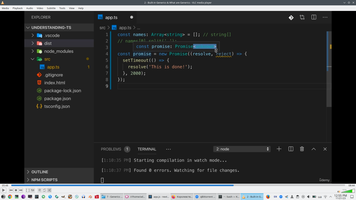
But we can define return datatype
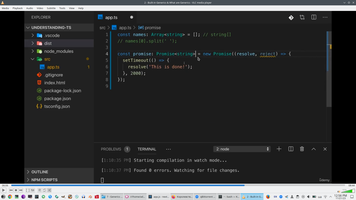
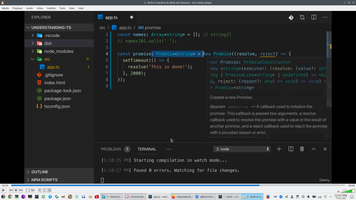
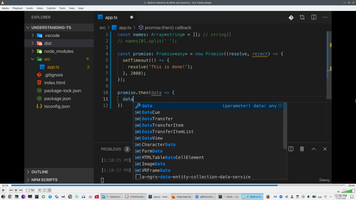
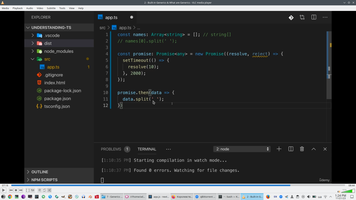
And can protect code from Split Number value (for example)
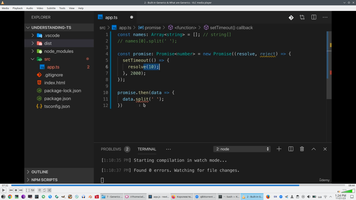
Object.Assign is JS method what allow copy all property from Source to Target object
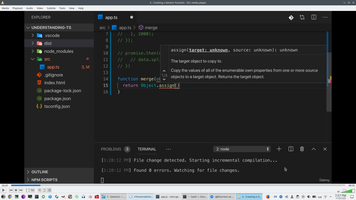
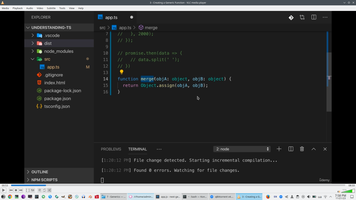
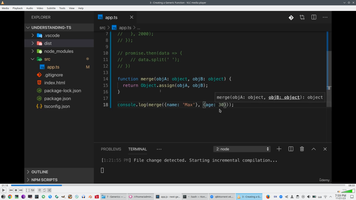
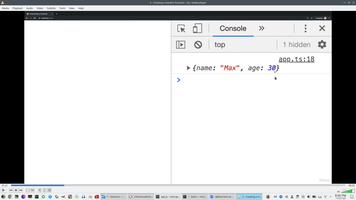
But TS not sure that property exists on target object.
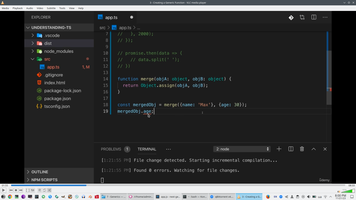
We can type cast of result object with "AS"
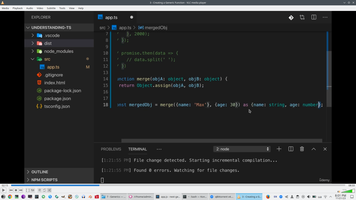
Or create Generic object
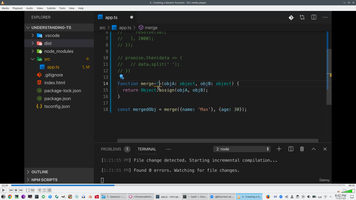
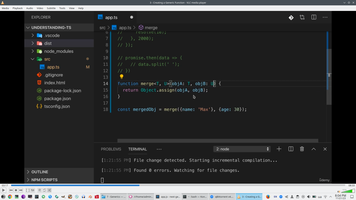
Result have intersection of type U "&" T, because for this function input parameters "T & U" explicitly setup output type as interception "T & U"
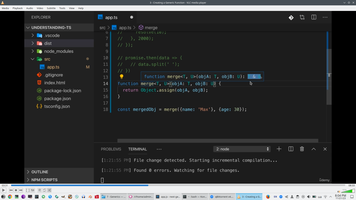
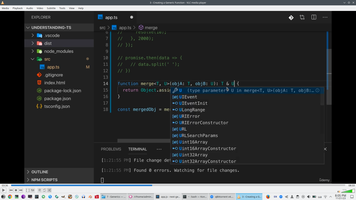
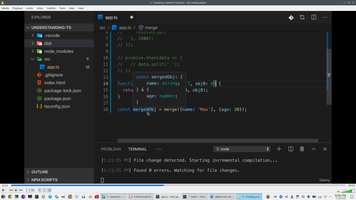
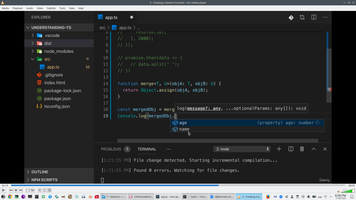
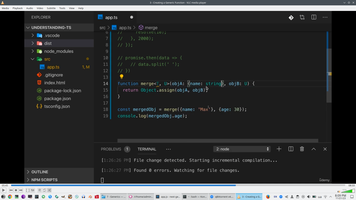
We call function with Array and TS correctly understand, we don't specify exact type, this is redundant.
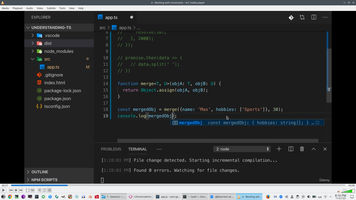
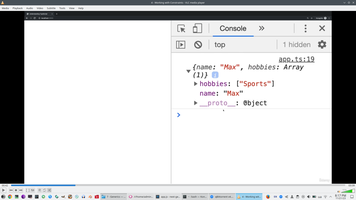
Constraint - limitation or restriction, we want to protect from call this function with simple value, because this function working only with Objects. Extends keyword help us.
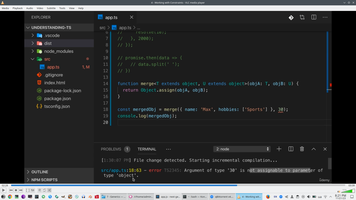
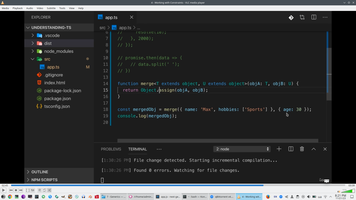
We can create any constraint
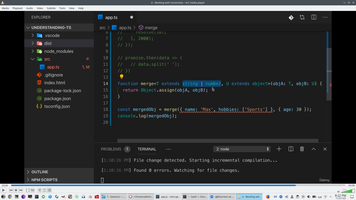
In this case function return tuple (value with description), Length property must be exist
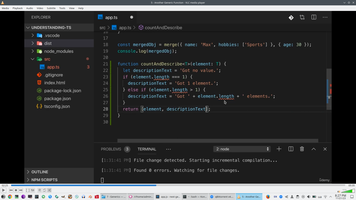
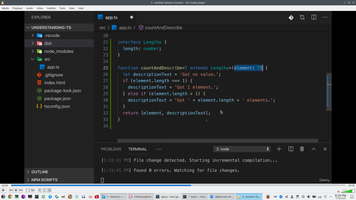
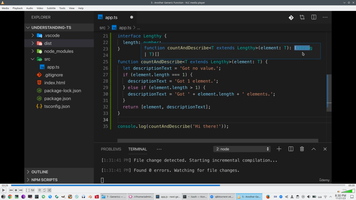
Also we can specify implicit output type
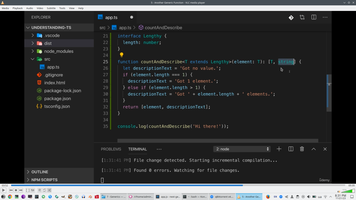
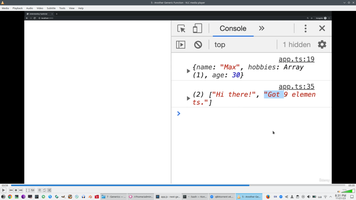
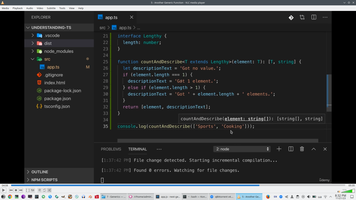
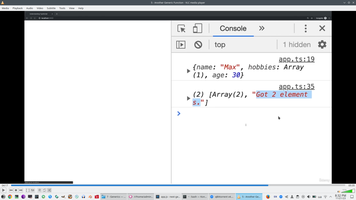
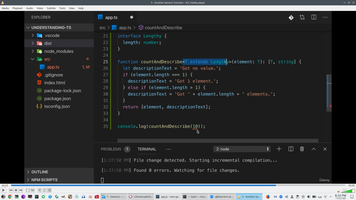
KeyOf Constraint - this is simple JS function, but this is not working on TS
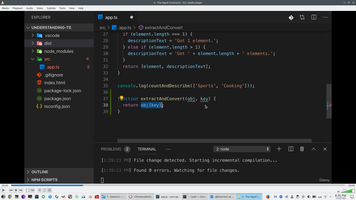
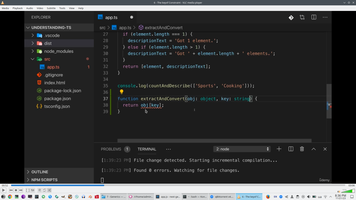
Because TS can not guarantee that key exist in object
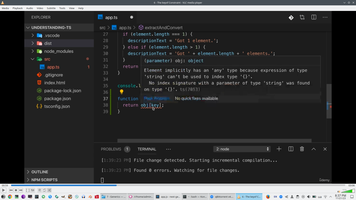
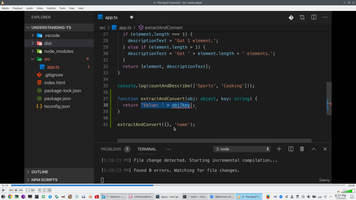
U extends KeyOf T - guarantee that U property exist in T object
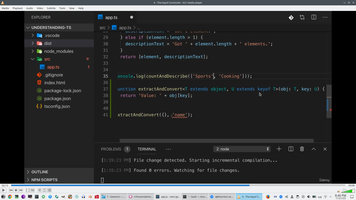
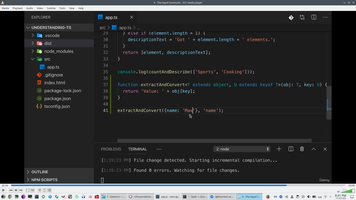
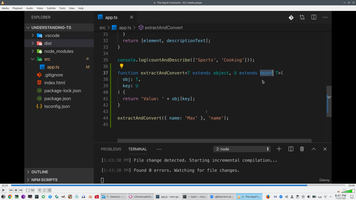
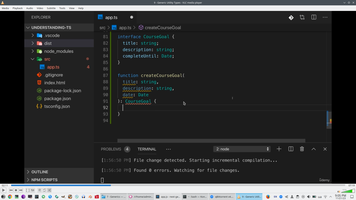
Generic utility types - Partial and Readonly - fix all object property of make it optional (locked ot open to modification)
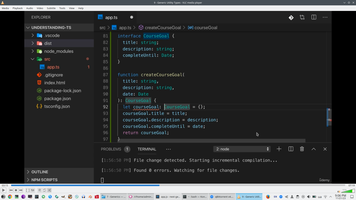
Partial mean that all property is optional, therefore we can create empty object firstly and than step-by-step adding property. And finally casting to clear type with "AS".
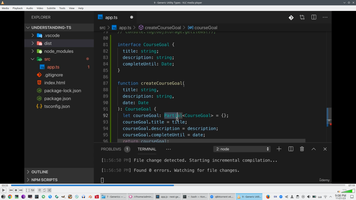
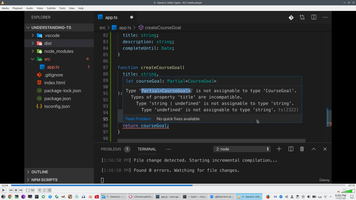
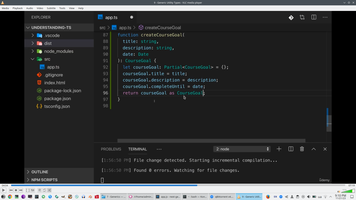
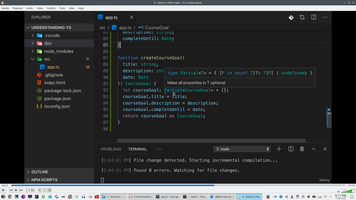
How to make array locked?
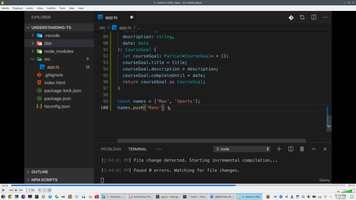
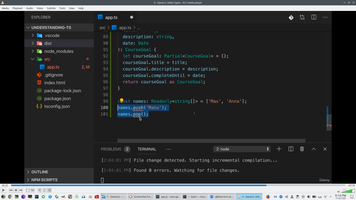
Readonly forbid changing any property
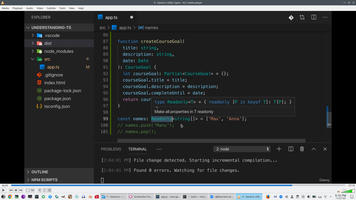
Union types vs Generic types. Union types allow story mixed value, buy Generic types allow stre only one object type. Generic type allow lock one certain type.
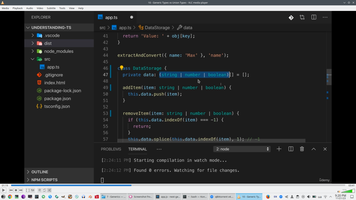
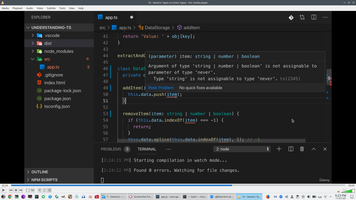
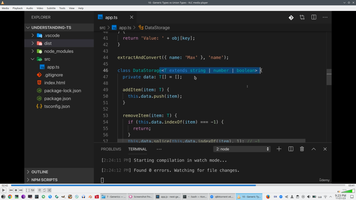
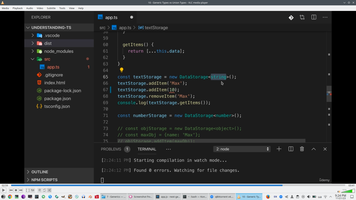
Related pages
- Angular documentation
- Typescript documentation
- Javascript documentation
- ECMAScript ES6 vs Typescript
- React vs Angular
- (2023) CloudflareWorker and Supabase
- (2022) NativeScript, AndroidBooks
- (2022) JS, Css
- (2022) Typescript, Webpack
- (2022) Angular, RxJs, Firebase, MongoDb
- (2022) Node, NestJs, Electron, Pwa, Telegram
- (2022) React, Redux, GraphQL, NextJs
- (2022) Angular/Typescript, JS books

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |