(back) Снова о делегатах (общие шаблоны VB/C#). (back)
1: Imports System
2:
3: Public Delegate Sub MyEventHandler() 'Delegate contains type safe reference to AddressOf
4: Module Program
5: Sub Main(args As String())
6:
7: Dim TestClassInstance2 As New TestClass
8: '3 way dynamically add event
9: AddHandler TestClassInstance2.Xevent, AddressOf MyHandler2 '1. AddressOff with implicit instance of delegate
10: AddHandler TestClassInstance2.Yevent, Sub() Console.WriteLine() '2. Action/Func
11: AddHandler TestClassInstance2.Zevent, New MyEventHandler(AddressOf MyHandler2) '3. Create explicit instance of delegate with strong delegate signature
12:
13: 'Delegate reference
14: Dim MyDelegate1 As MyEventHandler = AddressOf MyHandler2 '1
15: Dim MyDelegate2 As MyEventHandler = Sub() Console.WriteLine() '2
16: Dim MyDelegate3 As MyEventHandler = New MyEventHandler(AddressOf MyHandler2) '3
17:
18: 'Delegate as parameter
19: TestSub(AddressOf MyHandler2) '1
20: TestSub(Sub() Console.WriteLine()) '2
21: TestSub(MyDelegate2) '3
22:
23: 'Invoke
24: MyDelegate1.Invoke() 'Invoke From other Thread
25: MyDelegate1.DynamicInvoke() 'Invoke with lite-bound negotiate delegate type
26: Dim Res1 As AsyncCallback = MyDelegate1.BeginInvoke(AddressOf MyHandler2, New Object) 'Invoke asynchronously
27:
28: 'Generic Delegate with Action/Func
29: Dim MyAct As Action = AddressOf MyHandler2
30:
31: 'Action/Func
32: Task.Run(AddressOf MyHandler2) '1
33: Task.Run(Sub() Console.WriteLine()) '2
34: Task.Run(New Action(AddressOf MyHandler2)) '3
35:
36: 'Multicast delegate
37: Dim Multicast As MyEventHandler = AddressOf MyHandler2
38: Multicast = CType([Delegate].Combine(New MyEventHandler(AddressOf MyHandler1), New MyEventHandler(AddressOf MyHandler2)), MyEventHandler)
39: For Each One As MyEventHandler In Multicast.GetInvocationList
40: One.Invoke
41: Next
42: End Sub
43:
44: Sub MyHandler2()
45: End Sub
46:
47: Sub TestSub(X As MyEventHandler)
48: End Sub
49:
50: 'All about event
51: Dim WithEvents TestClassInstance1 As New TestClass 'Declare all events statically
52: Sub MyHandler1() Handles TestClassInstance1.Xevent, TestClassInstance1.Zevent 'This is static link eventHandler to class
53: End Sub
54: Friend Class TestClass
55: Public Event Xevent() 'Simple declaration
56: Public Event Yevent()
57: Public Event Zevent As MyEventHandler 'Declaration with strong type delegatees signature
58: Sub FlashEvent()
59: RaiseEvent Xevent() 'Simple raise event
60: RaiseEvent Zevent()
61: YeventEvent() 'Raise event with suffix <Event>
62: End Sub
63: End Class
64:
65: End Module
1: using System; using System.Threading.Tasks; using System.Runtime.CompilerServices;
2:
3: namespace Test1
4: {
5: public delegate void MyEventHandler(); // Delegate contains type safe reference to AddressOf
6: static class TestDelegate
7: {
8: static TestDelegate() => new TestClass();
9: public static void Main(string[] args)
10: {
11: TestClass TestClassInstance2 = new TestClass();
12: // 3 way dynamically add event
13: TestClassInstance2.Xevent += MyHandler2; // 1. AddressOff with implicit instance of delegate
14: TestClassInstance2.Yevent += () => Console.WriteLine(); // 2. Action/Func
15: TestClassInstance2.Zevent += new MyEventHandler(MyHandler2); // 3. Create explicit instance of delegate with strong delegate signature
16:
17: // Delegate reference
18: MyEventHandler MyDelegate1 = MyHandler2; // 1
19: MyEventHandler MyDelegate2 = () => Console.WriteLine(); // 2
20: MyEventHandler MyDelegate3 = new MyEventHandler(MyHandler2); // 3
21:
22: // Delegate as parameter
23: TestSub(MyHandler2); // 1
24: TestSub(() => Console.WriteLine()); // 2
25: TestSub(MyDelegate2); // 3
26:
27: // Invoke
28: MyDelegate1.Invoke(); // Invoke From other Thread
29: MyDelegate1.DynamicInvoke(); // Invoke with lite-bound negotiate delegate type
30: IAsyncResult Res1 = MyDelegate1.BeginInvoke(MyHandler3, new object()); // Invoke asynchronously
31:
32: // Generic Delegate with Action/Func
33: Action MyAct = MyHandler2;
34:
35: // Action/Func
36: Task.Run(MyHandler2); // 1
37: Task.Run(() => Console.WriteLine()); // 2
38: Task.Run(new Action(MyHandler2)); // 3
39:
40: // Multicast delegate
41: MyEventHandler Multicast = MyHandler2;
42: Multicast = (MyEventHandler)System.Delegate.Combine(new MyEventHandler(MyHandler1), new MyEventHandler(MyHandler2));
43: foreach (MyEventHandler One in Multicast.GetInvocationList())
44: One.Invoke();
45: }
46: private static void MyHandler3(IAsyncResult ar) { }
47: public static void MyHandler2() { }
48: public static void TestSub(MyEventHandler X) { }
49:
50: // All about event
51: private static TestClass _TestClassInstance1; // Declare all events statically
52: private static TestClass TestClassInstance1
53: {
54: [MethodImpl(MethodImplOptions.Synchronized)]
55: get
56: {
57: return _TestClassInstance1;
58: }
59:
60: [MethodImpl(MethodImplOptions.Synchronized)]
61: set
62: {
63: if (_TestClassInstance1 != null)
64: {
65: _TestClassInstance1.Xevent -= MyHandler1;
66: _TestClassInstance1.Zevent -= MyHandler1;
67: }
68:
69: _TestClassInstance1 = value;
70: if (_TestClassInstance1 != null)
71: {
72: _TestClassInstance1.Xevent += MyHandler1;
73: _TestClassInstance1.Zevent += MyHandler1;
74: }
75: }
76: }
77:
78: public static void MyHandler1() // This is static link eventHandler to class
79: {
80: }
81: internal class TestClass
82: {
83: public delegate void XeventEventHandler();
84: public delegate void YeventEventHandler();
85: public event XeventEventHandler Xevent; // Simple declaration
86: public event YeventEventHandler Yevent;
87: public event MyEventHandler Zevent; // Declaration with strong type delegatees signature
88: public void FlashEvent()
89: {
90: Xevent?.Invoke(); // Simple raise event
91: Zevent?.Invoke();
92: Yevent(); // Raise event with suffix <Event>
93: }
94: }
95: }
96: }
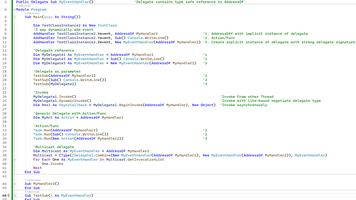
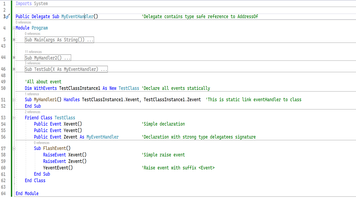
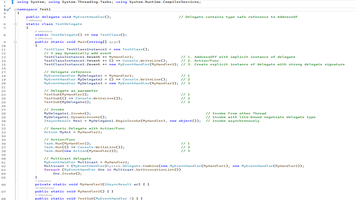
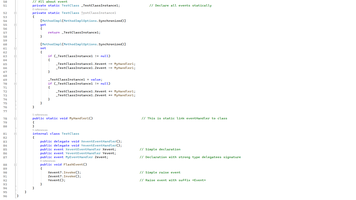
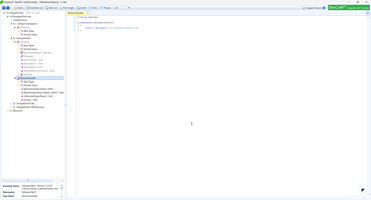
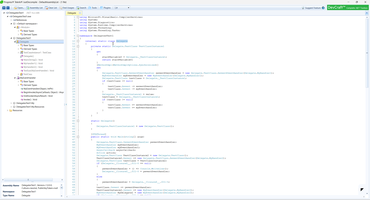
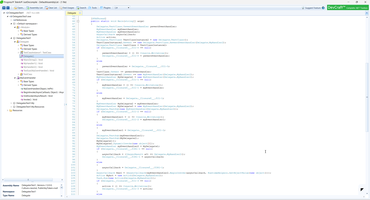
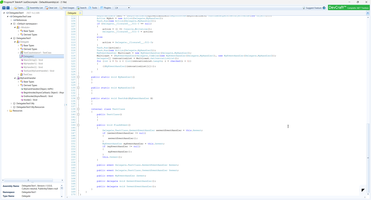
- More about events and delegates
- Events/Delegates in VB.NET (Static/Dynamic declaration, AddressOf/RaiseEvent/AddHandler/WithEvents/Handles statement,SingleCast/MultiCast/InvocationList, Invoke/BeginInvoke/DynamicInvoke/AsyncInvoke, Automatically instantiate.
- MS: Delegates and Events (VB.NET)
Comments (
)

<00>
<01>
<02>
<03>
<04>
<05>
<06>
<07>
<08>
<09>
<10>
<11>
<12>
<13>
<14>
<15>
<16>
<17>
<18>
<19>
<20>
<21>
<22>
<23>
Link to this page:
http://www.vb-net.com/QuickHelp/Delegates/Index.htm
<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |